IoT Based LED Control using Google Firebase & ESP8266
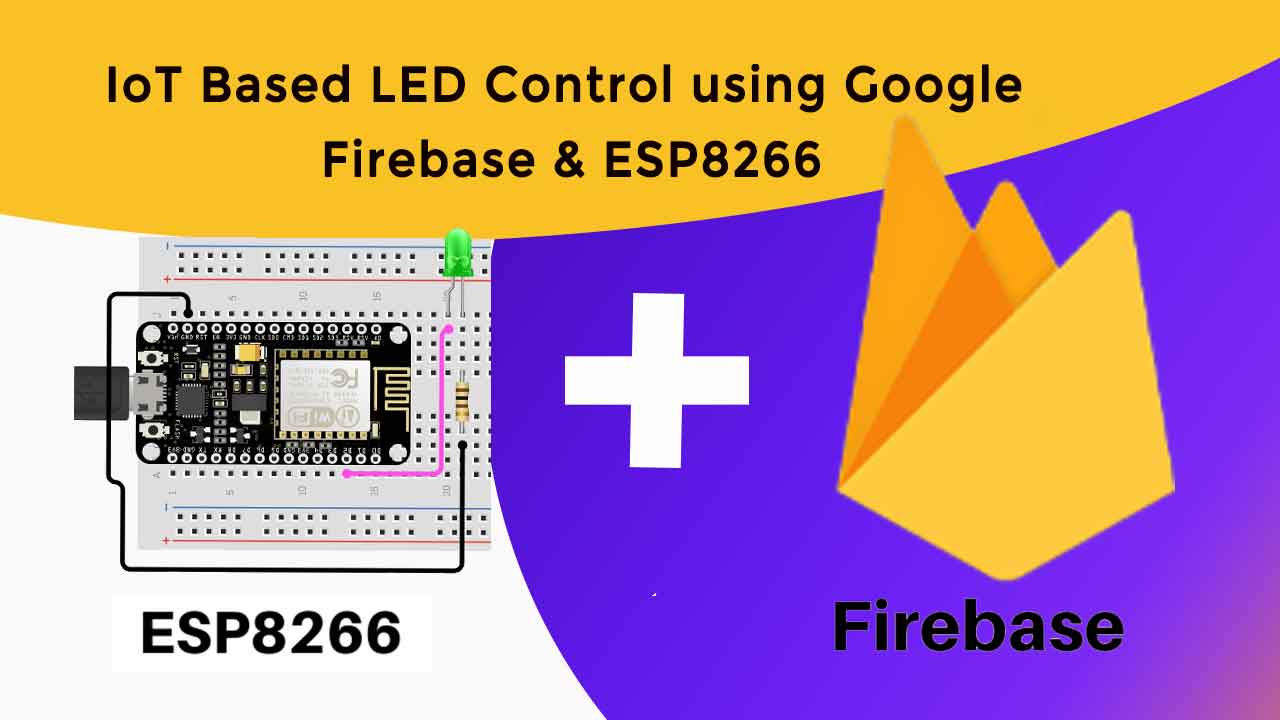
In this quick tutorial, we will learn to control LED using Google Firebase and ESP8266 (NodeMCU) development board. Simply integrating google firebase in our previous project, you can control your LED from anywhere in the world.
- Introduction: IoT Based LED Control using Google Firebase & ESP8266
- Components Required
- Set up Google Firebase -15 steps
- Circuit Diagram for IoT based LED Control
- Installing Required Library
- Control LED using Google Firebase & ESP8266
- Program Code: IoT Based LED Control using Google Firebase & ESP8266
- Code Explanation
- Wrapping Up
Introduction: IoT Based LED Control using Google Firebase & ESP8266
NodeMCU ESP8266 is a powerful hardware platform for IoT applications. It is widely used for the development and prototyping of IoT applications. While Google Firebase is Google’s database platform widely used for creating, managing, and modifying data generated from any Android/IOS application, web services, sensors, etc.
Internet of Things (IoT) based projects that you may like:
- IoT Based RFID Smart Door Lock System Using NodeMCU ESp8266
- RFID Based Attendance System Using NodeMCU with PHP Web App
- NodeMCU ESP8266 Monitoring DHT11/DHT22 Temperature and Humidity with Local Web Server
- Connect RFID to PHP & MySQL Database with NodeMcu ESP8266
Basically we have various methods of controlling LEDs such as using Web Server/ Webpage, Blynk IoT Platform, and using other API based services. But here we will only focus on Google Firebase to control LED using ESP8266.
To control LED using Google Firebase first we will setup Google Firebase console and then ESP8266 module.
Components Required
Following are the list of all the components that are required to complete this project. All the components are easily available.
S.N. | Components Name | Description | Quantity |
---|---|---|---|
1 | NodeMCU | ESP8266-12E Board | 1 |
2 | LED | 5mm LED of Any Color | 1 |
3 | Resistor | 220 ohm | 1 |
4 | Power Supply | 5V, 1A Power Adapter | 1 |
Set up Google Firebase -15 steps
To control LEDs using Google Firebase and Nodemcu ESP8266, you need to first set up Google Firebase. The step may be a bit long but do not panic, as I actually have described all in 15 steps and it is super easy.
Step 1: If you’ve got a Gmail account, then you’re ready for signing up for Firebase. But if you do not have a Gmail ID, register for Gmail first: https://gmail.com/
Step 2: Go to the link: https://firebase.google.com/ and click on on go to console within the top right corner.
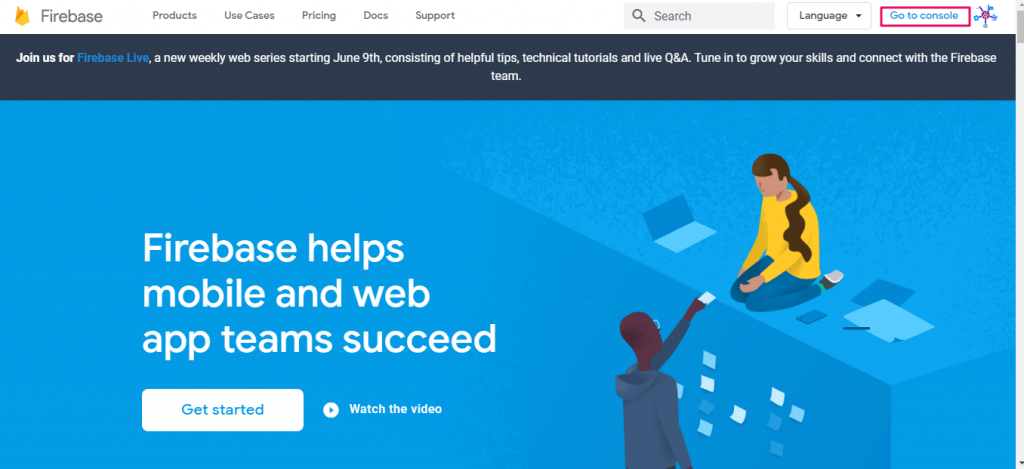
Step 3: Again Click on “Create Project“.
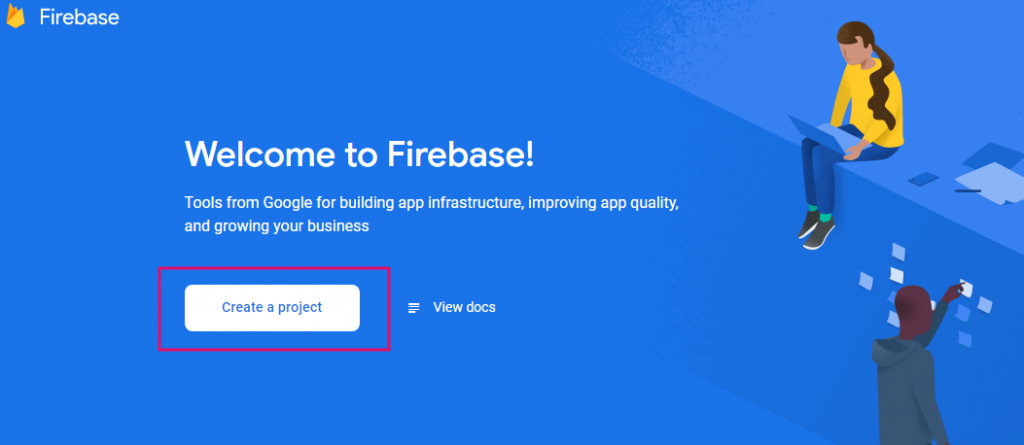
Step 4: Enter your “Project Name“, then click “I accept Firebase Terms” and finally click “Continue“.
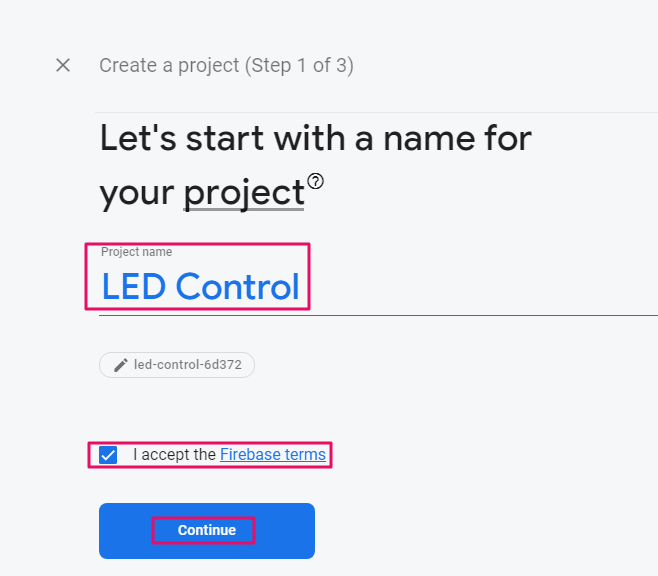
Step 5: The next window will appear. So click “Continue“.
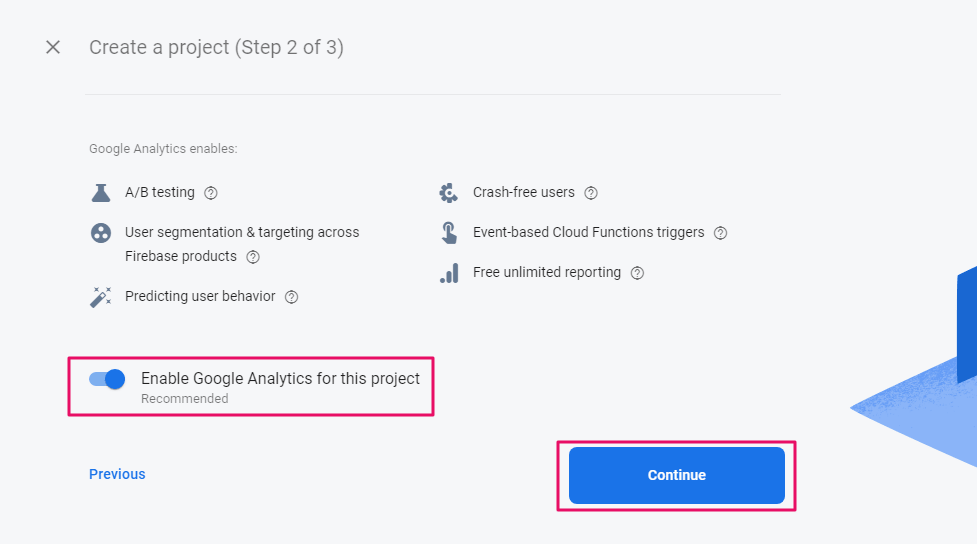
Step 6: Select “Google Analytics Account” created using Gmail ID. Then click “Create Project“.
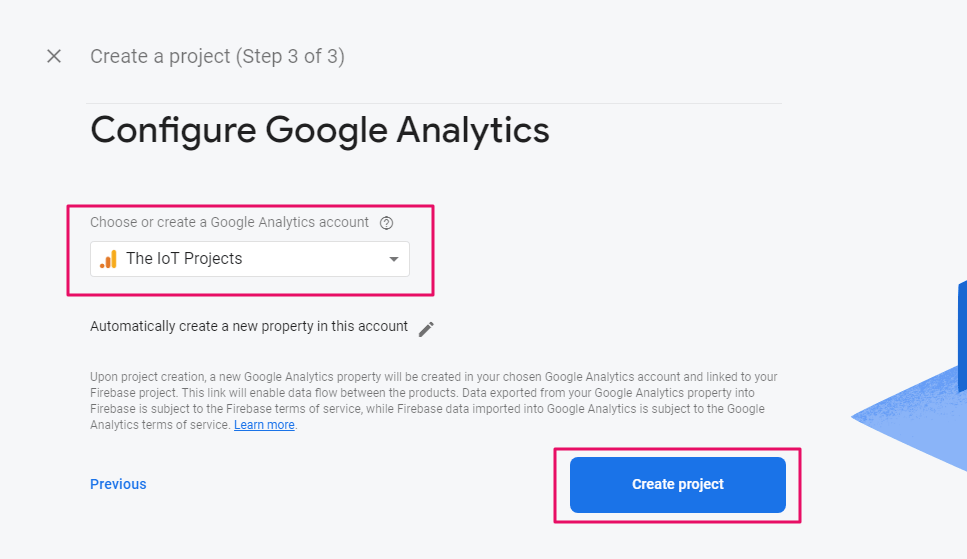
Step 7: Your project is now ready. Then you will get the following window. Click “Continue“.
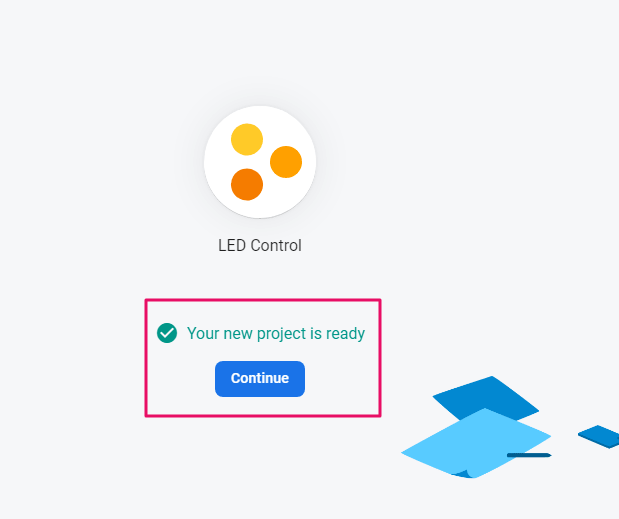
Step 8: Simply click on “Project Settings“.
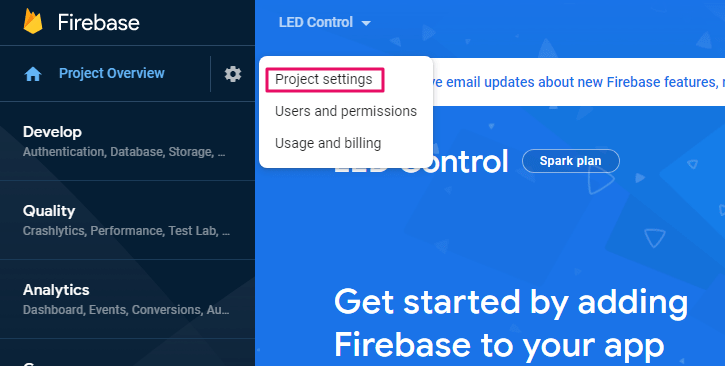
Step 9: Under Project Settings, click on “Service Account“. Copy the secret key below. Ordino code requires code.
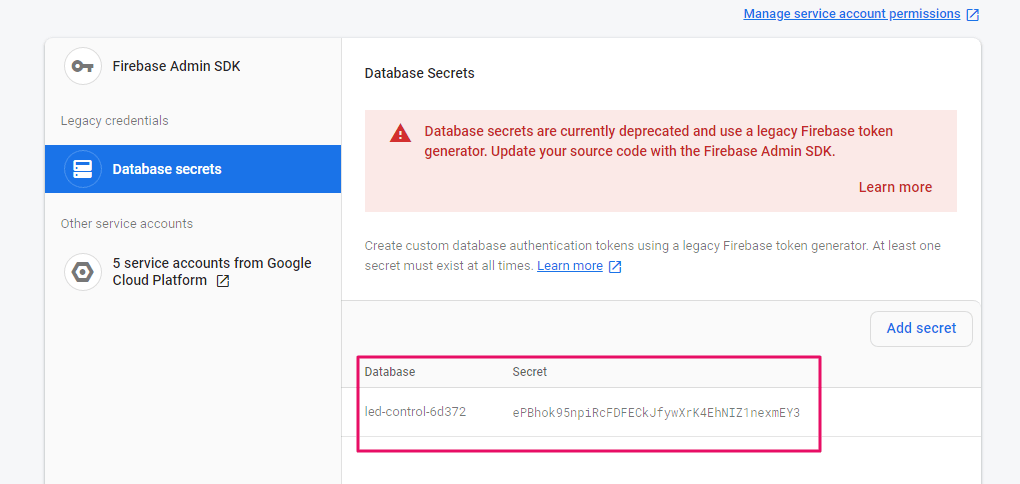
Step 10: Here, Click on “Create Database“.
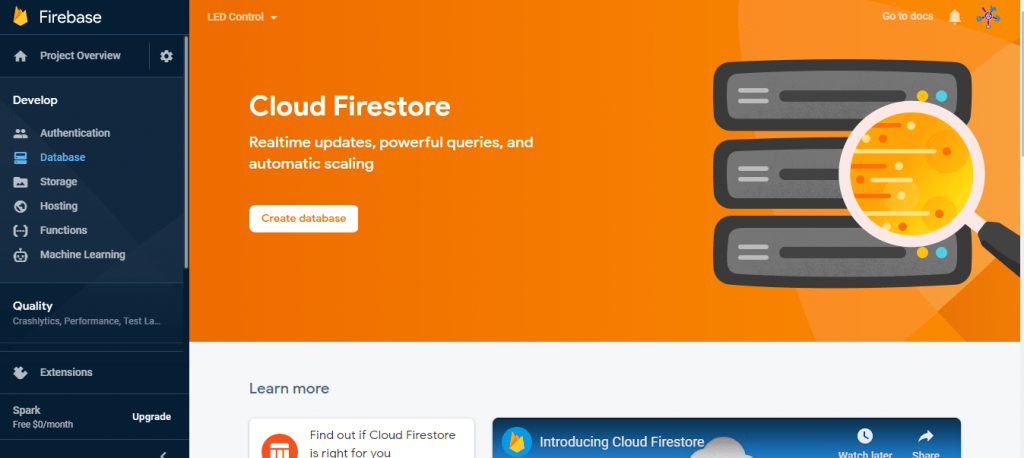
Step 11: Select “Start in Test Mode” and then click on “Next“.
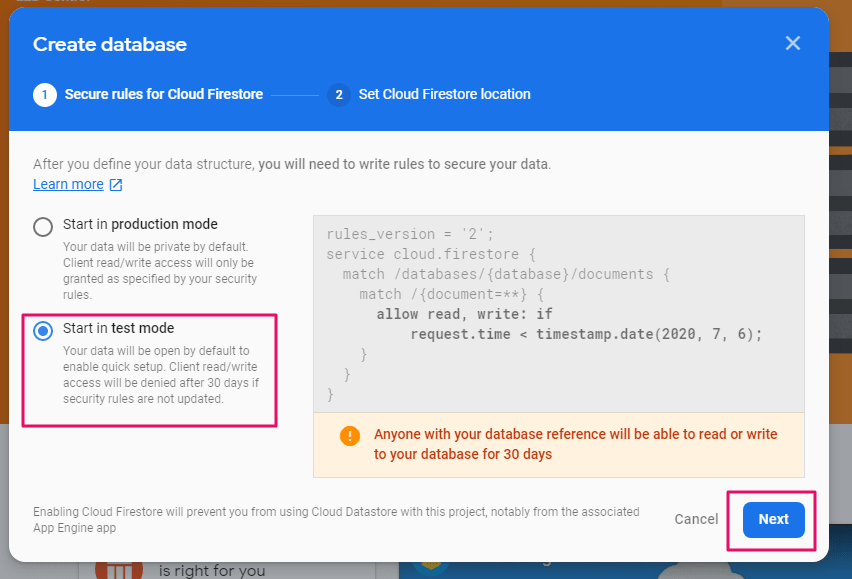
Step 12: Finally, click on “Done” and left-click on “Database”.
Step 13: Select the “Realtime Database” option from the Database list.
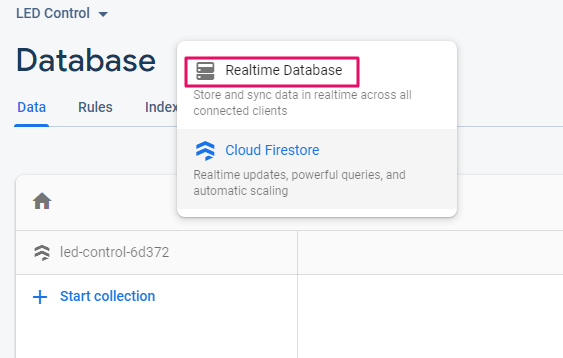
Step 14: Now your final project is ready. You can modify LED status “On/Off” from the list.
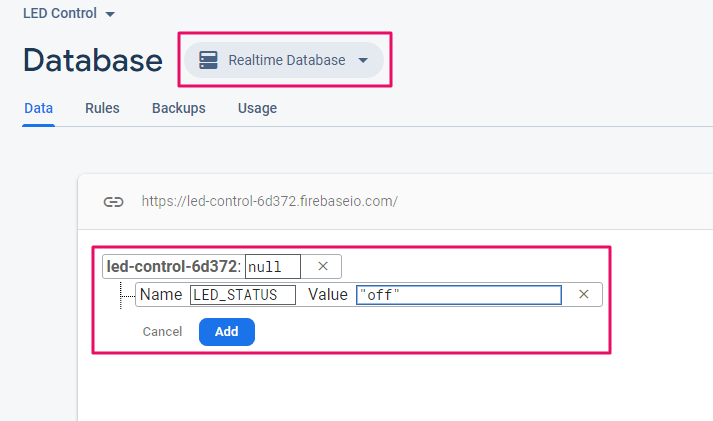
Step 15: Copy “Secret Key” and “Project Name”. This is usually required in the Arduino code. The project name looks like “https://your_project_name.firebaseio.com/“.
Circuit Diagram for IoT based LED Control
Here is the circuit diagram for LED connection with NodeMCU ESP8266 board. Connect the positive terminal of the LED to the GPIO4, i.e the D2 pin of ESP8266 using 220ohm resister as shown in the figure below.
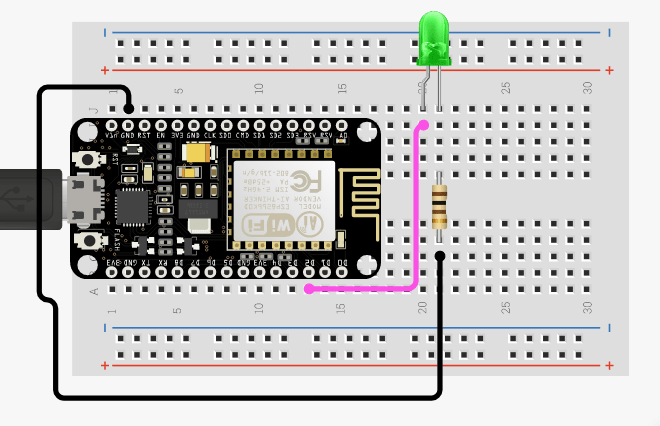
Installing Required Library
To run the code on Arduino IDE, we have to install FirebaseArduino and ArduinoJSON library. FirebaseArdino is a library that makes it easy to connect to the Firebase database from the Arduino client. This is a complete summary of the REST APIs of open firebases via C ++ calls in a wiring friendly way. All JSON Parsing is handled by the library and you can deal in pure C / Arduino types.
The library cannot function alone. Then you should also add the ArudinoJSON library.
1. Installing ArduinoJSON Library
- First of all, go to the library manager on Arduino IDE, and search for “Arduino JSON” & install the library as shown in the figure below
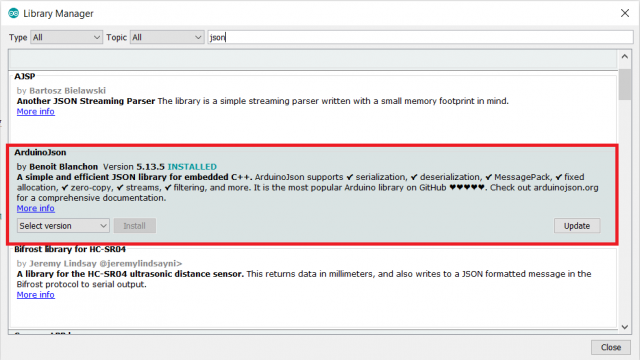
Note: Due to some conflict in the JSON library. The latest JSON library may not work with the following code. So you may need to downgrade the library to version v5.13.5
2. Google FirebaseExtended Library
Now it’s time to install Google Firebase library as well. So, download the library from the below link and add it to the Library folder after extraction. Download Google FirebaseExtended Library
Control LED using Google Firebase & ESP8266
Finally, we are done with both hardware setup & Google Firebase Setup. Now you can upload the code to the NodeMCU ESP8266 Board using Arduino IDE. The complete code is provided below.
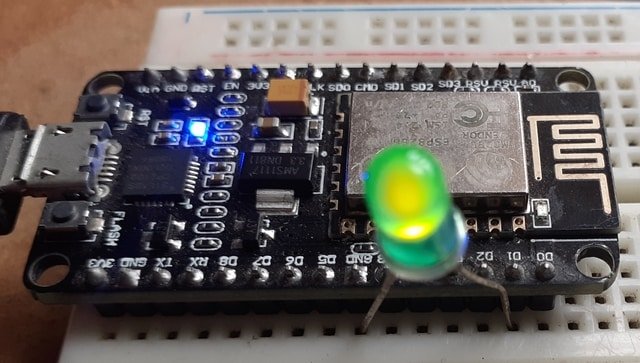
Note: You can type “ON” to ON the LED using Firebase. Also, if you want to change the LED to “OFF” you have to type “OFF”.
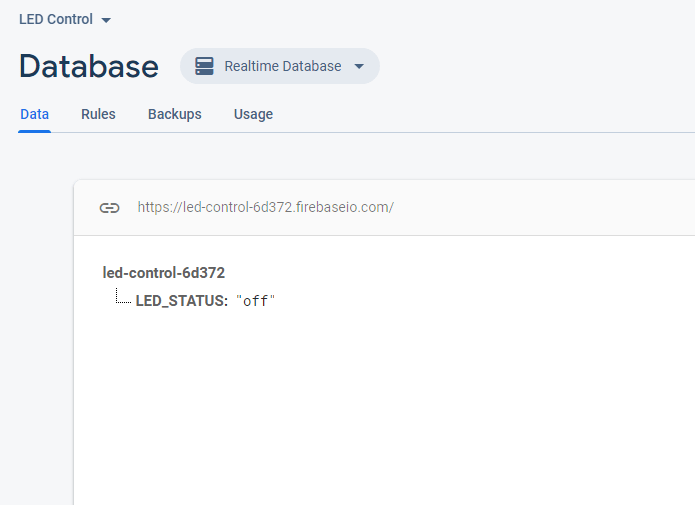
Program Code: IoT Based LED Control using Google Firebase & ESP8266
Here is a Program Code/sketch for Controlling LED using Google Firebase console & ESP8266. Simply, copy the code and upload it to the NodeMCU ESP8266 Board.
Before uploading the code, change the following parameters FIREBASE_HOST, FIREBASE_AUTH, WIFI_SSID & WIFI_PASSWORD with your credentials.
#include <ESP8266WiFi.h>
#include <FirebaseArduino.h>
#define FIREBASE_HOST "ledcontrolproject-34e8e.firebaseio.com" // the project name address from firebase id
#define FIREBASE_AUTH "xyxyzxcvbx********************LdNXL" // the secret key generated from firebase
#define WIFI_SSID "xxxxx"
#define WIFI_PASSWORD "xxxxx"
String fireStatus = ""; // led status received from firebase
int led = 5;
void setup()
{
Serial.begin(9600);
delay(1000);
pinMode(led, OUTPUT);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to ");
Serial.print(WIFI_SSID);
while (WiFi.status() != WL_CONNECTED)
{
Serial.print(".");
delay(500);
}
Serial.println();
Serial.print("Connected to ");
Serial.println(WIFI_SSID);
Firebase.begin(FIREBASE_HOST, FIREBASE_AUTH); // connect to firebase
Firebase.setString("LED_STATUS", "OFF"); //send initial string of led status
}
void loop()
{
fireStatus = Firebase.getString("LED_STATUS"); // get ld status input from firebase
if (fireStatus == "ON")
{ // compare the input of led status received from firebase
Serial.println("Led Turned ON");
digitalWrite(led, HIGH); // make external led ON
}
else if (fireStatus == "OFF")
{ // compare the input of led status received from firebase
Serial.println("Led Turned OFF");
digitalWrite(led, LOW); // make external led OFF
}
else
{
Serial.println("Command Error! Please send ON/OFF");
}
}
Code Explanation
First, we all include the libraries required for this projects.
#include <ESP8266WiFi.h>
#include <FirebaseArduino.h>
Then we define the following two parameters FIREBASE_HOST & FIREBASE_AUTH. We get these parameters from Google Firebase Setup. These two parameters are responsible for communicating with Firebase. It enables the data exchange between the ESP8266 and Google Firebase.
#define FIREBASE_HOST "ledcontrolproject-34e8e.firebaseio.com"
#define FIREBASE_AUTH "KeiqJV41s********************LdNXL"
Then we define the WiFi Network SSID & Password. Replace the credentials with your network SSID and password. The NodeMCU ESP8266 will connect to the WiFi & communicates with Google Firebase.
#define WIFI_SSID "xxxxxxx"
#define WIFI_PASSWORD "xxxxxxx"
The following code will make ESP8266 WiFi Module connect to the network. Once connected it will display the WiFi SSID name and IP address.
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to ");
Serial.print(WIFI_SSID);
while (WiFi.status() != WL_CONNECTED)
{
Serial.print(".");
delay(500);
}
This statement lets the NodeMCU to connect with the Firebase server. Only, If the host address and authorization key are correct then it will connect successfully.
Firebase.begin(FIREBASE_HOST, FIREBASE_AUTH);
It send a string to the Firebase server. The status of LED is changed with this code.
Firebase.setString("LED_STATUS", "OFF");
It helps to get the status of LED from the same Firebase path and save it to a variable.
fireStatus = Firebase.getString("LED_STATUS"); =
if (fireStatus == "ON") {
Serial.println("Led Turned ON");
digitalWrite(led, HIGH);
}
else if (fireStatus == "OFF") {
Serial.println("Led Turned OFF");
digitalWrite(led, LOW);
}
else {
Serial.println("Command Error! Please send ON/OFF");
}
The LED will turn ON/OFF depending upon the String received from firebase as “ON” or “OFF respectively. If any other character is sent, the serial monitor will display “Command Error! Please send ON/OFF”
Wrapping Up
This was all about IoT Controlled LED using Google Firebase Console and ESP8266 NodeMCU Project. I hope this tutorial was helpful to you. Do let me know if you have any doubts and queries regarding this project.