IoT vs IIoT and its protocols
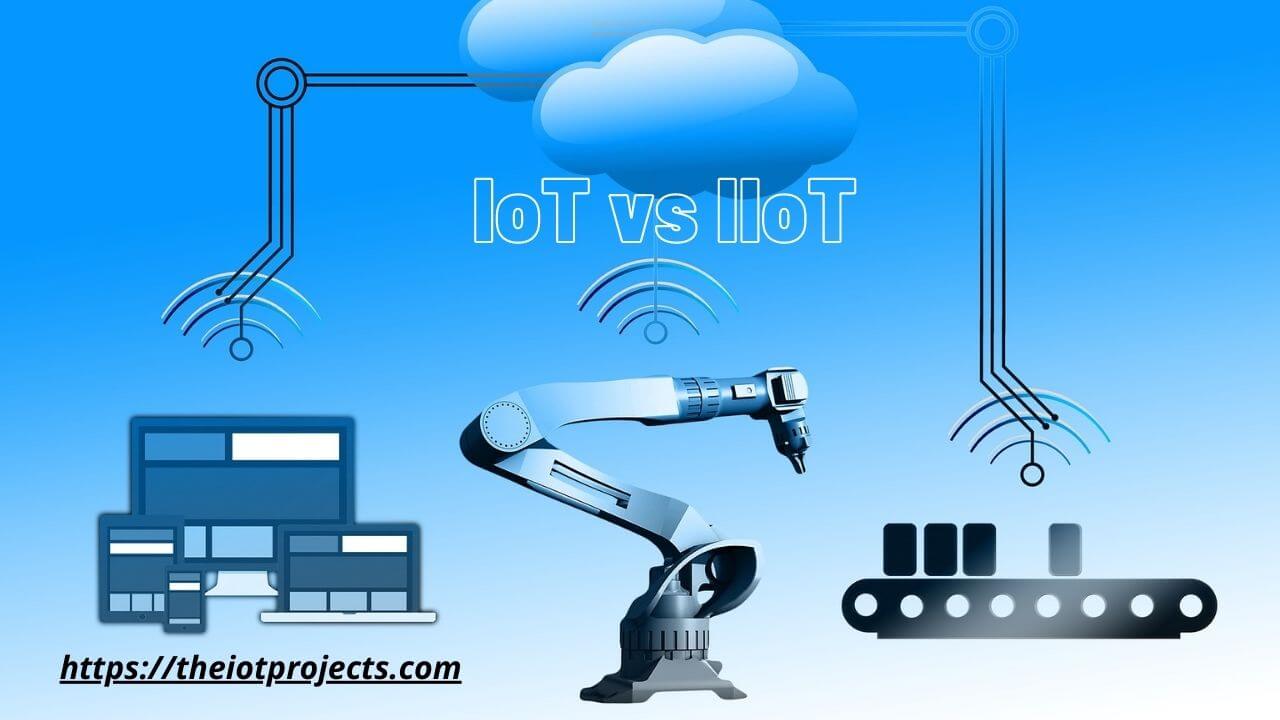
Today’s world is adopting new working technology to make human tasks faster and easier. Yes! Now we’re going to discuss one of those technologies, the Internet of Things (IoT) and Industrial Internet of Things (IIoT).
What is (IoT) Internet of Things?
The Internet of Things, or IoT, is a system of built-in computer devices, mechanical and digital machines, objects, animals, or persons that provided with unique identifiers. They have the ability to transfer data over a network without any interaction between human-to-human or human-to- Computer.
What does a Thing Mean in IoT?
A Thing on the Internet of Things could be a patient heart monitor implant, a farm animal with a biochip transponder, a vehicle with built-in sensors to alert the driver when the tire pressure is low, or some other natural or man-made object that can be assigned an Internet Protocol (IP) address and is capable of transferring data over a network.
Recommended Reading: Top 10 Coolest IoT Devices of 2020
Protocols used in IoT
Nowadays, we can collect, send, and process data on other servers and other applications by collecting a range of objects around. The IoT protocol is a system that transfers data online. But it transfers data only when the communication network between the two connected devices is secure.
We can divide the Internet of Things protocol into two basic types: IoT Network Protocols and IoT Data Protocols.
Bluetooth
Bluetooth is one of the most widely used short-range wireless technologies. You can quickly get Bluetooth apps that provide you with wearable technology for pairing with smart gadgets. Among the IoT protocols the most recently introduced Bluetooth protocol is BLE or Bluetooth low-energy protocol. It carries the range of traditional Bluetooth with low power consumption supremacy
- Standard: Bluetooth 2.2 core specification
- Frequency: 2.4GHz (ISM)
- Range: 50-150m (Smart / BLE)
- Data rates: 1Mbps (smart / BLE)
WiFi
For IoT integration, WiFi is a favorable option according to many electronic designers. This is because of the infrastructure that it causes. It has a fast data transfer rate with the ability to control a large amount of data.
- Standard: Based on 802.11n (commonly used in homes today)
- Frequencies: 2.4GHz and 5GHz bands
- Range: about 50m
- Data rates: M00 Mbps max, but 150-200 Mbps is more specific, based on channel frequency used and number of antennas (current 802.11-AC standard should offer 500Mbps-1Gbps)
Note: This IoT protocol has its drawbacks that can consume excessive power for IoT application
ZigBee
Like Bluetooth, there is a huge user base of ZigBee. On the Internet of Things protocol, ZigBee is designed for more industrial and fewer consumers. It typically operates at a frequency of 2.4GHz. This is ideal for industrial sites where data is usually transferred between homes or buildings at small rates.
ZigBee and the popular ZigBee Remote Control are popular as well-known IoT security protocols for supplying secure, low-power, scalable solutions with high node calculations. ZigBee 3.0 takes the protocol to a single standard.
- Standard: ZigBee 3.0 is based on IEEE802.15.4
- Frequency: 2.4GHz
- Range: 10-100 meters
- Data rates: 250kbps
MQTT IoT
MQTT IoT is a messaging protocol and full form Message Queue Telemetry Transport. MQTT’s main task is to get data from multiple electrical devices. It works on top of TCP for providing reliable but simple streams of data.
This MQTT protocol is made up of three core components or mechanisms: Subscribers/Members, Publishers, and Brokers. The work of the publisher is generating data and transmitting the data to the client with the help of the broker. It is the job of the broker to ensure security. It does this by checking and verifying the authenticity of Subscribers and publishers.
CoAP
COAP is designed to enable simple, constrained devices to join IoT through constrained networks, including low bandwidth and low availability. It is commonly used for machine-to-machine (M2M) applications such as smart energy and building automation.
CoAP or constrained application protocol, Internet productivity, and utility protocol are primarily developed for restricted smart gadgets. CoAP is designed to be used between similar devices that have a similarly restricted community. It includes common nodes and devices on the Internet and various restricted networks and devices that are connected to the Internet.
DDS
Among the Internet of Things protocols, the IoT messaging protocol – DDS or Data Distribution Service – is a standard for high-performance, scalable, and real-time machine-to-machine communication. Data Distribution Service -is Developed and designed by DDA and OMG or Object Management Group. With the help of DDS, you can transfer data through both low-tracking devices and cloud platforms.
Data Distribution services involve two important layers. These are DCPS and DLRL. DCPS or Data-centric publish works by providing information to subscription customers. DLRL or Data Local Reconstruction Layer does its job by providing an interface to the data-centric public membership functionality.
NFC
NFC or Near Field Communications allows customers to connect to electronic devices, use digital content, and make contactless payment transactions. The essential task of the NFC is to expand the “contactless” card technology. It works between 4cm (between devices) by enabling the devices to share information.
IoT vs IIoT
The Internet of Things brings the physical world into the digital world, expanding in detail what information technology can achieve. By connecting digital assets to the digital realm using sensors and actuators, we can electronically monitor and manage our ‘things‘.
IIoT is the Industrial Internet of Things – it applies IoT technology to the construction industry. Also known as the Industrial Internet, IIoT focuses on improving connectivity, efficiency, scalability, time savings, and cost savings for industrial organizations, and will sometimes be used in conjunction with Industry 4.0
The difference between IoT and IIoT
S.N | IoT (Internet of things) | S.N | IIoT (Industrial Internet of Things) |
1 | Served applications can be used in all IoT verticals. This means the IoT is used not only in industrial applications but also in personal or research-oriented cases. | 1 | IIoT cannot be used in all verticals. Moreover, it means Industrial IoT is an industry application-specific platform. |
2 | Sensors used in IoT Ultimately, sensors can be of any type. For example Soil moisture, Motion Sensor, heart rate monitoring, gas sensors, or any other sensors that can be accessed through the cloud. | 2 | The sensors used in industrial IoT are the sensors that are most often used in various industries. For example instance heat, temperature, flow, air, light, vibration, and pressure sensors. |
3 | The most common type of cloud technology used in IoT is the public cloud. | 3 | But, industrial IoT uses the private cloud. By comparison, IIoT is safer than IoT. |
4 | Cybersecurity IoT is not much more secure than industrial IoT. In other words, IoT is mostly used as open access. | 4 | Moreover, industrial IoT maintains very strong cybersecurity. IIoT has been implemented in a very important area of the industry. |
5 | Operation Safety is not always very high in IoT. | 5 | Industrial IoT operation Safety is the most important thing. While the lack of safety measures leads to explosion/property damage / human death. |
6 | For example, we use IoT in agriculture or healthcare or banking. | 6 | For example, IIoT can be used in the oil or gas sector within a particular industry. |
Conclusion on IoT vs IIoT
The term IoT refers to the connection of devices (things) over the Internet so that they can collect and share data without human assistance.
The Industrial Internet of Things (IIOT) refers to the use of Internet of Things (IoT) technology in manufacturing and related industries such as agriculture, gas and oil, utilities, and transportation to build smart, self-regulating systems.
IIoT is Opening a new era of economic growth and competitiveness, it will transform companies and countries. We look to the future where the intersection of people, data, and intelligent machines will have an impact on the productivity, efficiency, and operation of industries around the world.
Recommend Readings:
- Top 10 IoT (Internet of Things) Projects
- IoT Based LED Control using Google Firebase & ESP8266
- Interfacing PIR Sensor with ESP8266 and EasyIoT
- IoT Based Patient Health Monitoring System Using ESP8266/ESP32 Web Server
- Fire Security System using Arduino & Flame Sensor
- Temperature Controlled Home Automation using Arduino
- ESP8266 Plot Sensor readings to Webserver in Real-Time Chart
- IoT Web Controlled Smart Notice Board using NodeMCU ESP8266
- Home Automation with MIT App Inventor and ESP8266