BMP280 Based Weather Station using Arduino and OLED Display
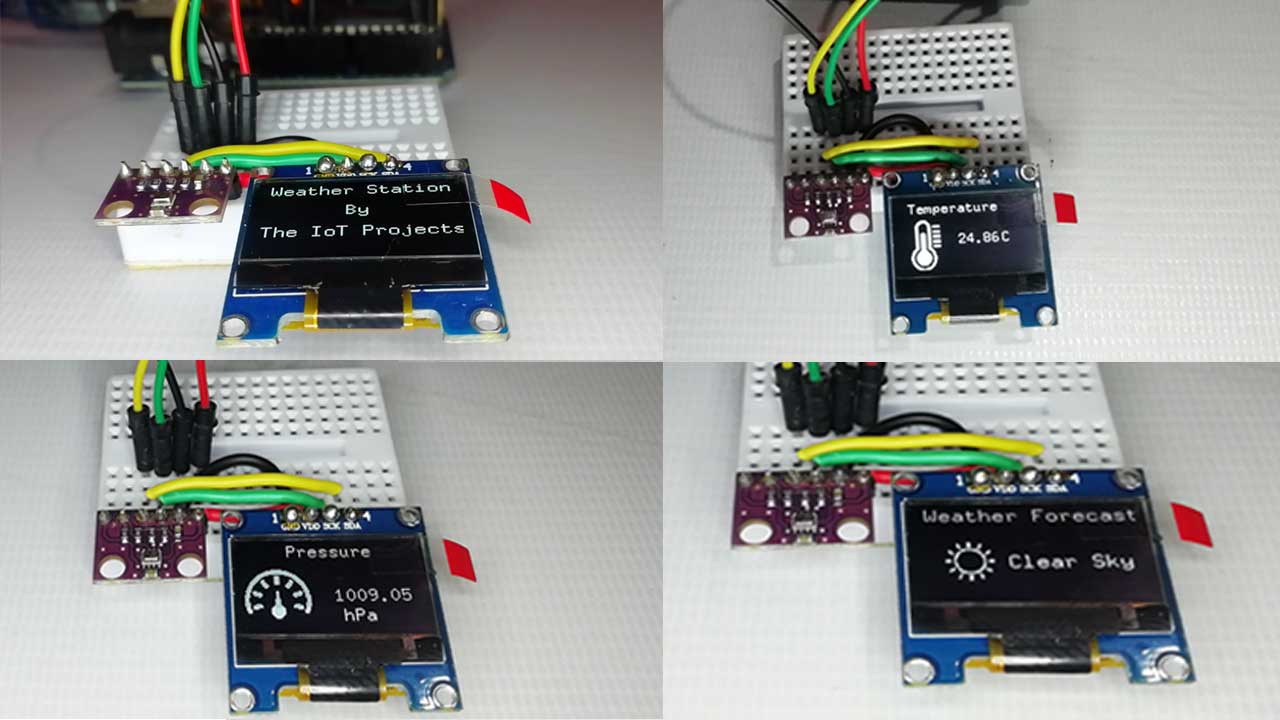
The weather station is an interesting project for beginners and very useful for specialist manufacturers. It is simple to build and in general provides sufficiently accurate and useful weather data. For today’s tutorial, we will build another BMP280 Based Weather Station using Arduino and OLED Display. Using the BMP280 sensor we will be able to monitor the temperature, atmospheric pressure, and altitude.
The Arduino reads the temperature, pressure, and altitude values from the BMP280 sensor and prints them on a 0.96″ (SSD1306) OLED display (128×64 pixel).
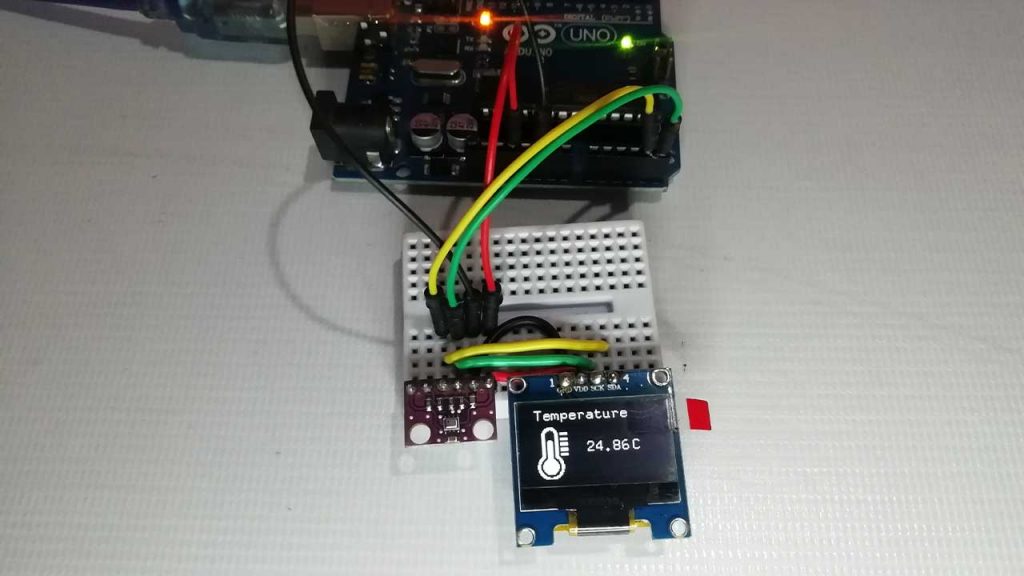
The BMP280 is Bosch’s next-generation sensor and is an upgrade of their previous sensors, including the BMP085, BMP180, and BMP183. The BMP280 is excellent for weather sensing with low noise of 0.25 meters. The conversion rate is as fast as the previous sensors. Additionally, the BMP280 can be used in both I2C and SPI modes. It also has an onboard voltage regulator and a level shifter, which means it can be used with either a 3volt or 5volt supply.
Along with the BMP280, we will use the I2C-based, 0.96″ (128×62 Pixel) OLED Display to show the values obtained from the BMP280 sensor.
At the end of this tutorial, you will also learn how to interface BMP280 or any other I2C device with Arduino and display data in the OLED display.
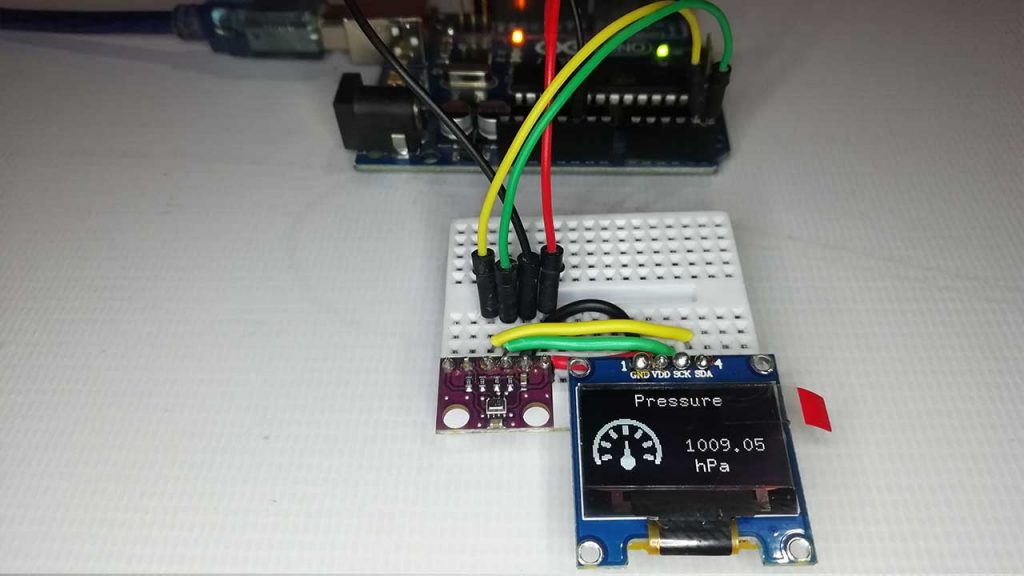
Required Components
The following components are required to build BMP280 based Weather Station project.
S.N | Components Name | Description | Quantity | ![]() |
---|---|---|---|---|
1 | Arduino UNO | ARDUINO UNO R3 | 1 | https://amzn.to/2L0iZf2 |
2 | BMP280 Sensor | Barometric Pressure & Altitude Sensor | 1 | https://amzn.to/2OmfYav |
0.96 Inch OLED Display | 0.96 Inch OLED Module 128x64 SSD1306 Driver I2C Serial Self-Luminous Display Board | 1 | https://amzn.to/3pGssa7 | |
3 | Jumper Wires | Male to Male Jumper Wires | 8 | https://amzn.to/2JWSR44 |
4 | USB 2.0 cable | USB 2.0 cable type A/B | 1 | https://amzn.to/3688OwF |
BMP280 Sensor Pinout
The following table is the BMP280 sensor Pinout table:

VCC | Powers the sensor |
GND | Ground Pin |
SCL | SCL pin for I2C communicationSCK pin for SPI communication |
SDA | SDA pin for I2C communicationSDI (MOSI) pin for SPI communication |
SDO | SDO (MISO) pin for SPI communication |
CSB | Chip select pin for SPI communication |
BMP280 Sensor Measures
As I already mentioned above, The BMP280 is a Barometric pressure and digital Temperature sensor that can measures:
- Temperature
- Barometric pressure
- Altitude
Accuracy and Operation Range of BMP280 Sensor
The following table shows the Accuracy and operation rangeof the temperature, pressure, and altitude of the BMP280 Sensor:
Sensor | Accuracy | Operation Range |
Temperature | +/- 1.0ºC | -40 to 85 ºC |
Pressure | +/- 1 hPa | 300 to 1100 hPa |
Altitude | +/- 1 M | 0 – 30,000ft |
Our Previous Weather Station projects
- IoT Weather Station using DHT11 Sensor
- Interface BMP280 Sensor With Arduino
- BME280 Based Mini Weather Station using ESP8266/ESP32
- Simple Weather station using Arduino & BME280 Barometric Pressure Sensor
- ESP8266 & BME280 IoT Weather Station
Interface BMP280 Sensor & OLED Display with Arduino
Now, Let’s wire the BMP280 sensor and 0.96″ OLED Display with the Arduino. The Connections are fairly simple because the OLED and the BMP280 both communicate with the Arduino via I2C mode. This means we can connect both modules to the same (I2C) pins on the Arduino. Connect the components as shown in the schematics below.
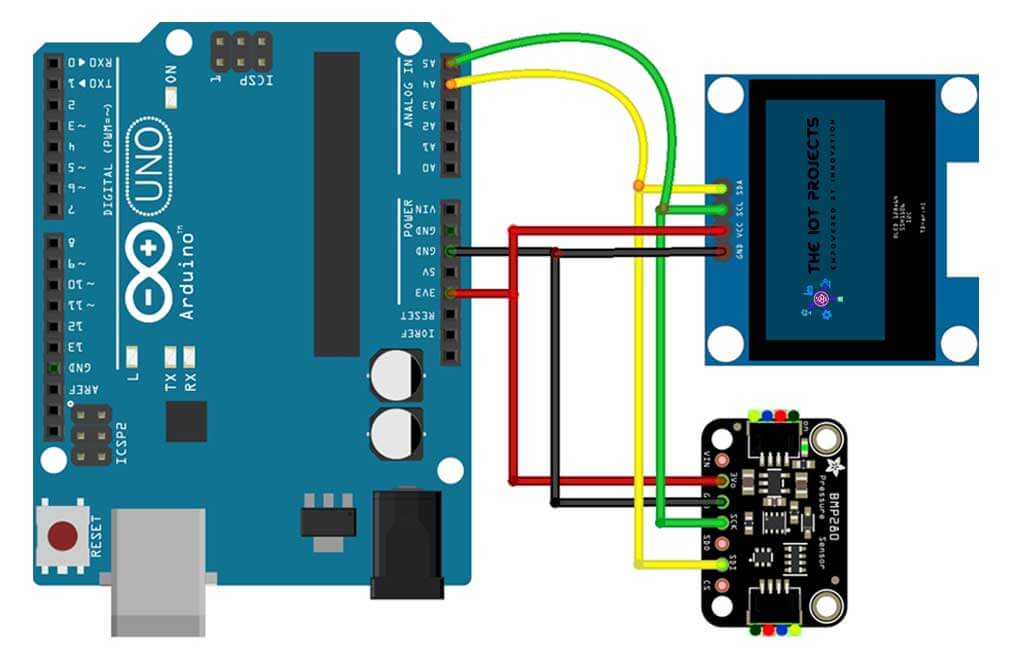
BMP280 | ARDUINO UNO |
Vin | 3.3V |
GND | GND |
SCL | A5 |
SDA | A4 |
OLED Display | ARDUINO UNO |
GND | GND |
VCC | 3.3V |
SCK | A5 |
SDA | A4 |
Go through the schematics once more to ensure everything is properly connected.
Programming Arduino for BMP280 based Weather Station
To write the program code for this weather station project, we will use four key libraries; the Adafruit BMP280 library, the Adafruit Unified Sensor Library, the Adafruit SSD1306 library, and U8Glib library. These four libraries can be downloaded from the Arduino Library Manager or via the download link attached below.
The Adafruit BMP280 contains functions that make it easy to write the code. It will obtain the parameters from the sensor while the U8Glib and SSD1306 libraries are used to easily display texts and graphics on the OLED display.
#include <Wire.h>
#include <SPI.h>
#include <Adafruit_BMP280.h>
#include <Adafruit_Sensor.h>
//for Pin defined for SPI Connections
#define BMP_SCK 13
#define BMP_MISO 12
#define BMP_MOSI 11
#define BMP_CS 10
#include "U8glib.h"
Creating storm bitmap graphics for OLED display
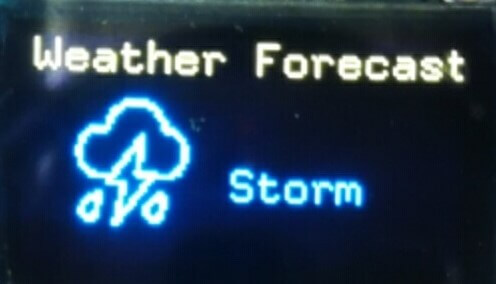
const uint8_t storm[] PROGMEM= {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF,
0x80, 0x00, 0x00, 0x00, 0x07, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x60, 0x00, 0x00, 0x00,
0x0C, 0x00, 0x60, 0x00, 0x00, 0x00, 0x18, 0x00, 0x30, 0x00, 0x00, 0x00, 0x18, 0x00, 0x18, 0x00,
0x00, 0x00, 0x10, 0x00, 0x18, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x1E, 0x00, 0x00, 0x07, 0xE0, 0x00,
0x0F, 0x00, 0x00, 0x0C, 0x00, 0x04, 0x01, 0x80, 0x00, 0x18, 0x00, 0x0C, 0x00, 0xC0, 0x00, 0x18,
0x00, 0x1C, 0x00, 0x40, 0x00, 0x10, 0x00, 0x1C, 0x00, 0x60, 0x00, 0x30, 0x00, 0x3C, 0x00, 0x60,
0x00, 0x30, 0x00, 0x6C, 0x00, 0x60, 0x00, 0x30, 0x00, 0xCC, 0x00, 0x60, 0x00, 0x10, 0x00, 0xCC,
0x00, 0x60, 0x00, 0x10, 0x01, 0x88, 0x00, 0x40, 0x00, 0x18, 0x03, 0x08, 0x00, 0xC0, 0x00, 0x0C,
0x06, 0x08, 0x01, 0x80, 0x00, 0x07, 0x06, 0x1F, 0xC3, 0x80, 0x00, 0x03, 0xFC, 0x0F, 0xFE, 0x00,
0x00, 0x00, 0x18, 0x01, 0x80, 0x00, 0x00, 0x00, 0x1F, 0x83, 0x00, 0x00, 0x00, 0x00, 0x01, 0x86,
0x00, 0x00, 0x00, 0x00, 0x01, 0x86, 0x00, 0x00, 0x00, 0x00, 0xC1, 0x8C, 0x30, 0x00, 0x00, 0x03,
0xC1, 0x18, 0x78, 0x00, 0x00, 0x07, 0xC1, 0x30, 0xF8, 0x00, 0x00, 0x0C, 0xC3, 0x31, 0x98, 0x00,
0x00, 0x08, 0x83, 0x63, 0x18, 0x00, 0x00, 0x18, 0x83, 0xC3, 0x10, 0x00, 0x00, 0x19, 0x83, 0x83,
0x30, 0x00, 0x00, 0x0F, 0x83, 0x01, 0xF0, 0x00, 0x00, 0x07, 0x07, 0x00, 0xE0, 0x00, 0x00, 0x00,
0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
Create pressure gauge bitmap graphics
const uint8_t presure[] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0F, 0xF0, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xC0, 0x00,
0x00, 0x0F, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x3F, 0xC0, 0x03, 0xFC, 0x00, 0x00, 0x7E, 0x03, 0xC0,
0x7E, 0x00, 0x00, 0xF8, 0x03, 0xC0, 0x1F, 0x00, 0x01, 0xF0, 0x03, 0xC0, 0x0F, 0x80, 0x03, 0xE6,
0x03, 0xC0, 0x67, 0xC0, 0x07, 0xCE, 0x03, 0xC0, 0x73, 0xE0, 0x0F, 0x8F, 0x03, 0xC0, 0xF1, 0xF0,
0x0F, 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0x1E, 0x07, 0xC0, 0x03, 0xE0, 0x78, 0x1C, 0x03, 0xC0, 0x03,
0xC0, 0x38, 0x3C, 0x01, 0x80, 0x01, 0x80, 0x3C, 0x38, 0x00, 0x00, 0x00, 0x00, 0x1C, 0x78, 0x00,
0x00, 0x00, 0x00, 0x1E, 0x7B, 0x80, 0x01, 0x80, 0x01, 0xDE, 0x73, 0xF0, 0x01, 0x80, 0x0F, 0xCE,
0x73, 0xF8, 0x01, 0x80, 0x1F, 0xCE, 0xF1, 0xF8, 0x01, 0x80, 0x1F, 0x8F, 0xF0, 0x30, 0x01, 0x80,
0x0C, 0x0F, 0xE0, 0x00, 0x01, 0x80, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0xE0, 0x00,
0x03, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F,
0xF0, 0xF0, 0x03, 0xC0, 0x0F, 0x0F, 0xF3, 0xF8, 0x07, 0xE0, 0x1F, 0xCF, 0x73, 0xF8, 0x0F, 0xF0,
0x1F, 0xCE, 0x73, 0xC0, 0x1F, 0xF8, 0x03, 0xCE, 0x78, 0x00, 0x1F, 0xF8, 0x00, 0x1E, 0x38, 0x00,
0x1F, 0xF8, 0x00, 0x1C, 0x38, 0x00, 0x1F, 0xF8, 0x00, 0x1C, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00,
0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xC0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
Display Rain bitmap graphics on OLED display.
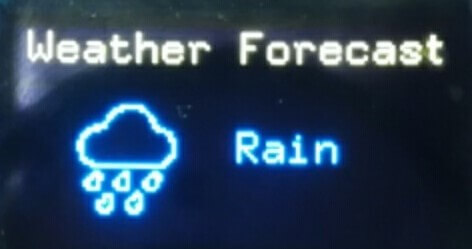
const uint8_t rain[] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x1C, 0x18, 0x00, 0x00,
0x00, 0x00, 0x30, 0x04, 0x00, 0x00, 0x00, 0x00, 0x60, 0x02, 0x00, 0x00, 0x00, 0x00, 0x40, 0x03,
0x00, 0x00, 0x00, 0x00, 0x80, 0x01, 0x00, 0x00, 0x00, 0x07, 0x80, 0x01, 0x80, 0x00, 0x00, 0x1E,
0x00, 0x00, 0xE0, 0x00, 0x00, 0x30, 0x00, 0x00, 0x18, 0x00, 0x00, 0x60, 0x00, 0x00, 0x08, 0x00,
0x00, 0x40, 0x00, 0x00, 0x0C, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x04, 0x00, 0x00, 0xC0, 0x00, 0x00,
0x04, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x04, 0x00, 0x00, 0x40, 0x00, 0x00, 0x04, 0x00, 0x00, 0x40,
0x00, 0x00, 0x0C, 0x00, 0x00, 0x60, 0x00, 0x00, 0x18, 0x00, 0x00, 0x38, 0x00, 0x00, 0x30, 0x00,
0x00, 0x0F, 0xFF, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x03, 0x01,
0x80, 0x00, 0x00, 0x0F, 0x07, 0x03, 0xC0, 0x00, 0x00, 0x1B, 0x09, 0x04, 0xC0, 0x00, 0x00, 0x32,
0x19, 0x0C, 0x80, 0x00, 0x00, 0x32, 0x19, 0x0C, 0x80, 0x00, 0x00, 0x1E, 0x0F, 0x07, 0x00, 0x00,
0x00, 0x00, 0x00, 0x20, 0x00, 0x00, 0x00, 0x00, 0xE0, 0x70, 0x00, 0x00, 0x00, 0x00, 0xA0, 0xD0,
0x00, 0x00, 0x00, 0x03, 0xA1, 0x10, 0x00, 0x00, 0x00, 0x02, 0x21, 0x30, 0x00, 0x00, 0x00, 0x03,
0x61, 0xB0, 0x00, 0x00, 0x00, 0x01, 0xC0, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00};
Creating sunny day graphics for OLED display
const uint8_t sunny[] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x83, 0x04, 0x00, 0x00, 0x00, 0x00,
0x83, 0x04, 0x00, 0x00, 0x00, 0x00, 0xC3, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x40, 0x08, 0x00, 0x00,
0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x00, 0x10, 0x30, 0x38, 0x20, 0x00, 0x00, 0x18, 0xC0, 0x0C,
0x60, 0x00, 0x00, 0x0C, 0x80, 0x04, 0xC0, 0x00, 0x00, 0x01, 0x80, 0x02, 0x00, 0x00, 0x00, 0x01,
0x00, 0x03, 0x00, 0x00, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x00, 0x02, 0x00, 0x01, 0x00, 0x00,
0x00, 0x72, 0x00, 0x01, 0x38, 0x00, 0x00, 0x72, 0x00, 0x01, 0x38, 0x00, 0x00, 0x02, 0x00, 0x01,
0x00, 0x00, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0x00, 0x03, 0x00, 0x00, 0x00, 0x01,
0x00, 0x02, 0x00, 0x00, 0x00, 0x04, 0x80, 0x06, 0x80, 0x00, 0x00, 0x1C, 0xC0, 0x0C, 0xE0, 0x00,
0x00, 0x10, 0x70, 0x38, 0x20, 0x00, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x40, 0x08,
0x00, 0x00, 0x00, 0x00, 0x43, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x83, 0x04, 0x00, 0x00, 0x00, 0x00,
0x83, 0x04, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
Generates the cloudy day bitmap graphics for cloudy weather
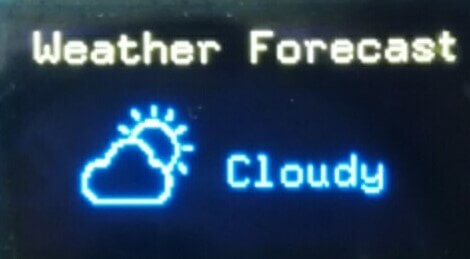
const uint8_t cloudy[] PROGMEM= {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0x02, 0x08, 0x40, 0x00,
0x00, 0x00, 0x03, 0x08, 0x40, 0x00, 0x00, 0x00, 0x01, 0x00, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x3C,
0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x31, 0x81, 0x8C, 0x00, 0x00, 0x00,
0x1B, 0x00, 0xD8, 0x00, 0x00, 0x00, 0x02, 0x00, 0x60, 0x00, 0x00, 0x00, 0x06, 0x00, 0x20, 0x00,
0x00, 0x00, 0x3F, 0x80, 0x20, 0x00, 0x00, 0x00, 0xE0, 0xC0, 0x10, 0x00, 0x00, 0x00, 0x80, 0x60,
0x17, 0x00, 0x00, 0x01, 0x80, 0x30, 0x10, 0x00, 0x00, 0x03, 0x00, 0x10, 0x10, 0x00, 0x00, 0x02,
0x00, 0x10, 0x20, 0x00, 0x00, 0x1E, 0x00, 0x1C, 0x20, 0x00, 0x00, 0x7C, 0x00, 0x0E, 0x48, 0x00,
0x00, 0xC0, 0x00, 0x01, 0xCC, 0x00, 0x00, 0x80, 0x00, 0x01, 0x84, 0x00, 0x01, 0x80, 0x00, 0x00,
0x80, 0x00, 0x01, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x00,
0x00, 0x00, 0xC0, 0x00, 0x01, 0x00, 0x00, 0x00, 0x80, 0x00, 0x01, 0x80, 0x00, 0x00, 0x80, 0x00,
0x00, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x00, 0x3F, 0xFF, 0xFE,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
Displays the temperature icon on display.
const uint8_t temperature[] PROGMEM = {
0x00, 0x00, 0x1F, 0x80, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x00, 0x00, 0x00, 0xF9, 0xE0,
0x00, 0x00, 0x00, 0x01, 0xE0, 0x70, 0x00, 0x00, 0x00, 0x01, 0xC0, 0x70, 0x00, 0x00, 0x00, 0x03,
0x80, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x80, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x80, 0x38, 0x00, 0x00,
0x00, 0x03, 0x80, 0x38, 0x00, 0x00, 0x00, 0x03, 0x80, 0x38, 0x00, 0x00, 0x00, 0x03, 0x80, 0x39,
0xFC, 0x00, 0x00, 0x03, 0x80, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x8E, 0x38, 0x00, 0x00, 0x00, 0x03,
0x9F, 0x38, 0x00, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00,
0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x38, 0x00, 0x00, 0x00, 0x03, 0x9F, 0x38,
0x00, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03,
0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x38, 0x00, 0x00, 0x00, 0x03, 0x9F, 0x38, 0x00, 0x00,
0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x38,
0x00, 0x00, 0x00, 0x07, 0x9F, 0x3C, 0x00, 0x00, 0x00, 0x0F, 0x1F, 0x1E, 0x00, 0x00, 0x00, 0x1E,
0x3F, 0x87, 0x00, 0x00, 0x00, 0x1C, 0x7F, 0xC7, 0x00, 0x00, 0x00, 0x38, 0xFF, 0xE3, 0x80, 0x00,
0x00, 0x39, 0xFF, 0xF3, 0x80, 0x00, 0x00, 0x31, 0xFF, 0xF1, 0x80, 0x00, 0x00, 0x73, 0xFF, 0xF9,
0xC0, 0x00, 0x00, 0x73, 0xFF, 0xF9, 0xC0, 0x00, 0x00, 0x73, 0xFF, 0xF9, 0xC0, 0x00, 0x00, 0x73,
0xFF, 0xF9, 0xC0, 0x00, 0x00, 0x31, 0xFF, 0xF1, 0x80, 0x00, 0x00, 0x39, 0xFF, 0xF1, 0x80, 0x00,
0x00, 0x38, 0xFF, 0xE3, 0x80, 0x00, 0x00, 0x1C, 0x7F, 0xC7, 0x00, 0x00, 0x00, 0x1E, 0x3F, 0x87,
0x00, 0x00, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x07, 0x80, 0x3C, 0x00, 0x00, 0x00, 0x03,
0xF1, 0xF8, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00
};
Creating Splash Screen for BMP280 based Weather Station on OLED display using Arduino
void draw(void) {
// graphic commands to redraw the complete screen should be placed here
u8g.setFont(u8g_font_8x13);
u8g.setPrintPos(5,10);
u8g.print("Weather Station");
u8g.setPrintPos(55,30);
u8g.print("By");
u8g.setPrintPos(2,50);
u8g.print("The IoT Projects");
}
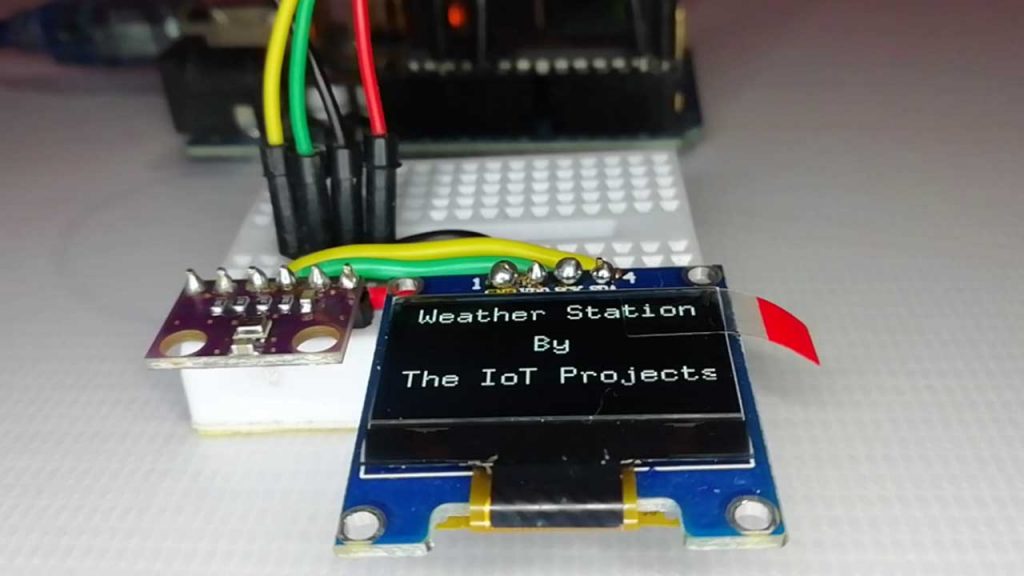
It reads the current pressure from BMP280 sensor and display it on the OLED Display.
void draw_4(void){
pressure=bmp.readPressure()/100;
delay(1);
u8g.setPrintPos(32,10);
u8g.print("Pressure");
u8g.setPrintPos(65,45);
u8g.print(pressure);
u8g.setPrintPos(70,60);
u8g.print("hPa");
u8g.drawBitmapP( 0, 15, 6, 48,presure);
}
This code display the current temperature on display by grabbing them from BMP280 module.
void draw_2(void){
u8g.setFont(u8g_font_8x13);
u8g.setPrintPos(0,10);
u8g.print(" Temperature");
u8g.setPrintPos(55,40);
u8g.print(temp);
u8g.setPrintPos(97,40);
u8g.print("C");
u8g.drawBitmapP( 0, 17, 6,48,temperature);
}
Displays the weather forecast using barometric pressure value.
void draw3(void){
u8g.setPrintPos(3,10);
u8g.print("Weather Forecast");
if ((pressure<=1000.59)&&(pressure>998.5)){
u8g.drawBitmapP( 10, 15, 6, 48,cloudy);
u8g.setPrintPos(60,45);
u8g.print("Cloudy");
}
else if((pressure<998.5)&&(pressure>996.5))
{
u8g.drawBitmapP( 10, 15, 6, 48,rain);
u8g.setPrintPos(66,38);
u8g.print("Rain");}
else if((pressure>1000.6)&&(pressure<1050)) { u8g.drawBitmapP( 5, 15, 6, 48,sunny); u8g.setPrintPos(50,45); u8g.print("Clear Sky");} else if((pressure>990)&&(pressure<=996.4))
{
u8g.drawBitmapP( 5, 15, 6, 48,storm);
u8g.setPrintPos(60,45);
u8g.print("Storm");}
else{
u8g.setPrintPos(40,40);
u8g.print("Error!");
}
}
In the setup part, we have started a serial monitor for debugging errors. then, we test the BMP280 sensor working or not. Finally, display all the weather station data by auto changing screen with sliding animation every 2 seconds.
void setup() {
Serial.begin(9600);
Serial.println(F("BMP280 test"));
if (!bmp.begin()) {
Serial.println(F("Could not find a valid BMP280 sensor, check wiring!"));
while (1);
}
u8g.firstPage(); // first page
do {
draw();
}
while( u8g.nextPage() );
delay(2000);
}
void loop() {
temp=bmp.readTemperature();
u8g.firstPage(); // Next page
do {
draw_2();
}
while( u8g.nextPage() );
delay(2000);
u8g.firstPage(); // Next page
do {
draw_4();
}
while( u8g.nextPage() );
delay(2000);
u8g.firstPage(); // Next page
do {
draw3();
}
while( u8g.nextPage() );
delay(2000);
}
Final Program Code For BMP280 based Weather Station
The complete code for the BMP280 Based Weather Station using Arduino and OLED Display project is provided below. You can simply copy the code to your Arduino IDE and upload it to your correct board.
#include <Wire.h>
#include <SPI.h>
#include <Adafruit_BMP280.h>
#include <Adafruit_Sensor.h>
//for Pin defined for SPI Connections
#define BMP_SCK 13
#define BMP_MISO 12
#define BMP_MOSI 11
#define BMP_CS 10
#include "U8glib.h"
Adafruit_BMP280 bmp;
U8GLIB_SSD1306_128X64 u8g(U8G_I2C_OPT_NONE|U8G_I2C_OPT_DEV_0); // I2C / TWI
float pressure;
float temp;
const uint8_t storm[] PROGMEM= {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF,
0x80, 0x00, 0x00, 0x00, 0x07, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x60, 0x00, 0x00, 0x00,
0x0C, 0x00, 0x60, 0x00, 0x00, 0x00, 0x18, 0x00, 0x30, 0x00, 0x00, 0x00, 0x18, 0x00, 0x18, 0x00,
0x00, 0x00, 0x10, 0x00, 0x18, 0x00, 0x00, 0x03, 0xF0, 0x00, 0x1E, 0x00, 0x00, 0x07, 0xE0, 0x00,
0x0F, 0x00, 0x00, 0x0C, 0x00, 0x04, 0x01, 0x80, 0x00, 0x18, 0x00, 0x0C, 0x00, 0xC0, 0x00, 0x18,
0x00, 0x1C, 0x00, 0x40, 0x00, 0x10, 0x00, 0x1C, 0x00, 0x60, 0x00, 0x30, 0x00, 0x3C, 0x00, 0x60,
0x00, 0x30, 0x00, 0x6C, 0x00, 0x60, 0x00, 0x30, 0x00, 0xCC, 0x00, 0x60, 0x00, 0x10, 0x00, 0xCC,
0x00, 0x60, 0x00, 0x10, 0x01, 0x88, 0x00, 0x40, 0x00, 0x18, 0x03, 0x08, 0x00, 0xC0, 0x00, 0x0C,
0x06, 0x08, 0x01, 0x80, 0x00, 0x07, 0x06, 0x1F, 0xC3, 0x80, 0x00, 0x03, 0xFC, 0x0F, 0xFE, 0x00,
0x00, 0x00, 0x18, 0x01, 0x80, 0x00, 0x00, 0x00, 0x1F, 0x83, 0x00, 0x00, 0x00, 0x00, 0x01, 0x86,
0x00, 0x00, 0x00, 0x00, 0x01, 0x86, 0x00, 0x00, 0x00, 0x00, 0xC1, 0x8C, 0x30, 0x00, 0x00, 0x03,
0xC1, 0x18, 0x78, 0x00, 0x00, 0x07, 0xC1, 0x30, 0xF8, 0x00, 0x00, 0x0C, 0xC3, 0x31, 0x98, 0x00,
0x00, 0x08, 0x83, 0x63, 0x18, 0x00, 0x00, 0x18, 0x83, 0xC3, 0x10, 0x00, 0x00, 0x19, 0x83, 0x83,
0x30, 0x00, 0x00, 0x0F, 0x83, 0x01, 0xF0, 0x00, 0x00, 0x07, 0x07, 0x00, 0xE0, 0x00, 0x00, 0x00,
0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const uint8_t presure[] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x0F, 0xF0, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xFF, 0xC0, 0x00,
0x00, 0x0F, 0xFF, 0xFF, 0xF0, 0x00, 0x00, 0x3F, 0xC0, 0x03, 0xFC, 0x00, 0x00, 0x7E, 0x03, 0xC0,
0x7E, 0x00, 0x00, 0xF8, 0x03, 0xC0, 0x1F, 0x00, 0x01, 0xF0, 0x03, 0xC0, 0x0F, 0x80, 0x03, 0xE6,
0x03, 0xC0, 0x67, 0xC0, 0x07, 0xCE, 0x03, 0xC0, 0x73, 0xE0, 0x0F, 0x8F, 0x03, 0xC0, 0xF1, 0xF0,
0x0F, 0x07, 0x83, 0xC1, 0xE0, 0xF0, 0x1E, 0x07, 0xC0, 0x03, 0xE0, 0x78, 0x1C, 0x03, 0xC0, 0x03,
0xC0, 0x38, 0x3C, 0x01, 0x80, 0x01, 0x80, 0x3C, 0x38, 0x00, 0x00, 0x00, 0x00, 0x1C, 0x78, 0x00,
0x00, 0x00, 0x00, 0x1E, 0x7B, 0x80, 0x01, 0x80, 0x01, 0xDE, 0x73, 0xF0, 0x01, 0x80, 0x0F, 0xCE,
0x73, 0xF8, 0x01, 0x80, 0x1F, 0xCE, 0xF1, 0xF8, 0x01, 0x80, 0x1F, 0x8F, 0xF0, 0x30, 0x01, 0x80,
0x0C, 0x0F, 0xE0, 0x00, 0x01, 0x80, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0xE0, 0x00,
0x03, 0xC0, 0x00, 0x07, 0xE0, 0x00, 0x03, 0xC0, 0x00, 0x07, 0xF0, 0x00, 0x03, 0xC0, 0x00, 0x0F,
0xF0, 0xF0, 0x03, 0xC0, 0x0F, 0x0F, 0xF3, 0xF8, 0x07, 0xE0, 0x1F, 0xCF, 0x73, 0xF8, 0x0F, 0xF0,
0x1F, 0xCE, 0x73, 0xC0, 0x1F, 0xF8, 0x03, 0xCE, 0x78, 0x00, 0x1F, 0xF8, 0x00, 0x1E, 0x38, 0x00,
0x1F, 0xF8, 0x00, 0x1C, 0x38, 0x00, 0x1F, 0xF8, 0x00, 0x1C, 0x00, 0x00, 0x1F, 0xF8, 0x00, 0x00,
0x00, 0x00, 0x0F, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xC0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const uint8_t rain[] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x1C, 0x18, 0x00, 0x00,
0x00, 0x00, 0x30, 0x04, 0x00, 0x00, 0x00, 0x00, 0x60, 0x02, 0x00, 0x00, 0x00, 0x00, 0x40, 0x03,
0x00, 0x00, 0x00, 0x00, 0x80, 0x01, 0x00, 0x00, 0x00, 0x07, 0x80, 0x01, 0x80, 0x00, 0x00, 0x1E,
0x00, 0x00, 0xE0, 0x00, 0x00, 0x30, 0x00, 0x00, 0x18, 0x00, 0x00, 0x60, 0x00, 0x00, 0x08, 0x00,
0x00, 0x40, 0x00, 0x00, 0x0C, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x04, 0x00, 0x00, 0xC0, 0x00, 0x00,
0x04, 0x00, 0x00, 0xC0, 0x00, 0x00, 0x04, 0x00, 0x00, 0x40, 0x00, 0x00, 0x04, 0x00, 0x00, 0x40,
0x00, 0x00, 0x0C, 0x00, 0x00, 0x60, 0x00, 0x00, 0x18, 0x00, 0x00, 0x38, 0x00, 0x00, 0x30, 0x00,
0x00, 0x0F, 0xFF, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x03, 0x01,
0x80, 0x00, 0x00, 0x0F, 0x07, 0x03, 0xC0, 0x00, 0x00, 0x1B, 0x09, 0x04, 0xC0, 0x00, 0x00, 0x32,
0x19, 0x0C, 0x80, 0x00, 0x00, 0x32, 0x19, 0x0C, 0x80, 0x00, 0x00, 0x1E, 0x0F, 0x07, 0x00, 0x00,
0x00, 0x00, 0x00, 0x20, 0x00, 0x00, 0x00, 0x00, 0xE0, 0x70, 0x00, 0x00, 0x00, 0x00, 0xA0, 0xD0,
0x00, 0x00, 0x00, 0x03, 0xA1, 0x10, 0x00, 0x00, 0x00, 0x02, 0x21, 0x30, 0x00, 0x00, 0x00, 0x03,
0x61, 0xB0, 0x00, 0x00, 0x00, 0x01, 0xC0, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00};
const uint8_t temperature[] PROGMEM = {
0x00, 0x00, 0x1F, 0x80, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xC0, 0x00, 0x00, 0x00, 0x00, 0xF9, 0xE0,
0x00, 0x00, 0x00, 0x01, 0xE0, 0x70, 0x00, 0x00, 0x00, 0x01, 0xC0, 0x70, 0x00, 0x00, 0x00, 0x03,
0x80, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x80, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x80, 0x38, 0x00, 0x00,
0x00, 0x03, 0x80, 0x38, 0x00, 0x00, 0x00, 0x03, 0x80, 0x38, 0x00, 0x00, 0x00, 0x03, 0x80, 0x39,
0xFC, 0x00, 0x00, 0x03, 0x80, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x8E, 0x38, 0x00, 0x00, 0x00, 0x03,
0x9F, 0x38, 0x00, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00,
0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x38, 0x00, 0x00, 0x00, 0x03, 0x9F, 0x38,
0x00, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03,
0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x38, 0x00, 0x00, 0x00, 0x03, 0x9F, 0x38, 0x00, 0x00,
0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x39, 0xFC, 0x00, 0x00, 0x03, 0x9F, 0x38,
0x00, 0x00, 0x00, 0x07, 0x9F, 0x3C, 0x00, 0x00, 0x00, 0x0F, 0x1F, 0x1E, 0x00, 0x00, 0x00, 0x1E,
0x3F, 0x87, 0x00, 0x00, 0x00, 0x1C, 0x7F, 0xC7, 0x00, 0x00, 0x00, 0x38, 0xFF, 0xE3, 0x80, 0x00,
0x00, 0x39, 0xFF, 0xF3, 0x80, 0x00, 0x00, 0x31, 0xFF, 0xF1, 0x80, 0x00, 0x00, 0x73, 0xFF, 0xF9,
0xC0, 0x00, 0x00, 0x73, 0xFF, 0xF9, 0xC0, 0x00, 0x00, 0x73, 0xFF, 0xF9, 0xC0, 0x00, 0x00, 0x73,
0xFF, 0xF9, 0xC0, 0x00, 0x00, 0x31, 0xFF, 0xF1, 0x80, 0x00, 0x00, 0x39, 0xFF, 0xF1, 0x80, 0x00,
0x00, 0x38, 0xFF, 0xE3, 0x80, 0x00, 0x00, 0x1C, 0x7F, 0xC7, 0x00, 0x00, 0x00, 0x1E, 0x3F, 0x87,
0x00, 0x00, 0x00, 0x0F, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x07, 0x80, 0x3C, 0x00, 0x00, 0x00, 0x03,
0xF1, 0xF8, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x80, 0x00, 0x00
};
const uint8_t sunny[] PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x83, 0x04, 0x00, 0x00, 0x00, 0x00,
0x83, 0x04, 0x00, 0x00, 0x00, 0x00, 0xC3, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x40, 0x08, 0x00, 0x00,
0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x00, 0x10, 0x30, 0x38, 0x20, 0x00, 0x00, 0x18, 0xC0, 0x0C,
0x60, 0x00, 0x00, 0x0C, 0x80, 0x04, 0xC0, 0x00, 0x00, 0x01, 0x80, 0x02, 0x00, 0x00, 0x00, 0x01,
0x00, 0x03, 0x00, 0x00, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x00, 0x02, 0x00, 0x01, 0x00, 0x00,
0x00, 0x72, 0x00, 0x01, 0x38, 0x00, 0x00, 0x72, 0x00, 0x01, 0x38, 0x00, 0x00, 0x02, 0x00, 0x01,
0x00, 0x00, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0x00, 0x03, 0x00, 0x00, 0x00, 0x01,
0x00, 0x02, 0x00, 0x00, 0x00, 0x04, 0x80, 0x06, 0x80, 0x00, 0x00, 0x1C, 0xC0, 0x0C, 0xE0, 0x00,
0x00, 0x10, 0x70, 0x38, 0x20, 0x00, 0x00, 0x00, 0x1F, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x40, 0x08,
0x00, 0x00, 0x00, 0x00, 0x43, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x83, 0x04, 0x00, 0x00, 0x00, 0x00,
0x83, 0x04, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
const uint8_t cloudy[] PROGMEM= {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0x02, 0x08, 0x40, 0x00,
0x00, 0x00, 0x03, 0x08, 0x40, 0x00, 0x00, 0x00, 0x01, 0x00, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x3C,
0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x31, 0x81, 0x8C, 0x00, 0x00, 0x00,
0x1B, 0x00, 0xD8, 0x00, 0x00, 0x00, 0x02, 0x00, 0x60, 0x00, 0x00, 0x00, 0x06, 0x00, 0x20, 0x00,
0x00, 0x00, 0x3F, 0x80, 0x20, 0x00, 0x00, 0x00, 0xE0, 0xC0, 0x10, 0x00, 0x00, 0x00, 0x80, 0x60,
0x17, 0x00, 0x00, 0x01, 0x80, 0x30, 0x10, 0x00, 0x00, 0x03, 0x00, 0x10, 0x10, 0x00, 0x00, 0x02,
0x00, 0x10, 0x20, 0x00, 0x00, 0x1E, 0x00, 0x1C, 0x20, 0x00, 0x00, 0x7C, 0x00, 0x0E, 0x48, 0x00,
0x00, 0xC0, 0x00, 0x01, 0xCC, 0x00, 0x00, 0x80, 0x00, 0x01, 0x84, 0x00, 0x01, 0x80, 0x00, 0x00,
0x80, 0x00, 0x01, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x00, 0x00, 0x00, 0xC0, 0x00, 0x01, 0x00,
0x00, 0x00, 0xC0, 0x00, 0x01, 0x00, 0x00, 0x00, 0x80, 0x00, 0x01, 0x80, 0x00, 0x00, 0x80, 0x00,
0x00, 0xC0, 0x00, 0x01, 0x80, 0x00, 0x00, 0x60, 0x00, 0x03, 0x00, 0x00, 0x00, 0x3F, 0xFF, 0xFE,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
void draw(void) {
// graphic commands to redraw the complete screen should be placed here
u8g.setFont(u8g_font_8x13);
u8g.setPrintPos(5,10);
u8g.print("Weather Station");
u8g.setPrintPos(55,30);
u8g.print("By");
u8g.setPrintPos(2,50);
u8g.print("The IoT Projects");
}
void draw_4(void){
pressure=bmp.readPressure()/100;
delay(1);
u8g.setPrintPos(32,10);
u8g.print("Pressure");
u8g.setPrintPos(65,45);
u8g.print(pressure);
u8g.setPrintPos(70,60);
u8g.print("hPa");
u8g.drawBitmapP( 0, 15, 6, 48,presure);
}
void draw_2(void){
u8g.setFont(u8g_font_8x13);
u8g.setPrintPos(0,10);
u8g.print(" Temperature");
u8g.setPrintPos(55,40);
u8g.print(temp);
u8g.setPrintPos(97,40);
u8g.print("C");
u8g.drawBitmapP( 0, 17, 6,48,temperature);
}
void draw3(void){
u8g.setPrintPos(3,10);
u8g.print("Weather Forecast");
if ((pressure<=1000.59)&&(pressure>998.5)){
u8g.drawBitmapP( 10, 15, 6, 48,cloudy);
u8g.setPrintPos(60,45);
u8g.print("Cloudy");
}
else if((pressure<998.5)&&(pressure>996.5))
{
u8g.drawBitmapP( 10, 15, 6, 48,rain);
u8g.setPrintPos(66,38);
u8g.print("Rain");}
else if((pressure>1000.6)&&(pressure<1050)) { u8g.drawBitmapP( 5, 15, 6, 48,sunny); u8g.setPrintPos(50,45); u8g.print("Clear Sky");} else if((pressure>990)&&(pressure<=996.4))
{
u8g.drawBitmapP( 5, 15, 6, 48,storm);
u8g.setPrintPos(60,45);
u8g.print("Storm");}
else{
u8g.setPrintPos(40,40);
u8g.print("Error!");
}
}
void setup() {
Serial.begin(9600);
Serial.println(F("BMP280 test"));
if (!bmp.begin()) {
Serial.println(F("Could not find a valid BMP280 sensor, check wiring!"));
while (1);
}
u8g.firstPage(); // first page
do {
draw();
}
while( u8g.nextPage() );
delay(2000);
}
void loop() {
temp=bmp.readTemperature();
u8g.firstPage(); // Next page
do {
draw_2();
}
while( u8g.nextPage() );
delay(2000);
u8g.firstPage(); // Next page
do {
draw_4();
}
while( u8g.nextPage() );
delay(2000);
u8g.firstPage(); // Next page
do {
draw3();
}
while( u8g.nextPage() );
delay(2000);
}
After successful upload of the program code, open the serial monitor. You can see the BMP280 Sensor test is running. If you see the error message like: “Could not find a valid BMP280 sensor, check wiring” then you need to change your BMP280 default I2C address in the Adafruit_BMP280.h file.

The Adafruit_BMP280.h file located at: DocumentsArduinolibrariesAdafruit_BMP280_Library. For reference, check the image below.

Now, re-upload the code and check your Serial Monitor. Finally, you got the temperature, barometric pressure data, and weather forecasting data on OLED Display.
Demonstration: BME280 based Weather Station
Now, if you have followed all the mentioned steps and connected everything accordingly, then you should see the weather station data on your OLED display without any errors.
The video tutorial on BMP280 Based Weather Station using Arduino and OLED Display is embedded below:
Conclusion
So, this is another BMP280 Based Weather Station using Arduino and OLED Display which will be able to monitor the temperature, atmospheric pressure, and altitude. I hope you love this project, if you did, please share it with others.
Excellent project!
Hey! tried to replicate your project. uploaded sketch to arduino. The screen shows small dots and that’s it. what am I doing wrong? arduino nano, bmp280, ssd1306
il faux isolé ou supprimé
//#define BMP_SCK 13
//#define BMP_MISO 12
//#define BMP_MOSI 11
//#define BMP_CS 10
et mettre ceci dans le void setup pour la connexion I2C
void setup() {
Serial.begin(9600);
if (!bmp.begin(0x76)) {
Serial.println(“Could not find a valid BMP280 sensor, check wiring!”);
while (1);
APARECEN PUNTITOS EN LA PANTALLA OLEP, QUE PUEDO HACER PARA ARREGLAR ESO, AYUDA PORFAVOR
Super
Witam
W tym układzie bmp280 podaje złą temperature o +2C jak można to zmienic