Interface LDR Photo Resistor to Arduino and Control LEDs
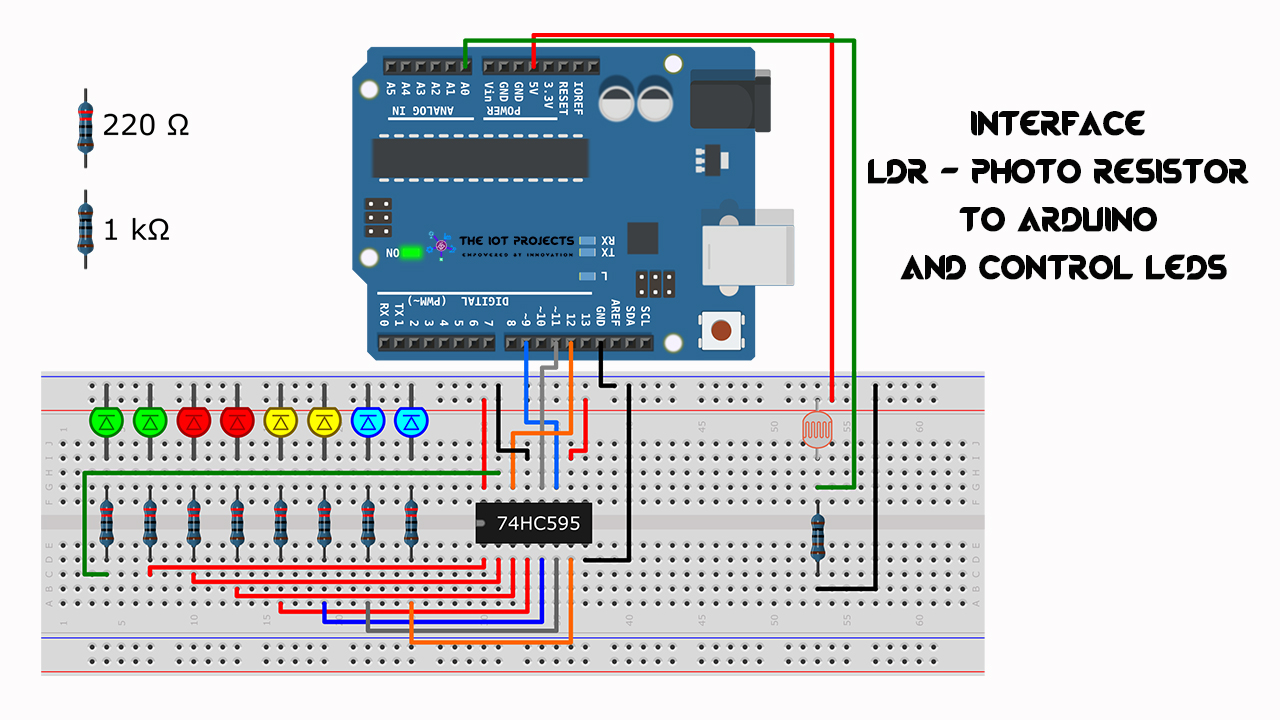
Hello, everyone in this LDR Tutorial we are going to Interface LDR Photo Resistor to Arduino and Control LEDs. Firstly, We will learn to Interface 74HC595 IC and 8 LEDs to the Arduino. Secondly, we will program the Arduino board to control the light using Analog input LDR i.e. Light Dependent Resistor. Finally, we will test the whole system.
Components Required for this project
- Arduino UNO
- LDR Photo Resistor
- Breadboard
- 1 -1k-ohm resistor
- 8 – 220- ohm resistors
- 8 – LEDs
- 74HC595 IC
- Jumper Wires
Working Process of LDR Sensor
As I have already mentioned, In this tutorial we are going to learn How to measure Light Intensity using an analog input (LDR) Light Dependent Resistor. As you can see in the image table below. The value of resistance decreases as the light intensity increases.
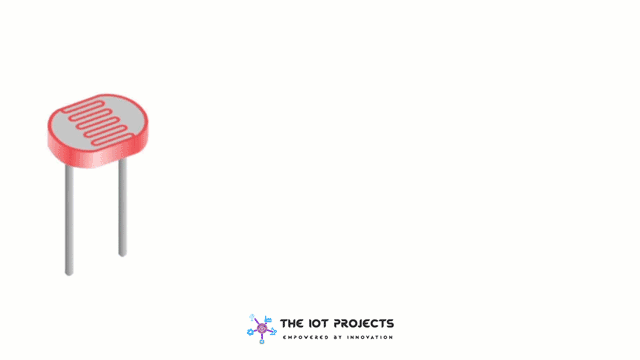
Lab Work with LDR
Actually, we are going to set up a voltage divider with the LDR and 1K-ohm resistor. We will connect the midpoint of the voltage divider to the analog input of Arduino.
As you can see in the below image that the voltage increasing over the light intensity.
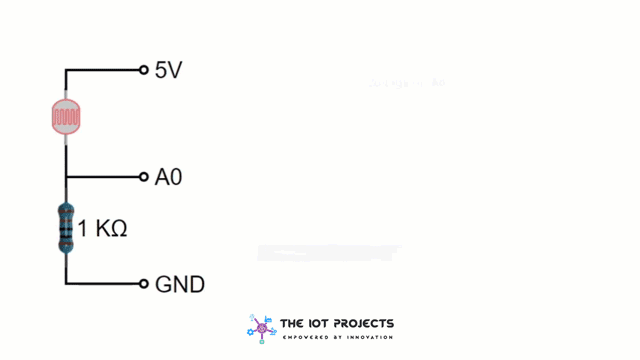
74HC595 IC Pinouts
- The 74HC595 IC has 16 pins in which power supply pins are number 8 (GND) and 16 (VCC). We will use 5 volts for VCC.
- Pin no. 10 is Master Reset, We have to keep this pin to high level to ensure proper operation of IC.
- Pin no 14 is DS, Data Serial Input. We simply call it Data Pin.
- Pin no. 11 is SHCP, that is known as Clock pin.
- Pin 12 is STCP known as Latch pin.
- Pin no. 13 is OE, Output Enable. It is used to enable the output. So we will set this pin always low (Always Active)
- Pin Q0 to Q7 are the outputs pins. We will connect them to LEDs.
- Finally, Pin Q7 is used when you want to connect multiple 595IC in a cascade.
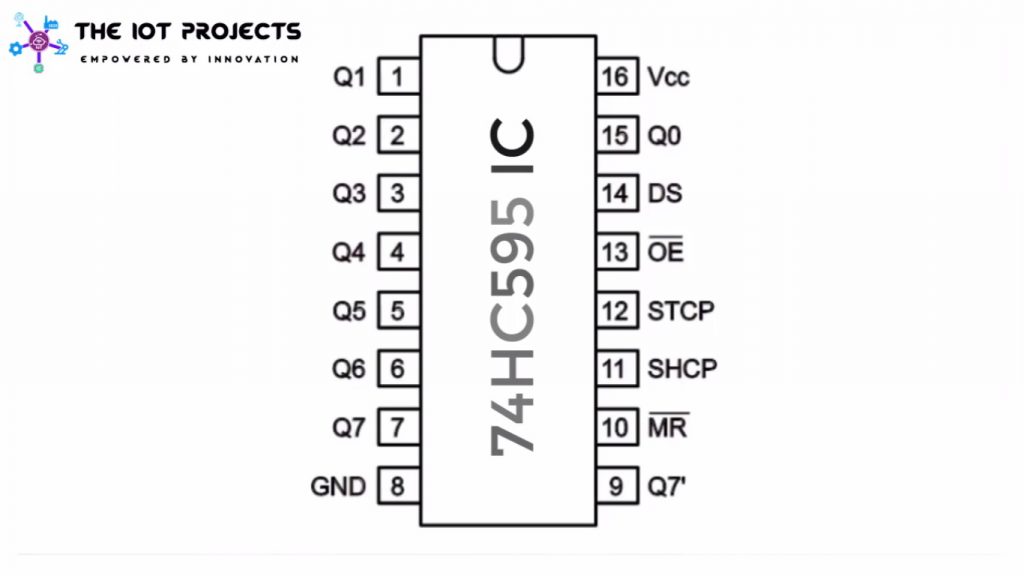
Learn more about Arduino UNO Pinout from this article
Internal overview of 74HC595 IC
Internally, the 595IC contains three Registers. The upper one is called “Shift Register“. It receives the data through the DS pin. On every rising edge of the SHCP(Clock) signal the register will shift to the left.
The Middle Register is used to store the values of the Shift Register. To copy the Shift Register to the Storage Register we need a rising edge on the STCP (i.e LATCH) Pin.
The Lower Register is the actual values of the Output. This will be either High Impedance if Output Enable (OE) is high or the value of the storage Register If Output Enable is Low.
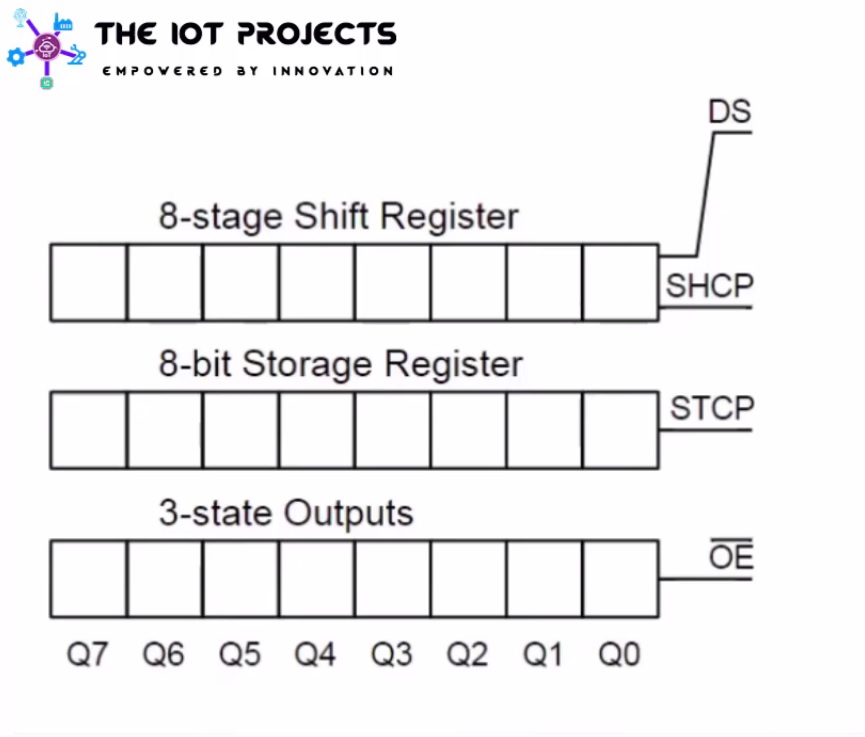
At every rising edge at the SHCP(clock) in the shift register is shifted by the level of the DS Pin. Basically, after 8 rising edges on the clock signal the shift register is full of Data. with a rising edge of the latch signal (STCP) pin the values of the shift register are copied on to the storage register. But the outputs are no updated and they are still in High Impedance because the pin Output Enable is High.
The output Enable Signal becomes low and the outputs reflect the values of the storage register. A second byte has entered the shift register. At the next rising edge of the latch signal (STCP), the storage Register is copied with the value of the shift register. And the outputs are updated with the new value because the Output Enable pin is low.
Interfacing 74HC595 IC, LEDs and LDR to Arduino
Let’s begin with the connections of 74HC595 IC and LEDs.
Connect GND and 5v pin of the Arduino to the upper horizontal rows of the breadboard.
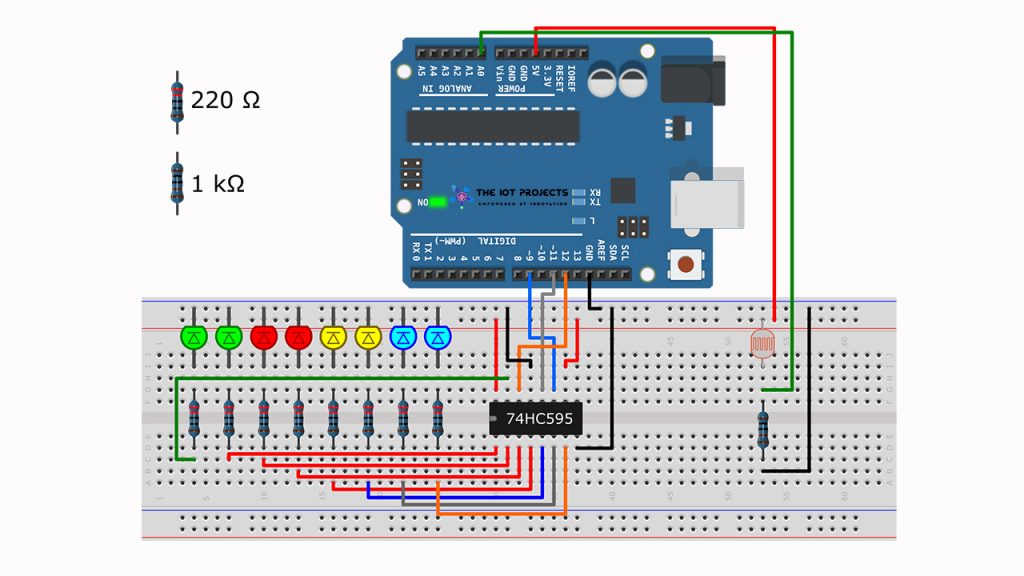
Connection of 74HC595 IC pins:
- Power Pins 8 – GND and 16 – 5 volts.
- Master Pin- 10 to the 5 volts. To have it always enabled.
- Pin 13 (Output Enable) to GND to have outputs always active.
- Arduino pin 12 to Data Pin (14) of IC
- Connect Arduino Pin 9 to Latch pin (12)
- Q0 to Q7 are outputs pin. Connect them to the LEDs through 220-ohm resistors. Note that the pin 9 (Q7) of the iC is not connected.
- Connect one end of the LDR to 5 volts.
- Now Connect another end of the LDR to 1k resistor.
- Other ends of the resistor to the GND.
- Mid pint of the voltage divider to the analog input pin A0 of the Arduino.
Sketch/Program codes
The variable reading will hold the analog value of the voltage divider formed by the LDR and 1k resistor.
int reading = analogRead(lightPin);
The variable “numLEDSLit” is the number of LEDs that we have to switch on: The calculations are explained as follows: The maximum value of the analog input is 1023. As we have 8 LEDs. we divide it by 9, and also by 2 because the divider will show the half to the value. When the value of the LDR resistance is 1k.
int numLEDSLit = reading / 57; //1023 / 9 / 2
With the if Statement you limit the number of LEDs to light to 8. leds=0 means no LEDs to start
if (numLEDSLit > 8) numLEDSLit = 8;
leds = 0; // no LEDs light to start
Similarly, with the for loop, you add so many 1 as LED to turn “ON”
for (int i = 0; i < numLEDSLit; i++)
{
leds = leds + (1 << i); // sets the i'th bit
}
Finally, the 74HC595 IC is updated with the values of the variable LEDs.
updateShiftRegister();
Final Sketch/Program
int lightPin = 0;
int latchPin = 11;
int clockPin = 9;
int dataPin = 12;
int leds = 0;
void setup()
{
pinMode(latchPin, OUTPUT);
pinMode(dataPin, OUTPUT);
pinMode(clockPin, OUTPUT);
}
void updateShiftRegister()
{
digitalWrite(latchPin, LOW);
shiftOut(dataPin, clockPin, LSBFIRST, leds);
digitalWrite(latchPin, HIGH);
}
void loop()
{
int reading = analogRead(lightPin);
int numLEDSLit = reading / 57; //1023 / 9 / 2
if (numLEDSLit > 8) numLEDSLit = 8;
leds = 0; // no LEDs light to start
for (int i = 0; i < numLEDSLit; i++)
{
leds = leds + (1 << i); // sets the i'th bit
}
updateShiftRegister();
}
Video Tutorials
Conclusion
Finally, we have completed the Interface LDR Photo Resistor to Arduino and Control LEDs projects. Now, you can control the 8 LEDs using the LDR Sensor Interfaced with Arduino Board. We hope you found this project useful! Drop a comment below if you have any doubts or queries. We’ll do our best to answer your questions.