Top 10 IoT (Internet of Things) Projects
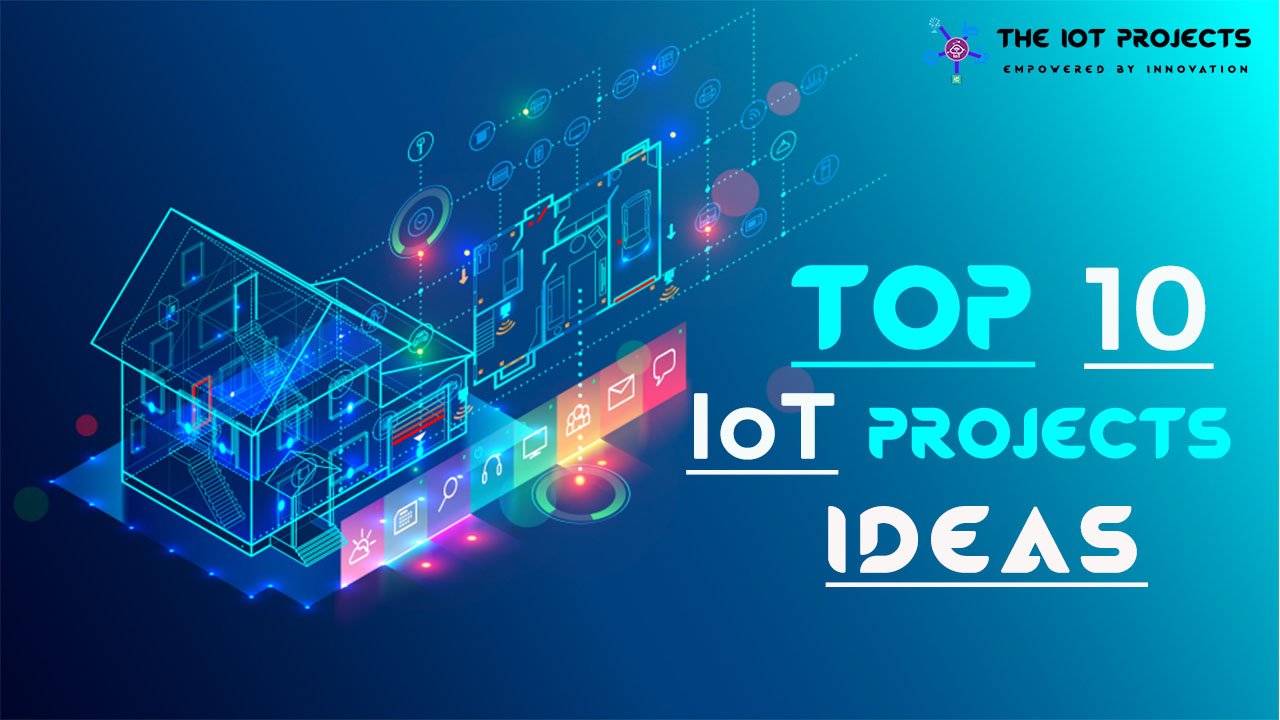
The IoT Projects Welcome you to this interesting article on Top 10 Internet of Things Projects. Now in this article, we’ll be talking about the Top 10 Projects which are outstanding themselves in the Internet of Things. Now before talking about each of these projects let me start off with a simple introduction to what is the Internet of Things?
Internet of Things basically is a concept or a technology that aims to connect all the devices to the Internet. And help them communicate with one another using the internet as a medium. Basically, these devices can be anything, it could be a TV, Mobile phone, watch or even your car. Actually, anything that can be connected to the Internet can be considered as a device for the Internet of Things (IoT).
The intention of using the Internet as a medium of communication is to help you to achieve a wider and greater reach. But, the end aim of the Internet of Things is to help you create a smart world. So, this was a short introduction to what is the Internet of Things (IoT). You can read more about IoT in our previous post. Now let’s talk about each of these projects.
Air Pollution Monitoring System
Air pollution is a major common problem in today’s world. Usually, Air pollution brings lots of diseases. As you know every month people suffer from a new disease. Let’s take an example now coronavirus is spreading all around the world. One of the main reasons behind the spreading of this virus is due to polluted air. Different particles like mold spores, lead, carbon dioxide, sulfur dioxide, and pollen are the main factors for polluting air. Hence, this polluted air brings lots of new diseases.
Nowadays, it is very essential to measure and monitor the air pollution level in an area. Different Research on IoT projects has brought a new solution. Recently, invented IoT devices can do regular monitoring of air pollution levels and save data to the web servers.
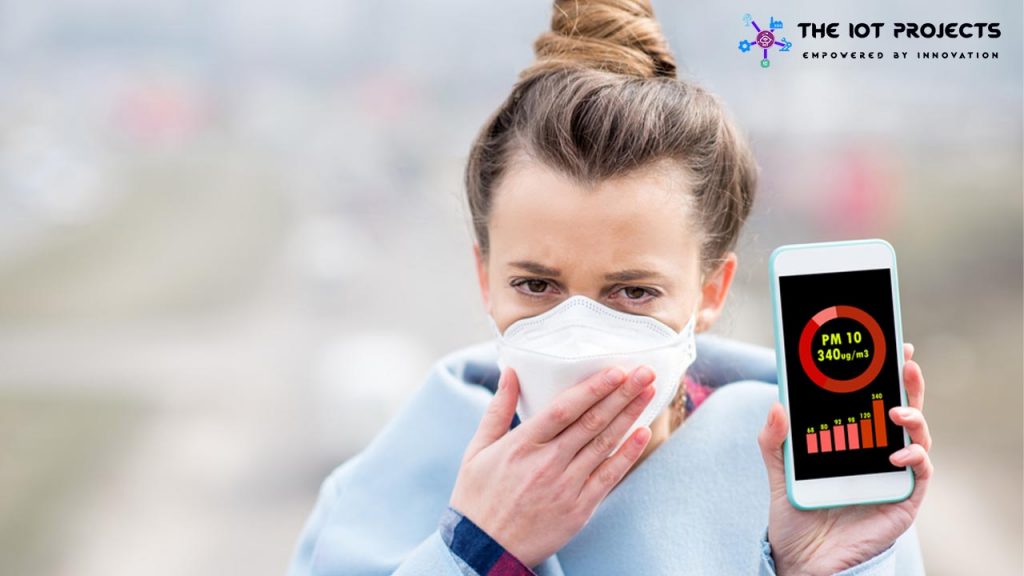
Usually, IoT project inventions like air pollution meters bring a solution to the existing problems. Unlike previous air pollution meters, this device uses the Internet to save data to the webserver. Hence this device will not get out of Memory and we can check log data remotely from anywhere in the world. This project is built on the Arduino Uno platform. The main feature of this project is it can be used to detect flammable gas leaks as well.
Street Light Monitoring System
According to our research, street light consumes more than 20% of the world’s energy. Most of the street lights remain ON all the time due to the lack of a light monitoring system. But, newly discovered ideas like IoT based street lights monitoring system brings a solution. Basically, this project consists of sensors that monitor vehicles and people around the lights. This means when the sensor detects any movement the lights are ON otherwise remains close.
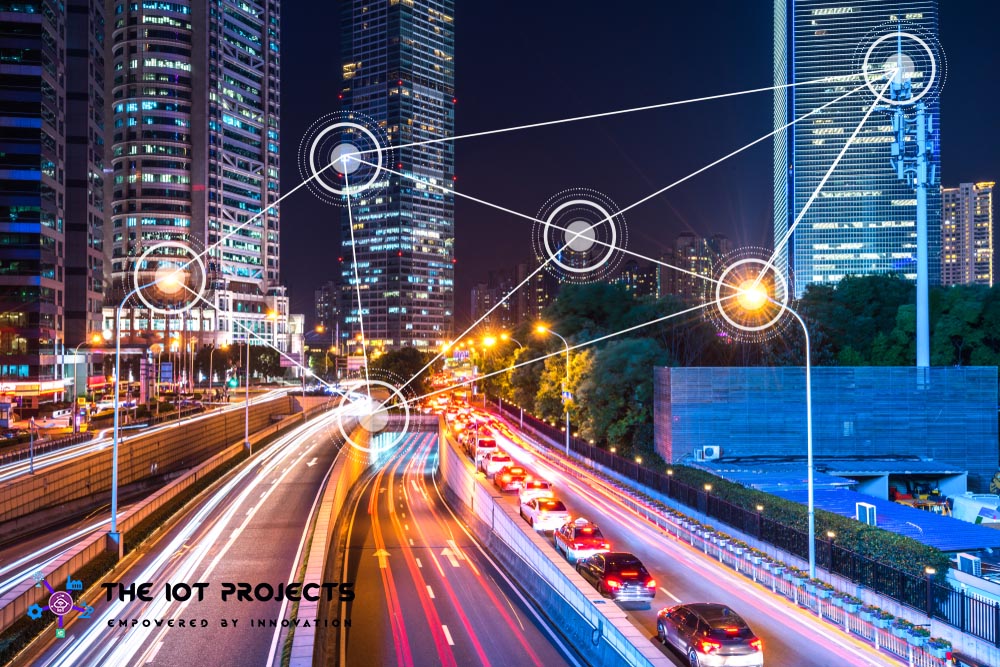
Usually, the device sense the movement around it and then the sensor sends a signal to the Microcontroller. After that, the device takes action according to the received signal. If no movement is found Microcontrollers Switch Off the lights. Actually, with this IoT project, we can save lots of energy. It has special features like automatically shut down during day time, which saves energy.
Smart Irrigation System
Next, we have a Smart Irrigation System. Let you are someone who personally likes gardening a lot. Now, this is something that would really make your life easier. Because usually what you can do is that on Sundays you spend your two hours just watering and looking after your plants. Through a smart irrigation system what it does is that it checks the moisture present within the environment. Or the moisture present in the soil using the sensor. Again, to help you understand how it works? Usually, there are two main Internet of Things devices that you can use which are the Adreno board and the Raspberry Pi. Your Raspberry Pi becomes the main processing unit and you can place an Arduino board for each of your water channels. These Arduino nodes themselves are connected to multiple sensors that are part of this water channel.
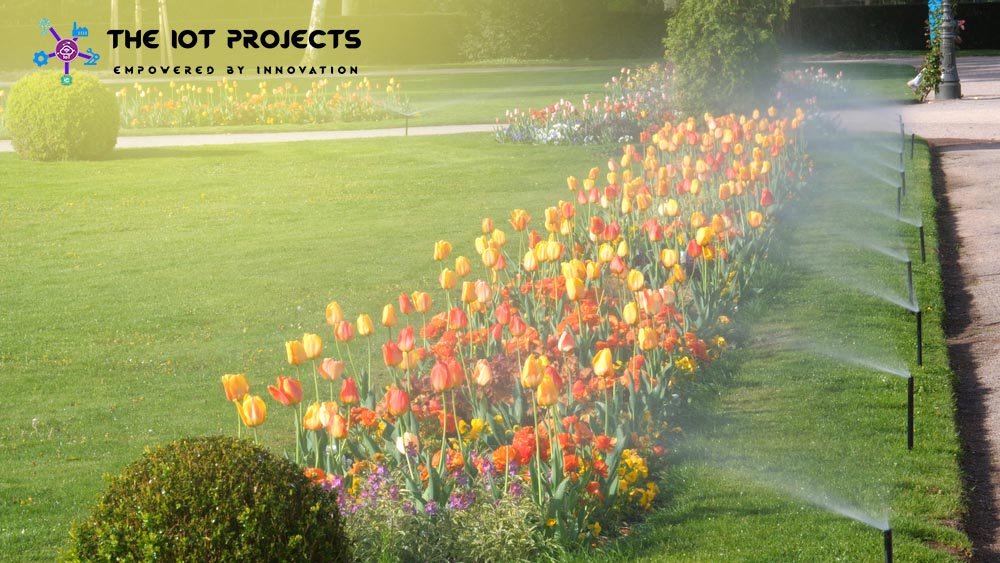
So what these sensors do is that they check the moisture present in the soil. As such, let’s assume that a specific link does not meet the minimum required moisture. Then what it would do is that it would send a signal to the Raspberry Pi. Again all these devices are connected on the same wireless router network. Now, the Raspberry Pi would identify the lack of moisture and pass a signal to the relay. The relay, in turn, would initiate the water pump and water would be irrigated.
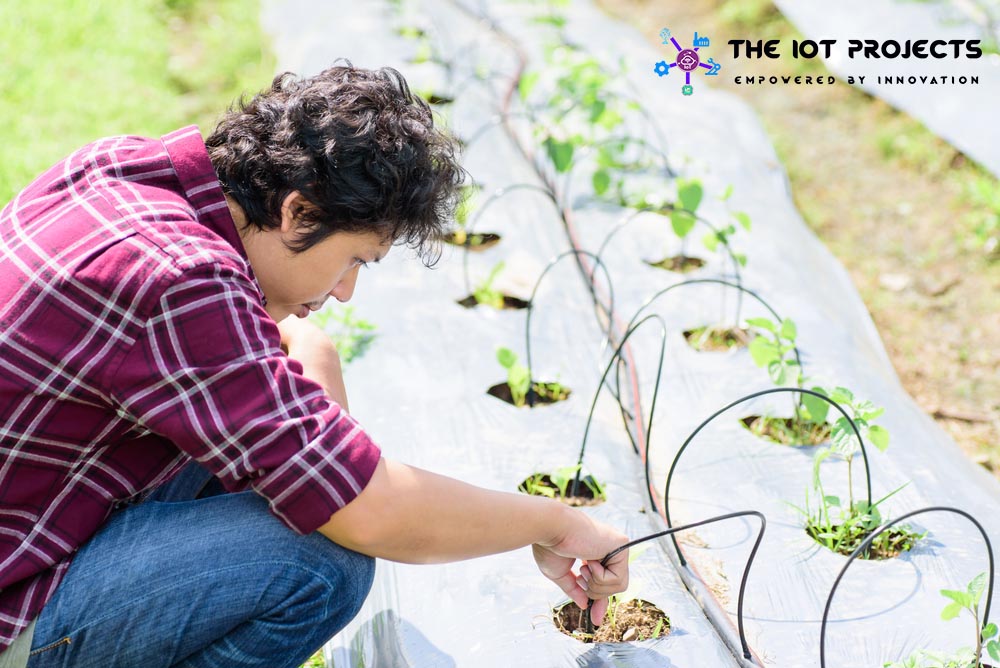
Now in order to ensure that water is not wasted. We would create gate controls. Only the gate where the moisture is a smaller amount would the gate be open. Once your sensor detects that the moisture level has gone beyond my required limit, it would again send another signal to the Raspberry Pi. Asking it to stop the pump as well. Hence, this, in turn, helps you to save a lot of water and also makes your life quite easier. So after this, your only task in your garden would be either setting up new plans or creating a new water channel.
Biometric System
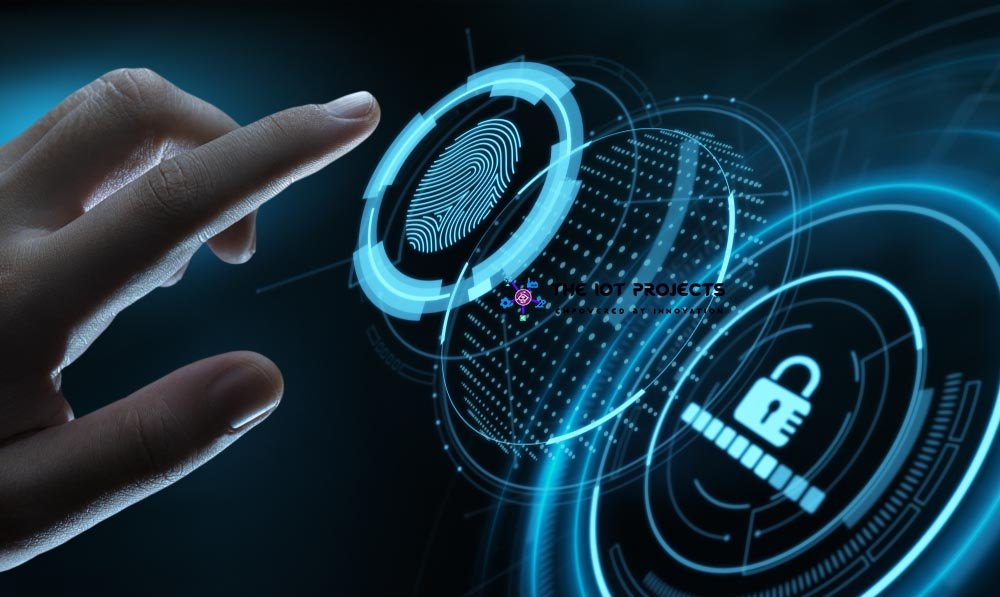
A biometric system is something that we always encounter on a daily basis. Because we always either use a fingerprint sensor or use an iris scanning even to unlock our phones. Being on the age of 2020 attendance at school, colleges, and offices is done with A Biometric System. So, it depends from organization to organization. But, how does this System actually work?
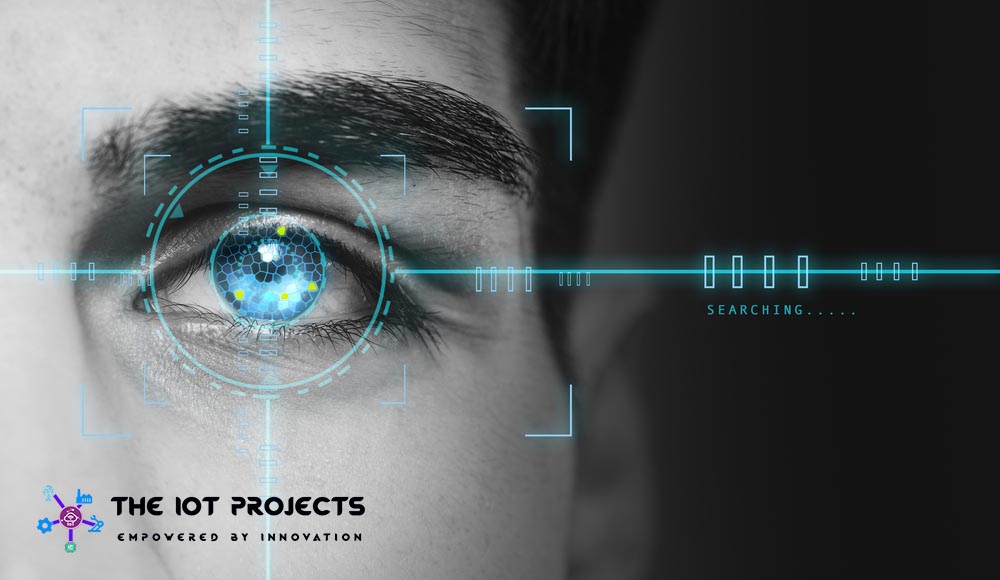
Let us take a case of this example. let the system have a fingerprint scanner. Now when you are presenting your fingerprint for the first time. The system scans the fingerprint and considers this as part of an enrollment process. From this fingerprint template what it does is, It extracts certain key features that make it different from others. Then stores it into a database. After this process, the system forwards the data every time that I placed my finger on top of this fingerprint scanner. Firstly, it creates a template and compares this with all the templates that are present in the database.
Secondly, if it matches then correspondingly it gives you attendance. or lets me access a Door. Finally, if it does not match the Biometric data, then it raises an alert. Again, this is just a foundation. As I said this biometric system can be a fingerprint, Iris scanning or it can be a combination of both as well. The voice recognition system is one of the key products in the Biometric System.
Security Camera and Door Unlock System
Next, the project on our list is a security camera and door unlock system. Now, this is something that’s quite interesting. I’ve personally tried to start out and it’s really something that you just should try out. Here what happens is that you simply place a camera on top foot door which successively clicks the photo of an individual, who comes into the frame. Now, this photo is sent to an analytical system that compares this with all the photos that it possesses.
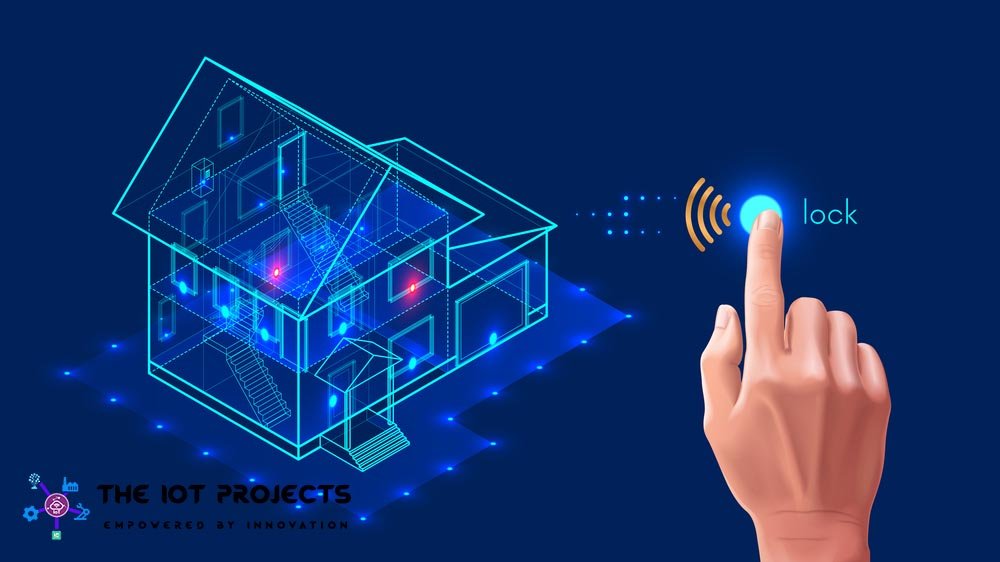
In order to identify whether to let the user open the door or not. Basically, an evolution to this is that if it does not find a photo of that person. It can notify the concern that this person is trying to access this door. Would you like to authorize this person? Also, the system adds his information to the database or would you like to deny access to this person as well. Usually, this is used in areas where you have highly sensitive information stored. In order to maintain strict control over access to this information. Another usage of the security camera and unlock system can be even useful at our homes. Using this system we can identify who is coming to our home when we’re not there. And either decide to give them access to our homes or not as well.
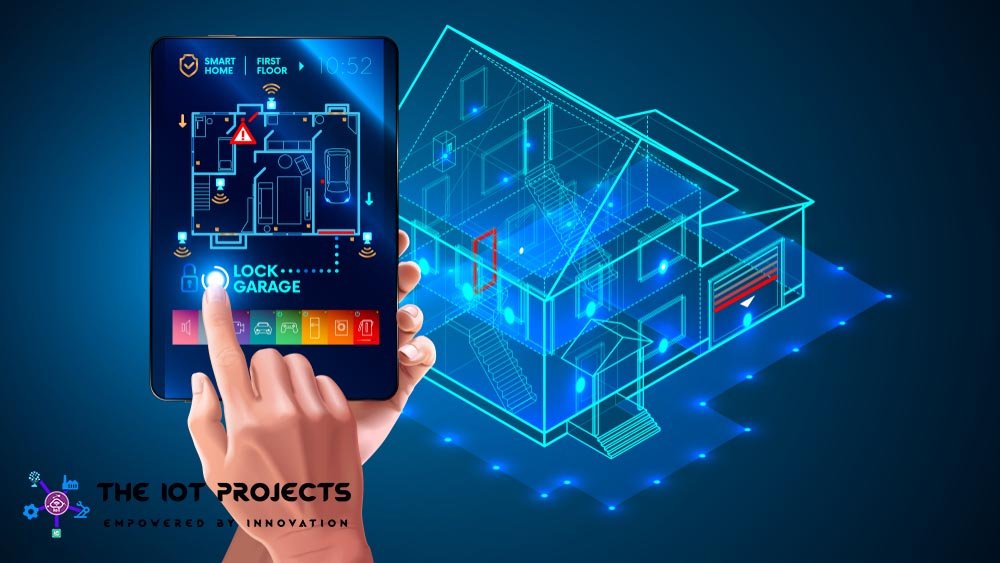
Smart Home System
Now the next thing is a few things that we all really desire to possess which could be a smart home system. The smart home system can be something that really makes our life quite easy. Starting from energy management where the light control system, the AC, the appliances that we use, the thermostat all of these things are managed.
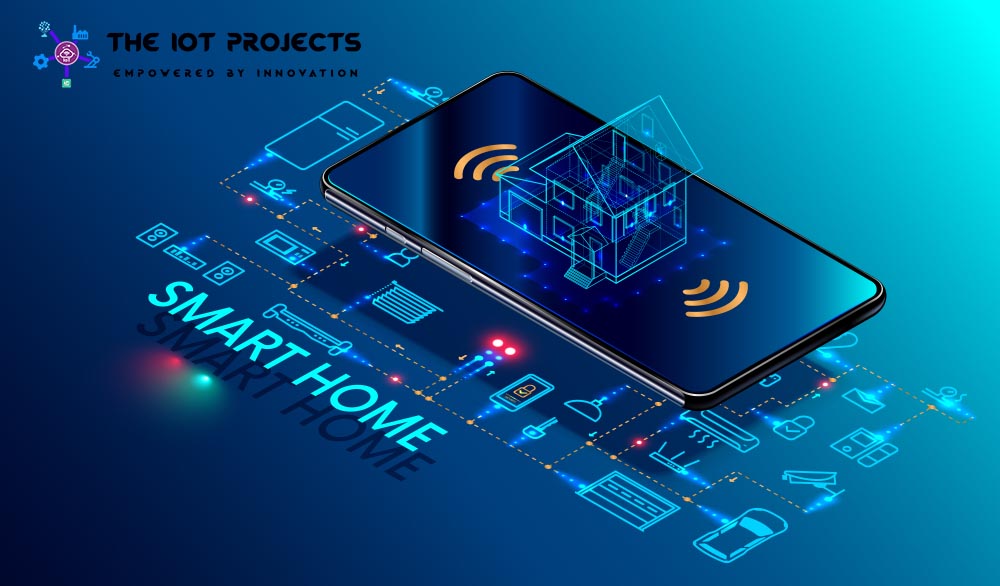
In short, trying to cut down the power consumption that’s taking place in my door management system is also part of this Smart Home System. It is also part of my water irrigation system as well. Again, these are key things that really stand out in the smart home system. But, again what I would personally recommend is that the limitations of a smart home are where our imagination stops. Anything that you wish to automate or may wish to make your life easier can be part of a Smart Home System. Basically, a smart home System usually is going to be a base for our next project which is a Smart City.
Smart City
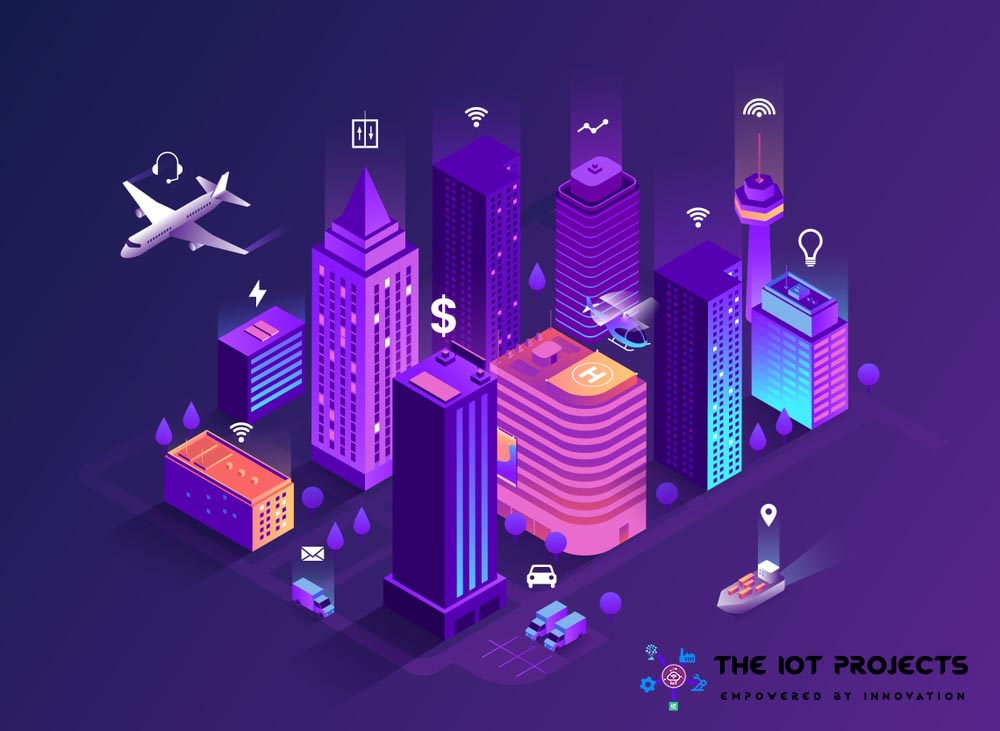
Actually, A smart city is an evolution of a smart home. Here it’s not just the sensors of a single home that is connected. Smart City is the correlation or a network or a connection between various organizations’ various domains as well as various segments of that city. The life of every single dependent person in that city becomes easier as a whole additionally. In turn, it will really help to develop that city to a greater extent. Now, the key factor here for a smart city is government support as well. If the government is really willing to take this step then I hope we would see a smart city completely built on the Internet of Things. Maybe in the next five to ten years, we can see this project in real life.
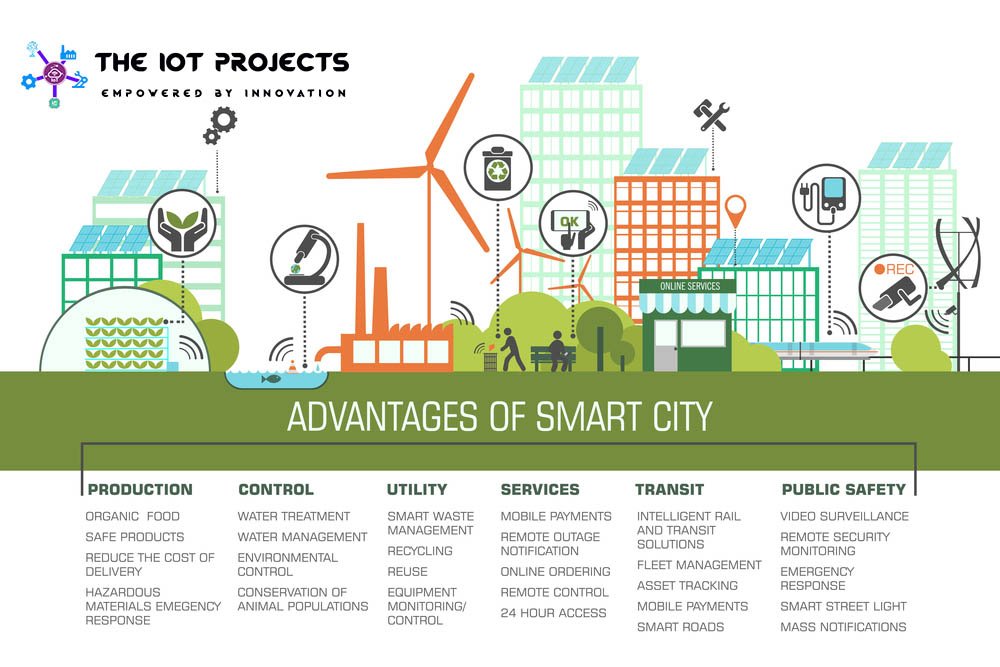
Zelda Ocarina
The next project is something that really stands out on a personal level. This is Zelda Ocarina’s controlled home automation system. This is personally something that I feel is the closest to a smart home system. Well, most of the elements of the home are completely controlled by an ocarina. What Allen pan has done here is that he’s created a node-based recognition system that completely automates his home. But, rather than telling more about this. let’s just look at a quick video that will give you a glimpse into how he has done this and what has he done?
Smart Parking System
Nowadays, Finding parking space is one of the problems for the driver. Sometimes lots of time is wasted to find a parking spot. But, now the IoT based project called smart parking system brings a solution. The main theme and purpose of discovering this project are to avoid unnecessary traveling by a driver for the parking spot. Basically, this device monitors the whole area even while driving and provides an entire image of the parking spot. It also provides a facility to choose the free parking space and park their vehicles.
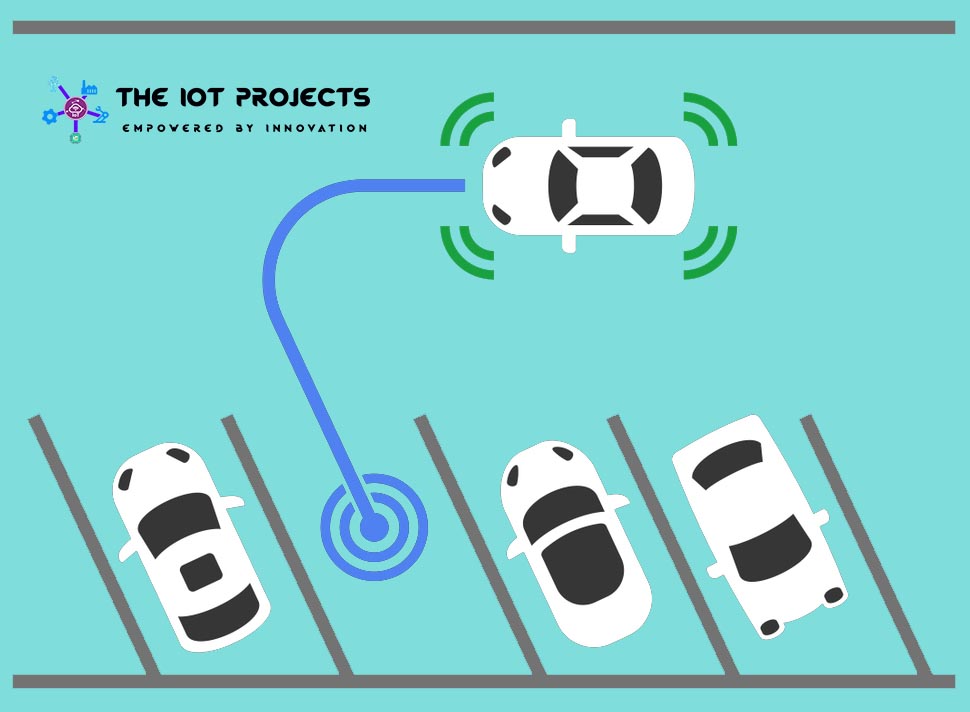
This project is completely based on the Daily Life Problem solution using IoT. The infrared distance sensor and IR Sensor can be used for discovering free parking spaces.
Jarvis Personal Assistant
The number one IoT project if you’ve not already guessed is Javis. Javis is the artificial intelligence system that Facebook’s creator Mark Zuckerberg has built for his home automated system. I am quite sure you’ve already seen the video of Mark Zuckerberg interacting with Jarvis that has Morgan Freeman’s voice. If you have not here is a quick glimpse into the same.
Final Thoughts
Now what you need to understand here is that the Internet of things is not something that is just dependent on a sensor or a few sensors which are connected to a Raspberry Pi or an Arduino. When I check out the whole architecture of IoT. This is a complete ecosystem where my sensors gather information. That is again stored on a platform and then processed.
If there have been any issues or failures that have been reported by these sensors then, I really need to create actionable items in order to ensure that this is never really repeated again. But, the information, once it’s been processed the processed data, is then passed into machine learning and artificial intelligence in order to understand analyze and identify various patterns. It also helps to pass this information back to the sensor. This, in turn, helps you to have a better experience and also improve the system as a whole.
A system where I come home every day at 8:30 start off my AC and wait for 15 minutes for it to cool down. This is really a tedious process. But, today my Internet of Things (IoT) platform identifies this pattern and already switches on the AC at 8:15. Then I just need to come home and I can relax right away.
This is one of the key examples or one of the key ideas that are out there today and the limitation here again is just your imagination.
Conclusion on Top 10 IoT Internet of Things Projects
You can find lots of IoT projects on the Internet. But, These Top 10 IoT Internet of Things Projects presented here will change our lifestyle. We are still working on IoT based Projects. Because it is a blessing to the new world with a huge source of solutions.
So with this, we came to the conclusion of this Top 10 IoT Internet of Things Projects. But, we are going to come up with a lot more interesting articles and various Projects on IoT (Internet of Things). So if there’s some project that really you want to know more about please allow us to know within the comment section below.
With this thank you and goodbye I hope you have enjoyed reading this article. Please be kind enough to share it on social media. You can comment on any of your doubts and queries and we will reply to them as soon as possible. Actually, you subscribe to our website newsletter to learn more happily.
You Love IoT Projects? Some of them are here:
- NodeMCU ESP8266 Monitoring DHT11/DHT22 Temperature and Humidity with Local Web Server
- RFID Based Attendance System Using NodeMCU with PHP Web App
- Temperature Controlled Home Automation using Arduino
- IoT Based Voice Controlled Home Automation Using NodeMCU & Android
- ESP8266 based Coronavirus Tracker for your country
- Internet Clock Using NodeMCU ESP8266 and 16×2 LCD without RTC Module
- IoT Based Flood Monitoring System Using NodeMCU & Thingspeak
- Home Automation with MIT App Inventor and ESP8266
- Capacitive Soil Moisture Sensor with OLED Display & Arduino
firstly I want to say the recommendable job. I appreciate and am thankful that you’ve shared your knowledge with us.
You’ll also agree with this statement IoT rapidly increasing day by day. You have shared very useful information with us. IoT devices now used in health industries projects vary widely, and their results are positive and worthful. UbiBot MS1 is an electronic device created to measure and detect the movement of its surroundings in hospitals. You can purchase This device from UbiBot Online Store & also they’ve shared the details about the product which makes it easy to understand and will make your choice strong.