Interface NEO 6M GPS Module with Arduino
How to Interface NEO 6M GPS Module With Arduino?
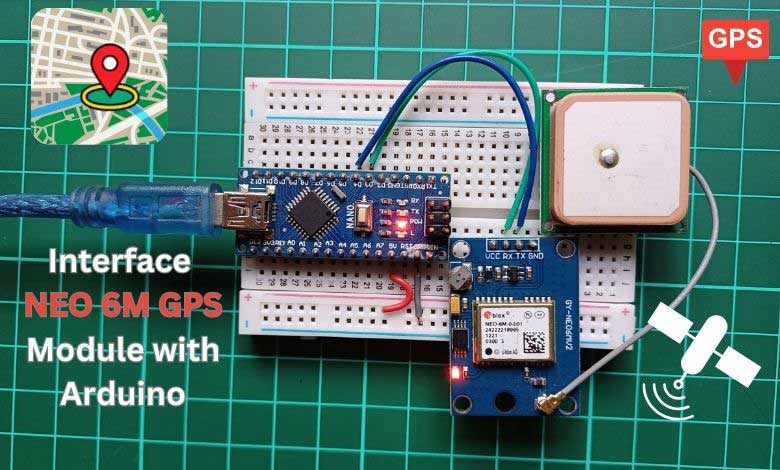
In this tutorial, You will learn to interface the NEO-6M GPS module With Arduino Microcontroller. This blog will teach you how to connect and communicate between a NEO-6M GPS module and an Arduino board to create a very basic GPS tracker. You may have seen the use of GPS trackers on many movies to track the location. Sometimes you may have thought of building your GPS tracker. So we will start from the basics knowing its key components, GPS module pinouts, interfacing it with Arduino microcontroller, and coding. At the end, you will have your basic GPS tracker which can track your real-time location on Google map.
Components Required
S.N | COMPONENTS NAME | QUANTITY | PURCHASE LINKS |
---|---|---|---|
1 | Arduino Nano | 1 | Amazon | AliExpress |
2 | Neo 6M GPS module | 1 | Amazon | AliExpress |
8 | Jumper wires | 10 | Amazon | AliExpress |
9 | Breadboard | 1 | Amazon | AliExpress |
*Please Note: These are affiliate links. I may make a commission if you buy the components through these links. I would appreciate your support in this way!
Also, check:
- Fingerprint Door Lock System using Arduino and Smartphone
- IoT based Silent Intruder Alarm using Arduino
NEO-6M GPS Module
The NEO-6M GPS module typically consists of several key components, the below image shows the parts of the Neo-6M GPS module.
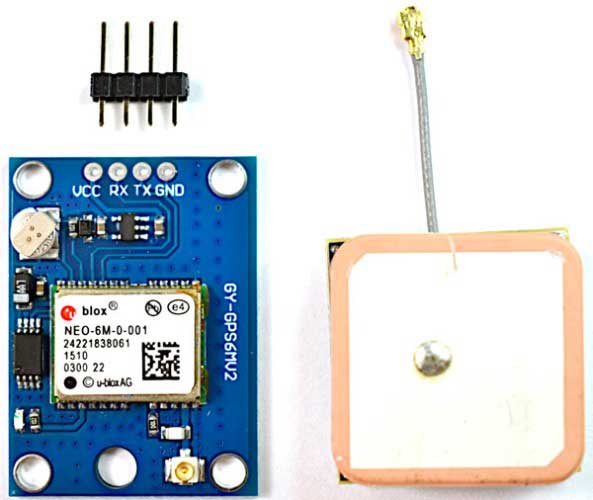
GPS Chipset
This Neo 6M GPS module consists of several small components. In the center, you can find the main GPS Cheap from Ublox. It is capable of tracking 22 satellites over 50 different countries. This is the heart of the module and is responsible for receiving signals from satellites and calculating positioning data.
Power supply components
This module features an onboard 3.3-volt regulator. It helps in converting the 5-volt signal from Arduino to the 3.3-volt required by the GPS chip. It also includes a few capacitors and other similar passive components.
Position Fix LED Indicators
You can also see an LED source as a GPS position fix indicator. It blinks every second if the GPS module is within the range of enough satellites to provide precise coordinates.
Battery-backed RAM
The battery-backed RAM consists of two main components: –
1) HK24C32 Serial EEPROM and 2) A Rechargeable button battery.
You can also see a small rechargeable battery that powers the RAM. This RAM stores essential data such as clock data, the latest GPS location, and the configuration of modules. This small battery can retain data for more than a week from the EEPROM without any power.
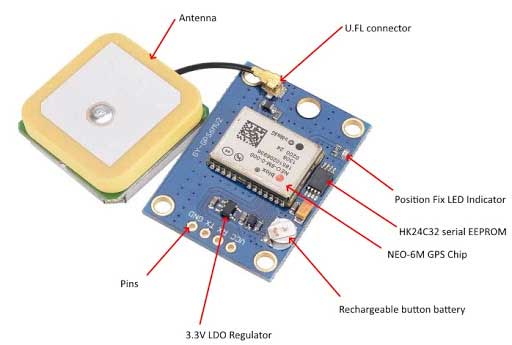
Battery-Backed RAM in NEO-6M GPS Module
Battery-backed RAM in a NEO-6M GPS module helps keep important information safe when there’s no power. This means the module remembers things like satellite details, the last place it knew you were, the time, and other key settings, even if it gets turned off. With this memory, the device can start up faster because it doesn’t have to search for satellite information all over again each time it turns on.
Antenna Connector
This part has a special antenna that works really well for picking up signals. It includes a UFL connector to connect it directly to the module.
Antenna
This is a part of the device that picks up signals from space satellites to figure out exactly where it is. This helps the device know its location with precision. Below is a picture showing the NEO 6M GPS Module with its Antenna.
NEO-6M GPS module Pinouts
The NEO 6M GPS module is a small device that helps in finding the location. It has four important connection points:
- VCC: This is where you connect the power to make the module work.
- GND: This is the ground pin, which you connect to the negative side of the power source.
- TX: This pin sends data out from the GPS module.
- RX: This pin receives data into the GPS module.
The image below will show you exactly where these pins are on the NEO6MV2 GPS module.
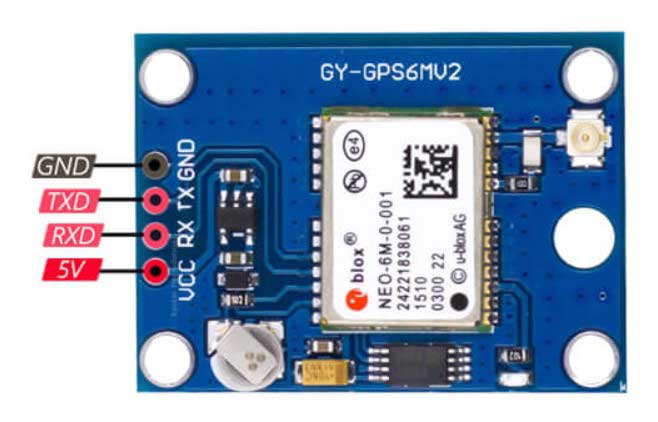
How Does a NEO-6M GPS Module Work?
The NEO-6M GPS module is like a digital compass, but much more advanced. It works by getting messages from lots of satellites up in the sky, which allows it to find out exactly where it is on Earth. The module is great at saving energy and can talk to up to 22 satellites at once.
The module uses invisible waves, like the ones used in radios, to get important information from each satellite. It learns where the satellite is and how long it took for the messages to arrive. With this data, the NEO-6M can calculate its latitude, longitude, altitude, time, and other relevant data.
You can connect this module to a microcontroller. It uses two special pins, RX and TX, to send the location information it gathers. This is helpful for things that need to know their location, like cars with GPS for directions, making maps, or tracking where something is.
Interface Neo 6M GPS Module with Arduino
To Interface Neo 6M GPS Module with Arduino we will use UART (Universal Asynchronous Receiver /Transmitter) protocol. You can check out the Arduino GPS Module Circuit Diagram below.
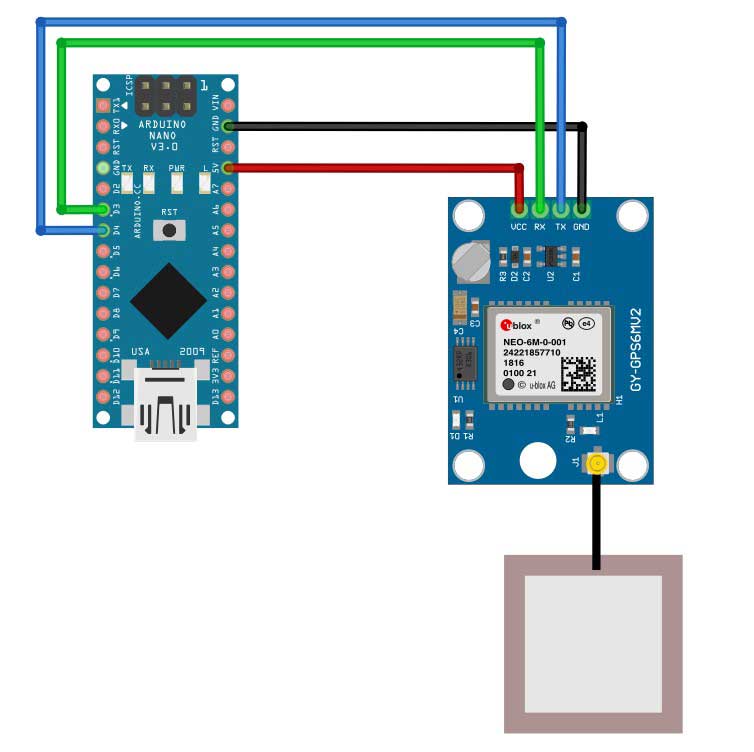
The VCC pin of the GPS module is connected to the 5V pin of the Arduino. The GND pin is connected to the GND. Similarly, the RX pin of the GPS module to the D3 pin of the Arduino and the TX pin of the GPS module to the D4 pin of the Arduino.
Arduino Code for NEO-6M GPS Module
The code provided below helps your device communicate with the GPS module. It also fetches and shows important information such as the current longitude and latitude, the current date, and the time.
The library you need to include before you can start uploading the code can be found in Sketch> Include> Manage Libraries and write “tinygpsplus” by Mikal Hart and then Install it. Special thanks to Mikalhart, for his awesome library.
Arduino GPS Tracker Code
//Arduino GPS Module Code #include <TinyGPSPlus.h> #include <SoftwareSerial.h> /* This sample sketch demonstrates the normal use of a TinyGPSPlus (TinyGPSPlus) object. It requires the use of SoftwareSerial, and assumes that you have a 4800-baud serial GPS device hooked up on pins 4(rx) and 3(tx). */ static const int RXPin = 3, TXPin = 2; static const uint32_t GPSBaud = 9600; // The TinyGPSPlus object TinyGPSPlus gps; // The serial connection to the GPS device SoftwareSerial ss(RXPin, TXPin); void setup() { Serial.begin(115200); ss.begin(GPSBaud); Serial.println(F("DeviceExample.ino")); Serial.println(F("A simple demonstration of TinyGPSPlus with an attached GPS module")); Serial.print(F("Testing TinyGPSPlus library v. ")); Serial.println(TinyGPSPlus::libraryVersion()); Serial.println(F("by Mikal Hart")); Serial.println(); } void loop() { // This sketch displays information every time a new sentence is correctly encoded. while (ss.available() > 0) if (gps.encode(ss.read())) displayInfo(); if (millis() > 5000 && gps.charsProcessed() < 10) { Serial.println(F("No GPS detected: check wiring.")); while(true); } } void displayInfo() { Serial.println(F("google map link below: ")); if (gps.location.isValid()) { Serial.print("http://www.google.com/maps/place/"); Serial.print(gps.location.lat(), 6); Serial.print(F(",")); Serial.println(gps.location.lng(), 6); } else { Serial.print(F("INVALID")); } Serial.print(F("Date/Time:")); if (gps.date.isValid()) { Serial.print(gps.date.month()); Serial.print(F("/")); Serial.print(gps.date.day()); Serial.print(F("/")); Serial.print(gps.date.year()); } else { Serial.print(F("INVALID")); } Serial.print(F(" ")); if (gps.time.isValid()) { if (gps.time.hour() < 10) Serial.print(F("0")); Serial.print(gps.time.hour()); Serial.print(F(":")); if (gps.time.minute() < 10) Serial.print(F("0")); Serial.print(gps.time.minute()); Serial.print(F(":")); if (gps.time.second() < 10) Serial.print(F("0")); Serial.print(gps.time.second()); Serial.print(F(".")); if (gps.time.centisecond() < 10) Serial.print(F("0")); Serial.print(gps.time.centisecond()); } else { Serial.print(F("INVALID")); } Serial.println(); }
Arduino GPS Code Explanation
Here’s the Arduino NEO 6M GPS code explanation before you upload the code.
#include <TinyGPSPlus.h> #include <SoftwareSerial.h>
This section includes the necessary libraries: TinyGPSPlus for handling GPS data and SoftwareSerial for software-based serial communication.
static const int RXPin = 3, TXPin = 2; static const uint32_t GPSBaud = 9600;
Defines constant values for the RX and TX pins for communication with the GPS module and sets the baud rate for the GPS communication.
TinyGPSPlus gps; SoftwareSerial ss(RXPin, TXPin);
Initializes the TinyGPSPlus object named GPS to handle GPS data and the SoftwareSerial object named ss to communicate with the GPS module using the specified RX and TX pins.
void setup() { Serial.begin(115200); ss.begin(GPSBaud); Serial.println(F("DeviceExample.ino")); // Printing information for demonstration and version of TinyGPSPlus library // by Mikal Hart }
Configures the serial communication for the Arduino board and the GPS module. It initializes the serial communication with the computer at a baud rate of 115200 and sets up the SoftwareSerial object at the defined GPS baud rate. It also prints some information about the demonstration and the version of the TinyGPSPlus library.
void loop() { while (ss.available() > 0) { if (gps.encode(ss.read())) { displayInfo(); } } if (millis() > 5000 && gps.charsProcessed() < 10) { Serial.println(F("No GPS detected: check wiring.")); while(true); } }
The main loop continually checks for available data from the GPS module using the SoftwareSerial object ss. If there is incoming data, it is read and processed by the gps.encode() function. If data is successfully encoded, the displayInfo() function is called to show GPS information. Additionally, it includes a check for GPS detection within a certain time frame and alerts if the GPS is not detected.
void displayInfo() { // Displaying Google Maps link with latitude and longitude information if GPS location is valid if (gps.location.isValid()) { Serial.print("http://www.google.com/maps/place/"); Serial.print(gps.location.lat(), 6); Serial.print(F(",")); Serial.println(gps.location.lng(), 6); } else { Serial.print(F("INVALID")); } // Displaying date and time information if valid if (gps.date.isValid()) { // Displaying date in MM/DD/YYYY format // If the date is invalid, it prints "INVALID" } else { Serial.print(F("INVALID")); } // Displaying time in HH:MM:SS format if valid // If the time is invalid, it prints "INVALID" }
The displayInfo() function prints GPS location information in the form of a Google Maps link (if the location is valid) containing latitude and longitude coordinates. It also displays date and time information if available in the specified formats.
Upload Arduino GPS Code to Arduino Nano
First Plug your Arduino Nano into your computer using a USB cable. Now copy and paste the program code provided above. Then Go to Tools > Board
and select “Arduino Nano“. Choose Processor (if necessary): Depending on your Nano version, you might need to select the Processor (e.g., “ATmega328P” or “ATmega328P (Old Bootloader)“). You can find this option in Tools > Processor
.
Now, Navigate to Tools > Port
and choose the right com port for Arduino Nano. Click on the checkmark icon in the top left corner of the IDE to compile and verify the code for errors.
Once compiled without errors, click on the right-arrow icon beside the checkmark to upload the code to the Arduino Nano. Wait for the IDE to show a message that the upload is done, confirming the program is successfully uploaded.
Arduino NEO 6M GPS Module – Working Demo
Once you successfully upload the program code to your Arduino board. You might notice different outputs on the Arduino NEO 6M GPS module. Here’s what the LED indicators mean after the device gets power.
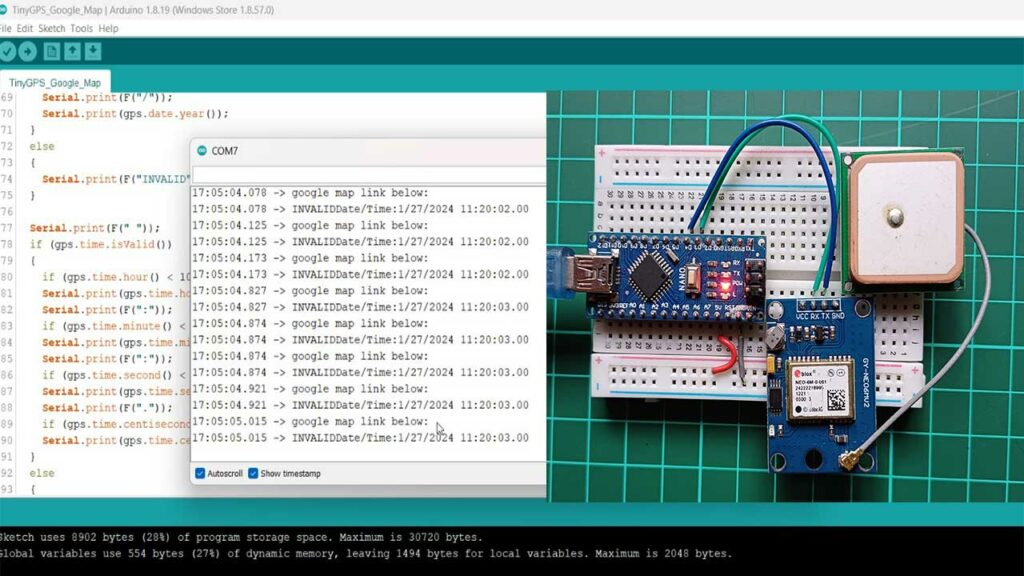
If the LED light on the Neo 6M GPS module is not blinking then try placing the device in an open area where it can receive satellite signals.
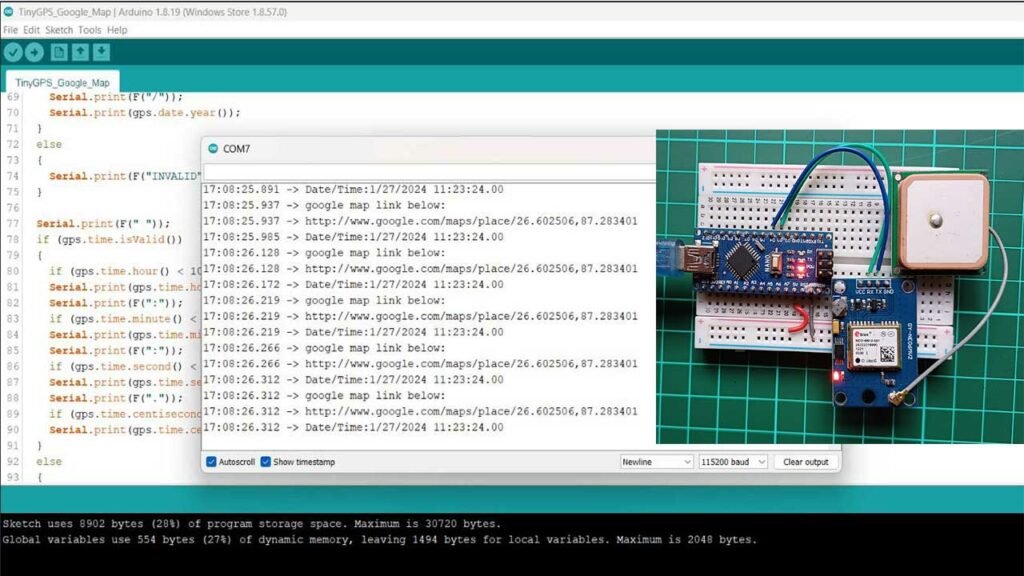
The Position Fix LED Indicator flashing once each second tells us that it has connected to enough satellites to provide our location details, including latitude and longitude.
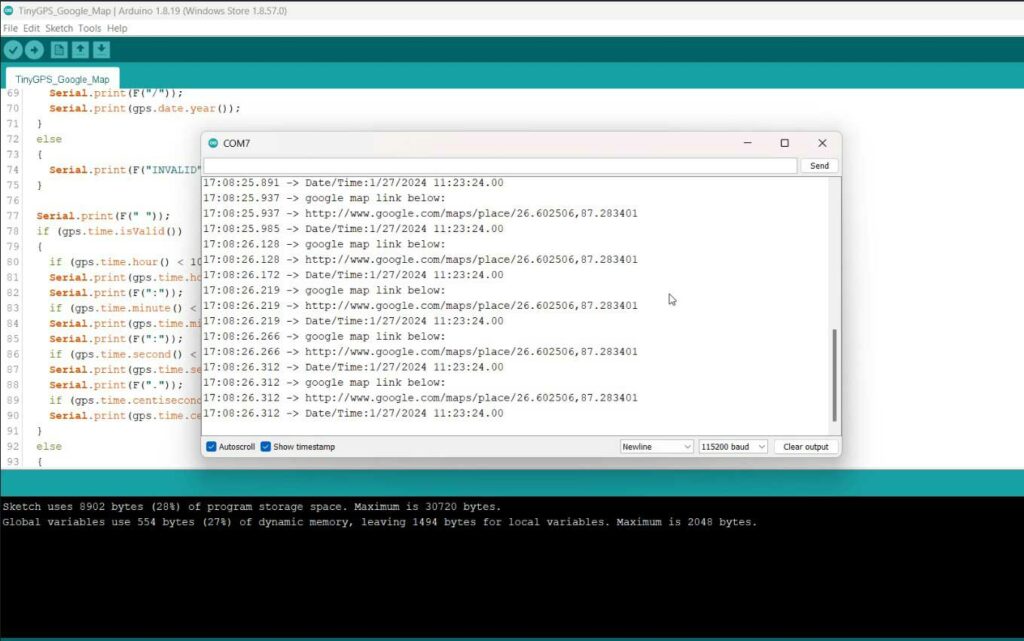
After the GPS gets a fix it will look like this, and the data will be displayed on the serial monitor
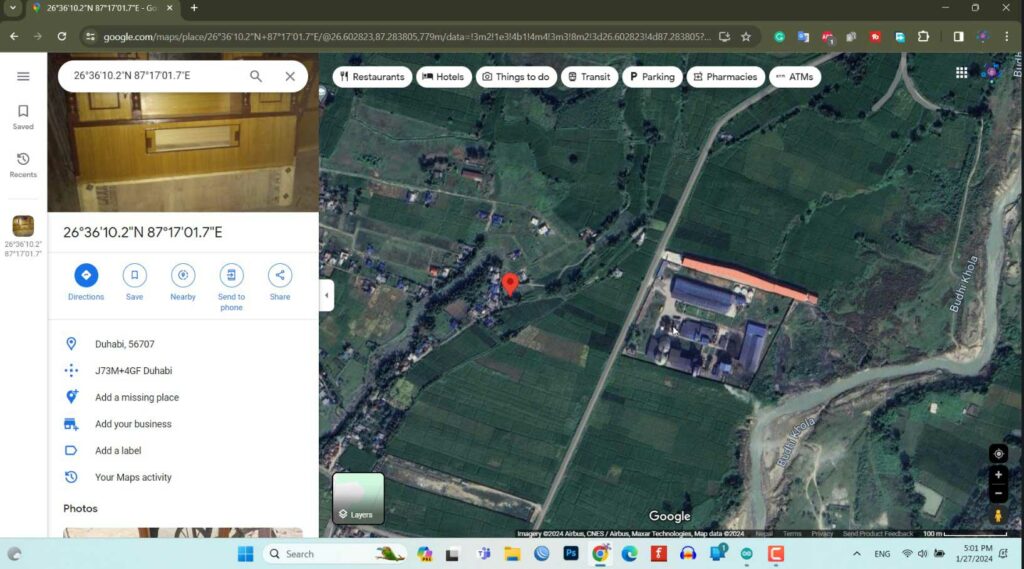
Now you can copy and paste the Google Map URL from the serial monitor and paste it in any web browser to see your real-time location.
NEO 6M GPS Module Not Working?
Getting the GPS Module Ready: If this is the first time you’re using the GPS module, you need to let it power up for a few hours before it’s ready to use. This process charges up internal parts of the module like the EEPROM and the clock. You’ll need a 3.7V Lithium Ion battery for this. To connect the battery, attach the plus side (often red) to the VCC pin on the module and the minus side (often black) to the GND pin. Once you’ve done this, leave the module alone to start up. When the LED light on the module starts to blink, it means it’s working. It might take around 2 hours for the LED to start blinking the first time you do this.
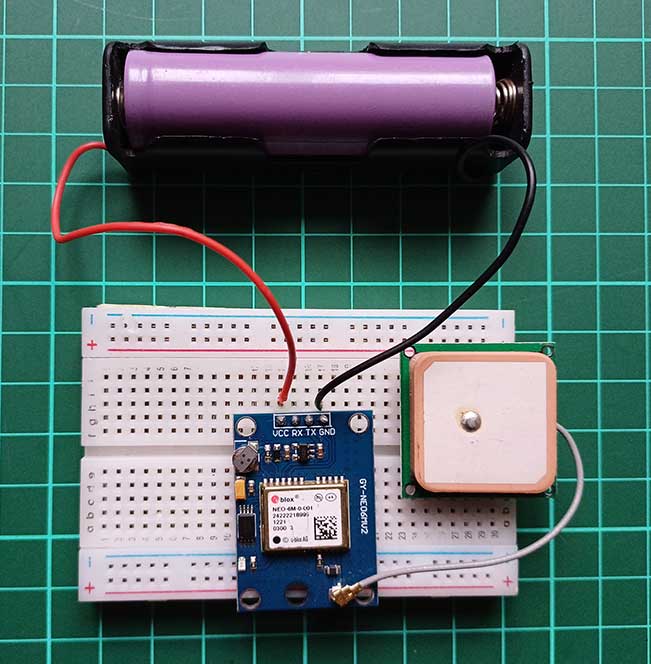
Best Place to Use GPS: Try to be outside or close to a window where there are not many things in the way. This helps your GPS work better and gives you more precise locations.
Check Your Cable Connections: Make sure that the pins marked as RX (for receiving signals) and TX (for sending signals) are plugged in the right spots. If it’s still not working, try swapping them around in case they were connected the wrong way. This could help get them to communicate properly.