Getting started with DWIN HMI Display
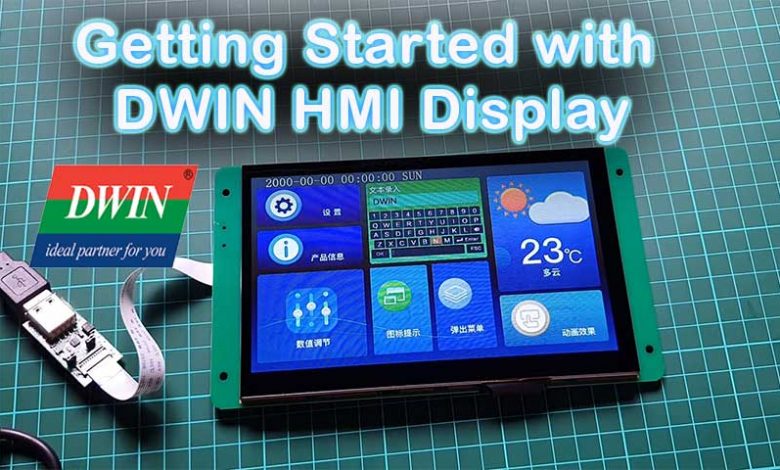
Today’s Tutorial is on DWIN HMI Touch Screen Display. HMI Means Human-Machine Interface. DWIN is specialized in making HMI Touch screen displays that are compatible with all microcontrollers like Arduino, STM32, PIC, and 8051 families of Microcontrollers. This is a Getting started tutorial with DWIN HMI Display. So, we will cover everything about this display in this session.
Overview: DWIN HMI (DMG80480C070_04WTC) Display
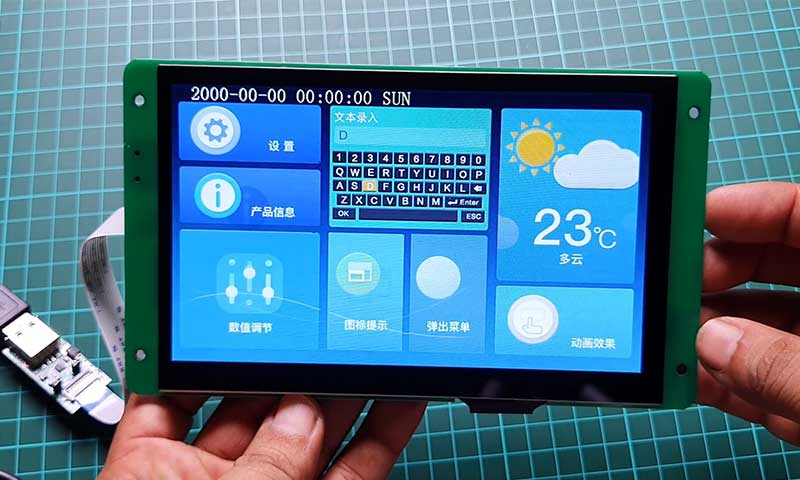
Recently I got this Smart Touch screen TFT-Display from the DWIN. The model of the display that I got is DMG80480C070_04WTC.
- The First two letters DM mean DWIN Smart LCM Product Line.
- G means (18-bit/24-bit) 262K colors.
- 80480 means 800 by 480-pixel resolution.
- C means Commercial grade display.
- 070 means a 7.0-inch Display size.
- 04 means a Basic type of screen.
- W stands for Wide Temperature.
- TC Stands for Capacitive touchpanel.
The operating Voltage is 4.5 to 5.5 Volt DC.
Order Your DWIN HIM Display
You can order any LCD Screen of your choice from https://www.aliexpress.com/
You can use this coupon DWINFERDINAN to get a discount
Hardware Interface of DMG80480C070_04WTC Display
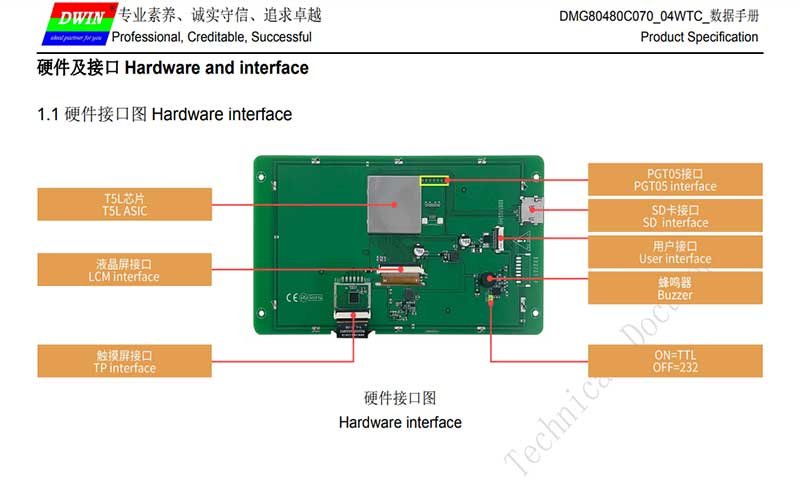
Here you can see different hardware interfaces of this product.
- T5L0 Interface
- LCM Interface
- TP Interface
- PGT05 Interface
- SD Card Interface
- User Interface
- Buzzer
- ON=TTL, OFF=232 Interface
Here you can see the SD Card Interface a buzzer and a User Interface to connect the FCC-10 terminal block.
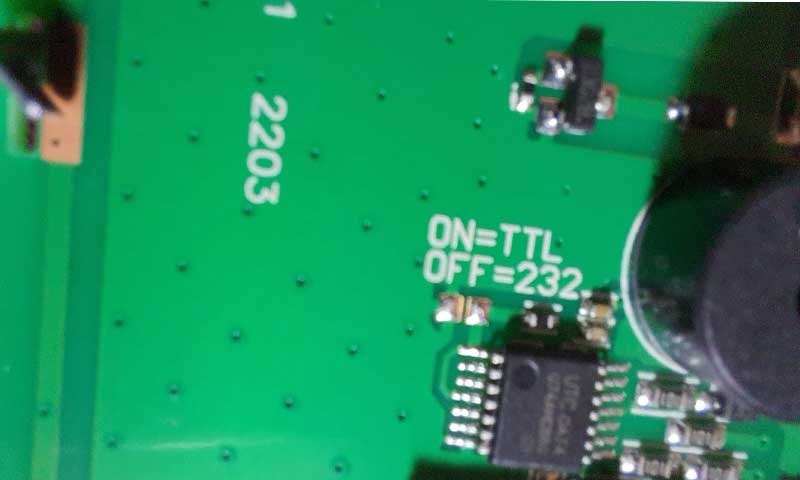
You can change the TTL Interface mode or RS232 mode from here. Just solder these two terminals as shown here to enable TTL Interface. By default, the module is in RS232 Interface.
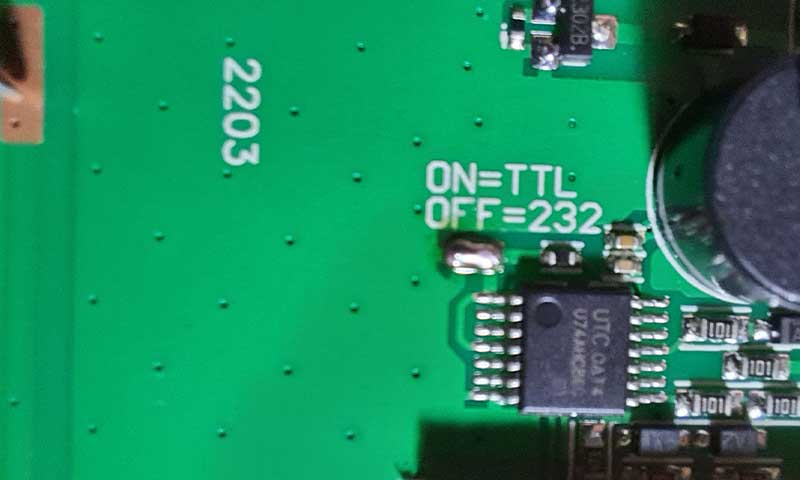
HDL662B adaptor with FCC Cable and Male to Male USB Cable
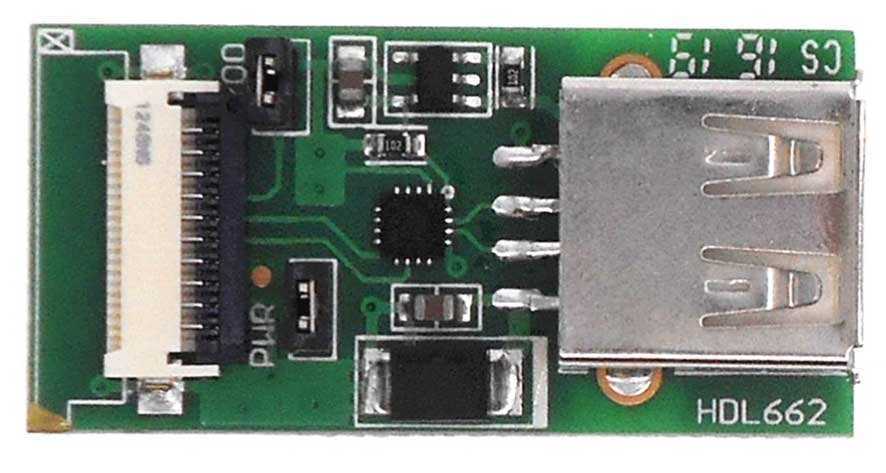
Here we are provided with an HDL662B adaptor board. This board is nothing but a USB to TTL board. Then we have here FCC cable which is supplied with this HDL board. On the LCD board, you can see the flip-open connector. Just flip open the connector and insert the FCC cable. Keep in mind that the blue ends should be on top. Now you can just press the lock so the FCC cable is locked.
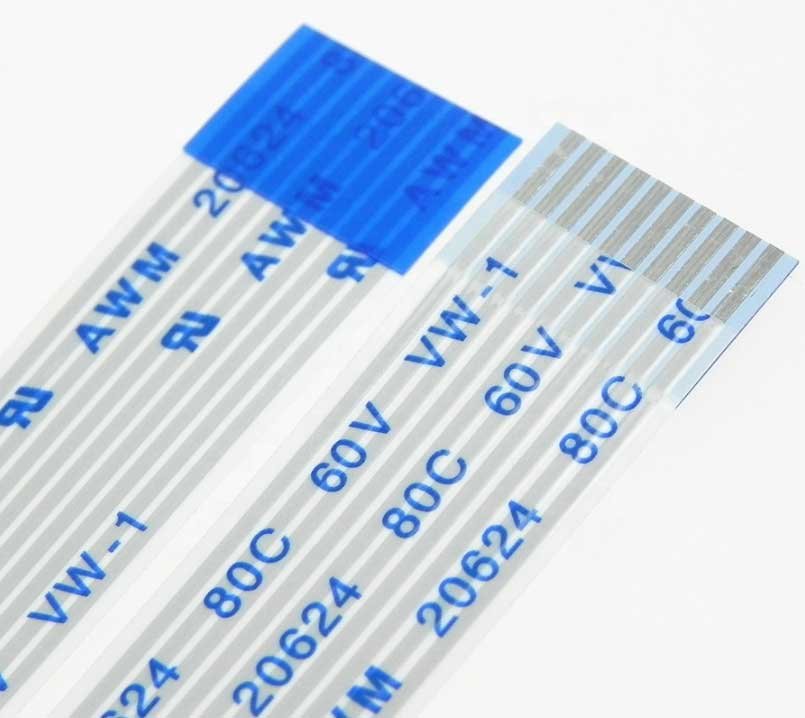
In the same way, another end of the FCC cable is connected to the HDL by keeping the blue end on the top.
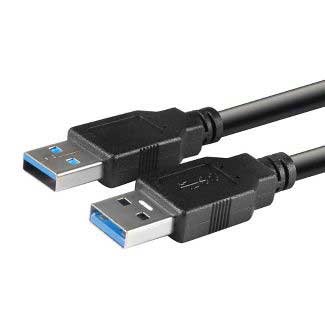
Now here we have a male-to-male USB cable. So, one end is connected to this HDL board and another END is connected to your PC.
Format SD Card
Here we can use an SD Card of up to 16GB to download the firmware files. Above 16 GB is not supported. We can easily insert the Micro SD card into its sd card slot.
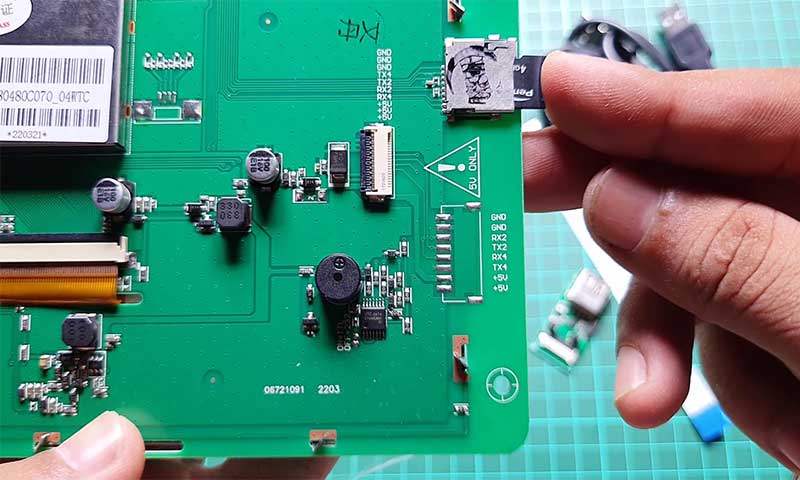
But we need to format this SD card in a FAT32 file system. You can follow this instruction to format the SD Card.
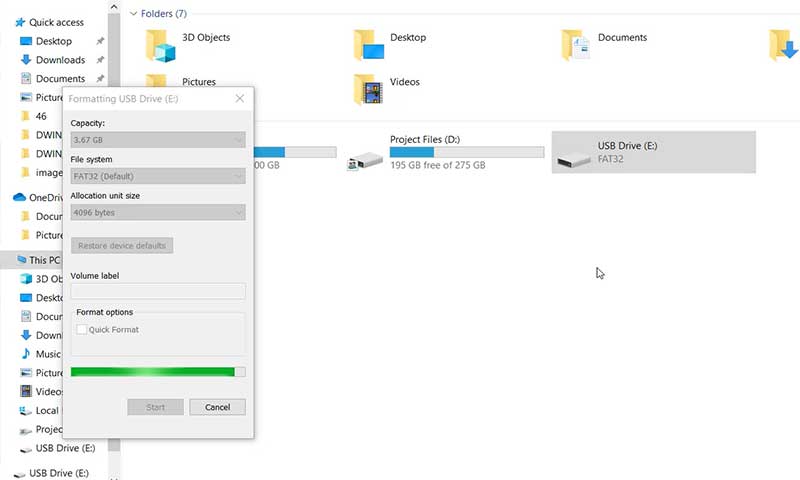
DGUS Software & HDL662B Driver
Now, let us visit the official website of dwin-global.com. You can see this DWIN company making a wide range of High-quality Touch screens for Android LCD, HDMI LCD, and Linux LCD Displays.
Under the Download, section Go to tools and Download the DGUS-DWIN Graphic utilized software i.e DGUS software and XR21X Driver. This driver is for HDL662B board. So, download and extract both files.
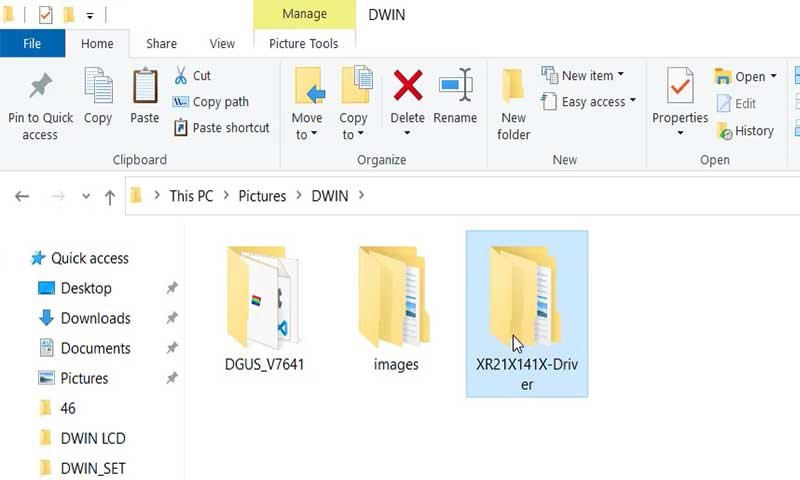
We don’t need to install this DGUS software. Under this DGUS folder, you can see some executables files. Which includes tools like the ICL tool, font generating tool, image resizing tool, etc. inside this DGUS Software.
Inside the XR21X folder, there are driver files. You need to install the driver on your PC to establish the communication between HDL662B and your PC. Double-click the executable file to launch the driver installer. Now click on Install to install the driver on your Windows PC.
Download Project UI on DWIN Display
I have created my own UI using this DGUS Software. I will cover the detailed video on How to create your own UI for DWIN Display using DGUS software In the upcoming episode.
Download through SD Card Interface
Now, let’s flash this UI on this 7-inch DWIN Display. You need to Copy the DWIN underscore SET Folder onto your SD Card then Insert the SD card on LCD Display. Now connect the USB cable connected to the HDL board.
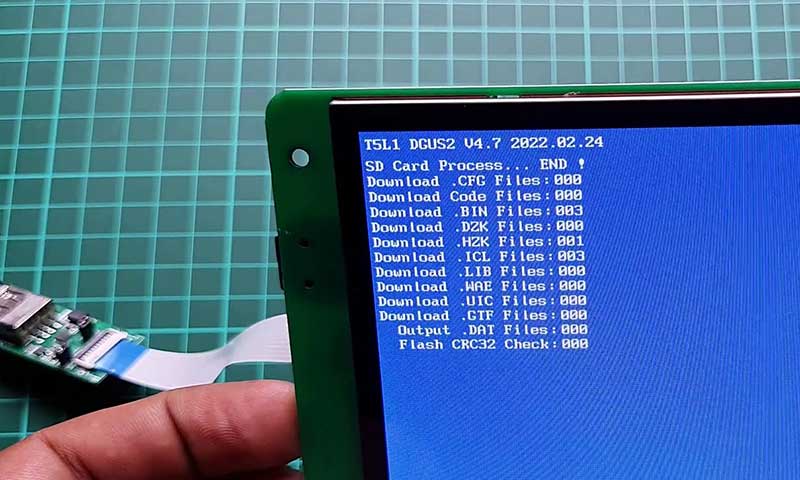
You can see the downloading process started immediately after powering the LCD. After completing the download process remove the USB from the PC then remove the SD Card from the Display. Now again connect the USB Cable. Now you can see our newly flash UI on LCD Display.
Flash through TL5 Download tool
Alternatively, if you don’t have an SD Card you can use the T5L Tool in DGUS Software to flash the UI onto your LCD Display.
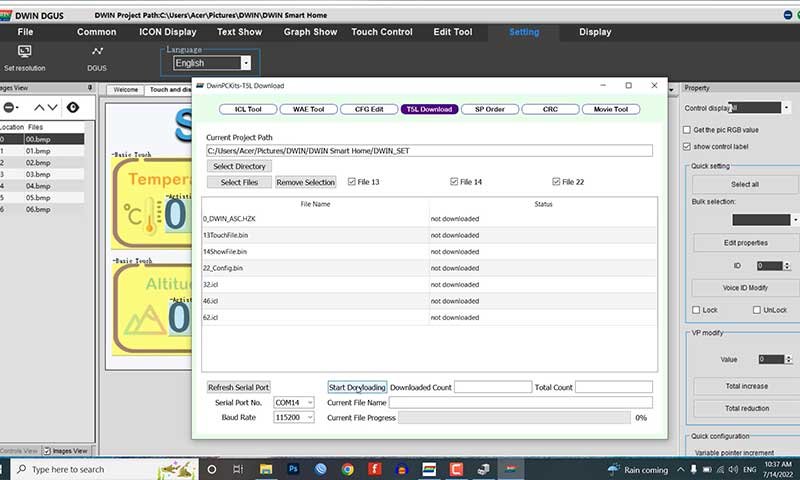
Here, select all these files which are available in the DWIN_SET folder as shown here. After that, select the COM port of the HDL board. You can see the COM port from Device Manager on Windows. Now you can click on Start downloading button.
Our Projects with DWIN HMI (DMG80480C070_04WTC) Display
This is the output of our project which we have designed using DGUS Software.
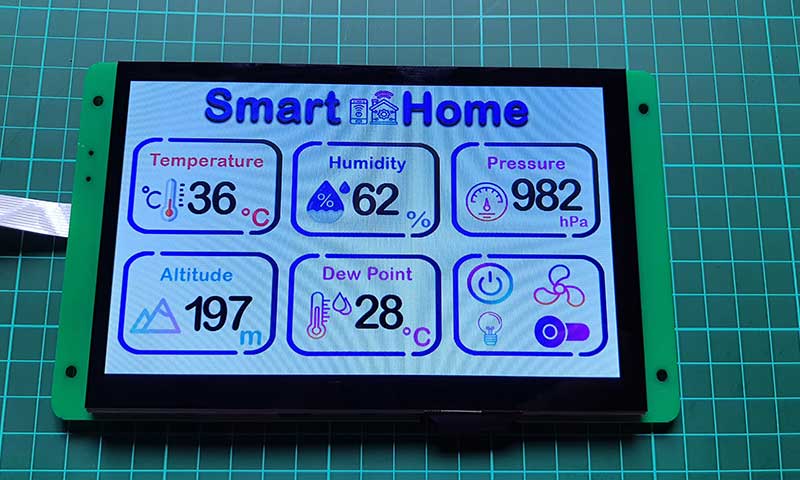
In our next episode, we will teach you to design and configure this UI. And Interface the sensors and relays to the Arduino board.
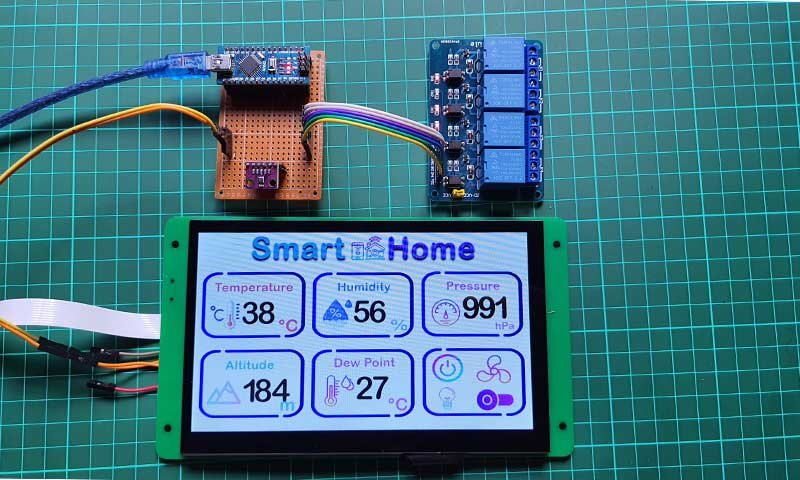
Then we will make a serial communication between Arduino and LCD Display to monitor the sensor data and control our AC Home appliances like Light, Fan, AC, TV, etc. using this LCD Touch Display.
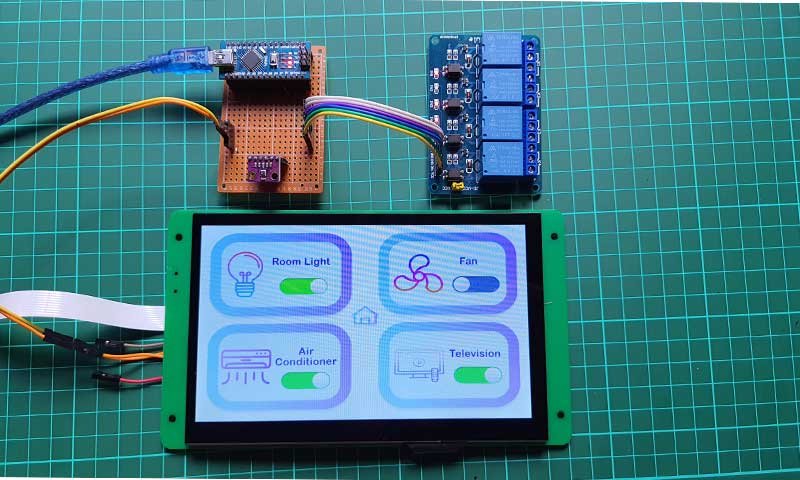
Video Tutorial & Guide
This is the Getting started with DWIN HMI Display video tutorial. Hope you will like it. You can subscribe to our youtube channel so you will not miss any future updates from our channel.
Grazie per la tua generosità nel condividere le informazioni di questo articolo.