What is MQTT and why do we need it?
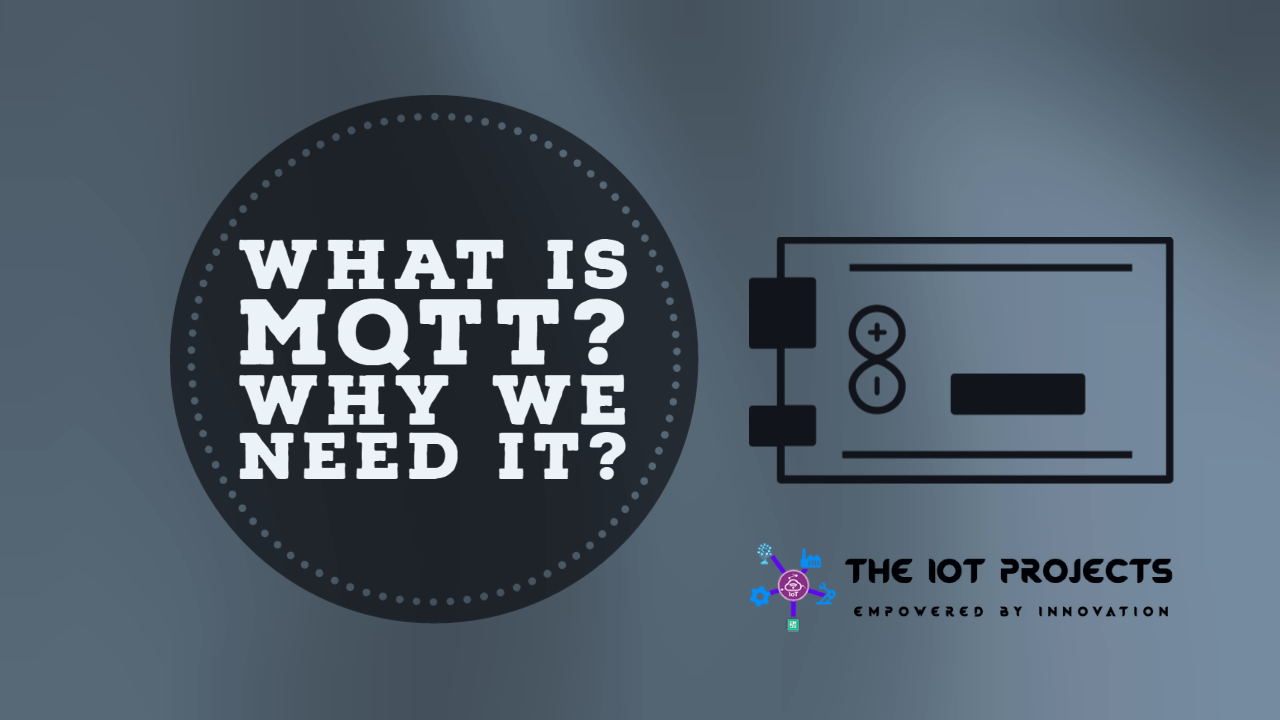
MQTT is an OASIS standard messaging protocol for the Internet of Things (IoT). it’s a light-weight messaging protocol for tiny sensors and mobile devices. Optimized for high latency or unreliable networks, enabling smarter projects and devices.
Introduction to MQTT Protocol
MQTT is a symbol messaging protocol. it’s designed for constrained devices with low bandwidth. So, it is the perfect solution for Internet of Things applications. MQTT allows you to send commands to regulate outputs, read unpublished data from the sensor node, and far more. Therefore, it makes it very easy to establish communication between multiple devices.
Note: If you prefer home automation and need to create an entire home automation system then you’ll be able to easily do this using MQTT.
Commands/Concepts of MQTT
Now so as to use MQTT, you need to understand the main commands and their use.
- Publish/Subscribe
- Messages
- Topics
- Broker
Publish/Subscribe Command
Now, let’s start with the main concept of the Publish and subscribe system. In publish and subscribe a device can publish a message on a subject or it can subscribe to a specific topic to receive messages. So, it can send a temperature sensor value to a particular topic online.
Messages Command
While the messages, the second concept is the data that you simply want to exchange between your devices, whether it’s a command or data.
MQTT Topics Command
The third concept here is that the Topics. It’s another important concept. Topics are the way you simply interest in incoming messages or how you specify where you would like to publish the message. Topics are presented with a string submitted by forward-slash(/). Each forward-slash(/) indicates a topic level.
Here you’ll take this as an example:
If we added (MCU/) MCU with forward-slash, it means we’ve added MCU as a topic. If we added ESP (After forwarding slash), then we are specifying another topic inside MCU. => MCU/ESP.
So, MCU is the main topic and ESP is the subtopic and that we can keep going like that.
MQTT Broker Command
Now, the fourth concept is a Broker. you furthermore might need to be aware that the broker is primarily liable for receiving all messages, filtering messages, deciding who is inquisitive about it, then publish the messages to all or any subscribed clients. So, it’s a very important concept, when it involves multi-level and multi-devices systems.
Note: we mainly use the publish concept to publish the information to MQTT online server from the ESP Board and ESP will receive those data from the microcontrollers in most of the IoT based projects.
Wrapping up
So, this is the main concept and this is what’s MQTT and why it is important?. you can also replace the MQTT with other APIs like Google Firebase. But, you need some coding concepts to adjust the code.
Recommended Readings:
- Introduction to The Internet Of Things (IoT)
- How 555 IC works in Astable Operation & Monostable Operation
- Everything about PIC Microcontroller
- The Internet of Things: Needs, Benefits and Features
- Top 10 Coolest IoT Devices of 2020
- IoT based Fire Detector & Automatic Extinguisher using NodeMCU
- The History of Printed Circuit Board PCB
- Monitor MPU6050 Tilt Angle on Blynk using NodeMCU
- IoT vs IIoT and its protocols