IoT Visitor Counter using ESP32 & Ultrasonic Sensor
IoT Visitor Counter & Automatic Light Control using ESP32
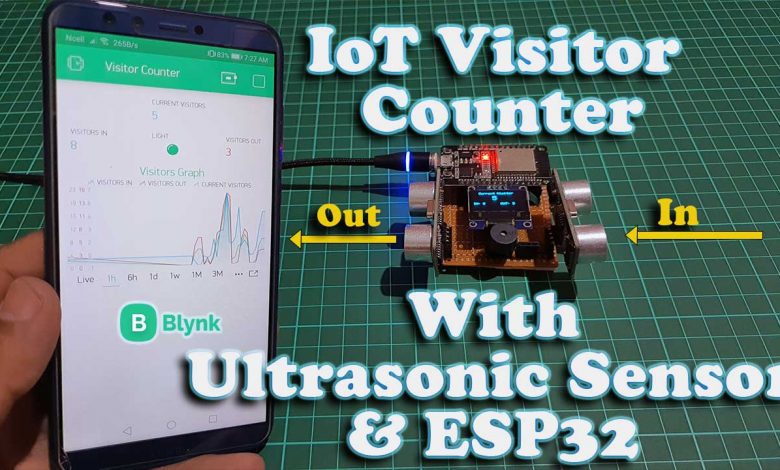
Overview: IoT based Visitor Counter
Today in this project, will make IoT Based Bidirectional Visitor Counter using Ultrasonic sensor, ESP32 & Blynk IoT cloud. This project is useful for monitoring the total number of people entering, exiting, and a current number of persons inside the room from any part of the world and using the Blynk IoT cloud platform. It also controls the AC home appliances like Light bulbs, AC, fan, cooler, etc. Automatically. A pair of Ultrasonic HC-SR04 Sensors are used to count the total number of incoming visitors and outcoming visitors. The Visitors’ data is uploaded automatically to the Blynk IoT cloud using the ESP32 microcontroller.
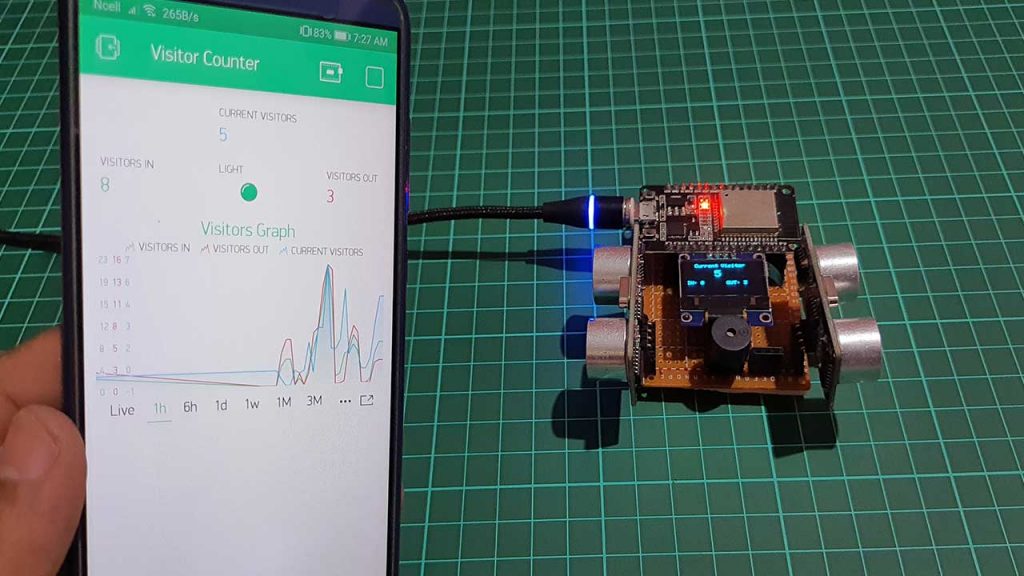
You can use this ESP32 based IoT Bidirectional Visitor counter in the hall, shopping mall, and office entrance gate to count the total number of visitors. This device counts the total number of visitors entering through the entrance gate & also counts the total number of visitors exiting through the exit gate. Then, it calculates the total number of current visitors by subtracting the outgoing visitor from the incoming visitor. When a single person enters the room, the light bulb connected to a relay turns on automatically. Whenever there are no persons present in the room, the light bulb turns off automatically.
Previously, we have made the Visitor Counter Project using Arduino IR sensor & OLED Display. But this time we will use ultrasonic sensor and ESP32 and then send data to the Blynk IoT cloud. We have an OLED Display to display the data locally.
Components Required
We can make this IoT Bidirectional Visitor Counter using ESP32 Development Module, a pair of ultrasonic Sensors, SSD1306 OLED Display, a Buzzer, and a Relay Module. The list of components that you need for creating an IoT Bidirectional Visitor Counter is as follows.
S.N | Components Name | Quantity | ![]() |
---|---|---|---|
1 | ESP32 | 1 | https://amzn.to/3F50M8f |
2 | HC-SR04 Sensor | 2 | https://amzn.to/3F5ipEJ |
3 | OLED Display | 1 | https://amzn.to/32WRubSv |
4 | Relay Module | 1 | https://amzn.to/3gJNJyJ |
5 | Jumper Wires | 20 | https://amzn.to/3vw9ZAt |
6 | Buzzer | 1 | https://amzn.to/3vuvX9M |
7 | Breadboard | 1 | https://amzn.to/2NP2UKL |
IoT Bidirectional Visitor Counter Circuit or Schematic
The Circuit Diagram for IoT Bidirectional Visitor Counter using ESP32 & Ultrasonic sensor is very simple. I designed the circuit diagram for this project using Fritzing Software.
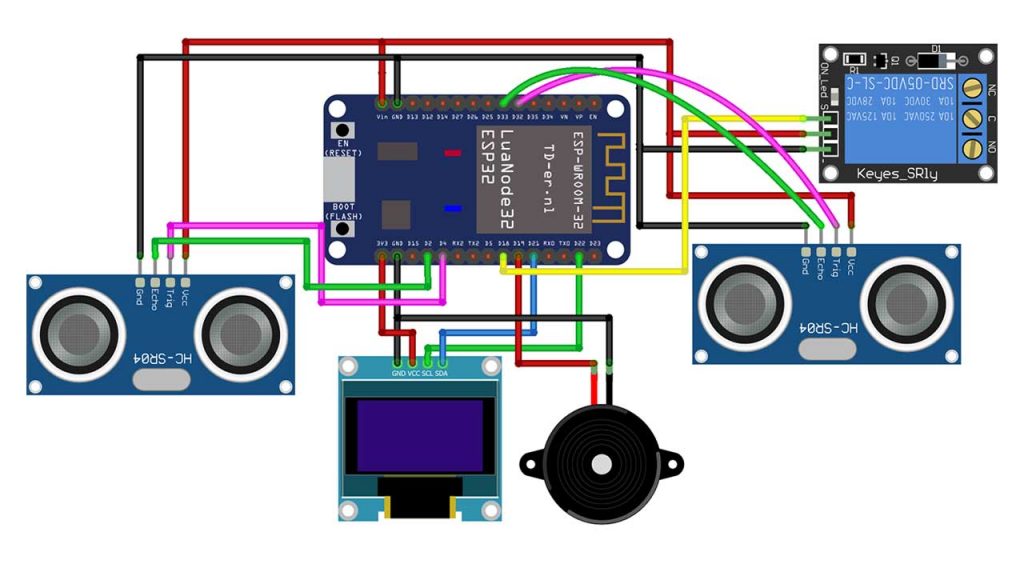
Connect the I2C Pins (SDA & SCL) of 0.96″ SSD1306 OLED Display with ESP32 D21 & D22 Pins. Interface the Trig and Echo pins of the first ultrasonic sensor to D32 and D33 pins. Similarly, connect the Trig and Echo pin of the second ultrasonic sensor D4 and D2 pins respectively. We will use one of the HC-SR04 Sensors at the Entrance gate for counting incoming visitors and the other at the exit gate for counting the outgoing visitor. Similarly, connect a 5V Relay Module to the D18 Pin of ESP32. Also, connect Buzzer positive pin to the D19 pin of ESP32. Provide 5V power to Ultrasonic sensor through Vin pin and connect all grounds to GND pins.
PCB Designing & Ordering
You can simply assemble the circuit on a breadboard. But if you don’t want to assemble the circuit on a breadboard, you can follow this schematic and build your own PCB. You can download the Gerber file of my PCB Design from the link attached below. The PCB looks like the image shown below.
I provided the Gerber File for IoT Visitor Counter using ESP32 & Ultrasonic Sensor PCB below.
You can simply download the Gerber File and order your custom PCB from PCBWay

Visit the PCBWay official website by clicking here: https://www.PCBWay.com/. Simply upload your Gerber File to the Website and place an order. The reason behind most people trusting PCBWay for PCB & PCBA Services is because of their quality. The PCB quality is superb and has a very high finish, as expected.
Setting Up Blynk Application
We need to set up the Blynk App to receive the Visitor Counter data from the ESP32 NodeMCU board. To set up Blynk Application, Download & Install the Application on your smartphone. Available in both Android PlayStore and IOS AppStore. Open the App & create a New Account using your email address.
- Click on create a new project
- Provide the Name of your project as “Visitor Counter”
- Choose ESP32 Dev Board
- Select connection type as Wi-Fi, then click on Create Button.
- They sent the Blynk authentication token to your email address. (We need it later on programming)
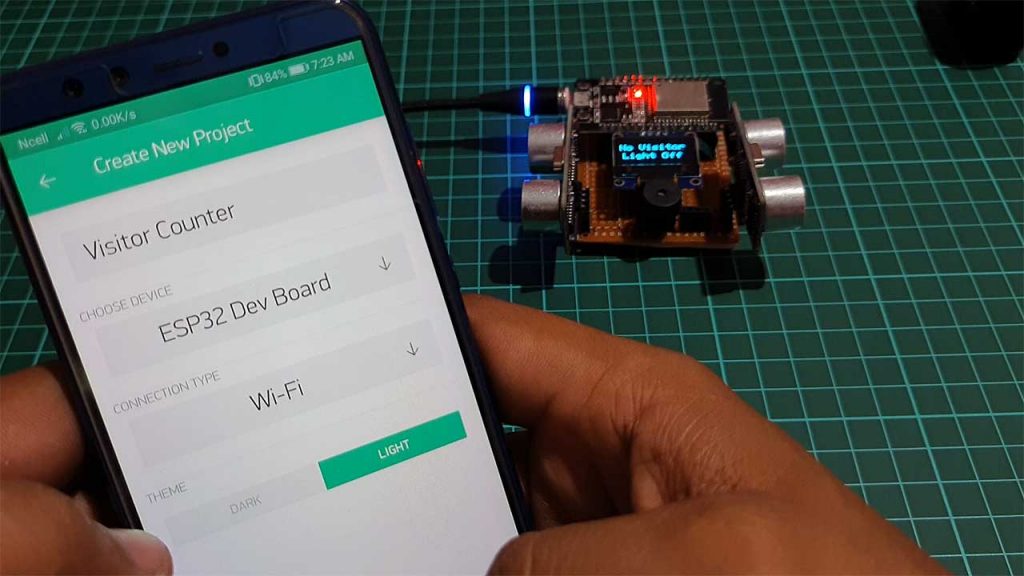
- Now, click on the (+) icon at the top right corner of the screen.
- Search for the “Value Display” widget and add 3 of them to your main screen.
- Also, add Super Chart Widget to the main screen.
- Click on the First Value Display.
- Name it as “Visitors Now”
- Set the Input Pin to Virtual Pin V3, Enter the input Range & Choose the refresh rate as 1sec.
- You can set colors and fonts size according to your need.
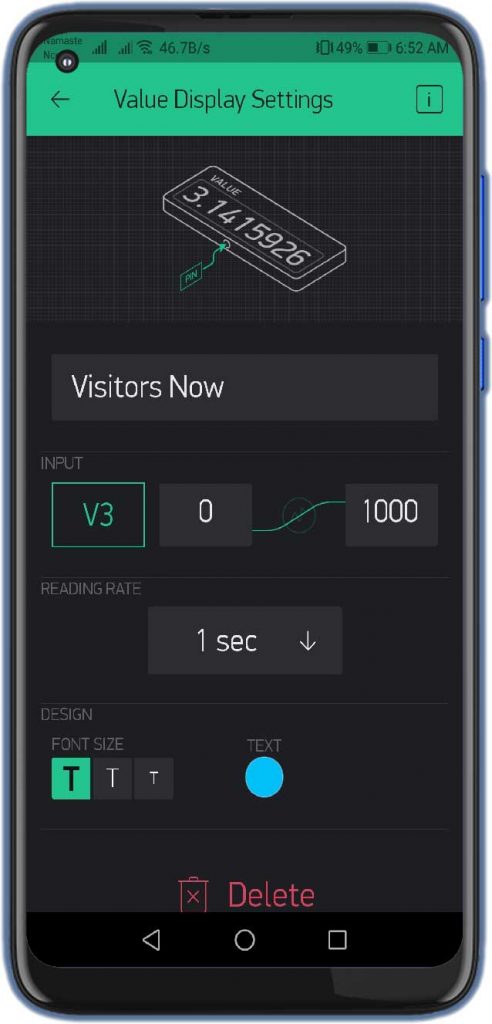
Similarly, do the same for Visitors In, Out, and LED Widget with the help of the images below.
Now, click on the Super Chart widget and Name it as “Visitors Graphs” Add three datastream as Visitors In, Out, and Now with their respective virtual pins.
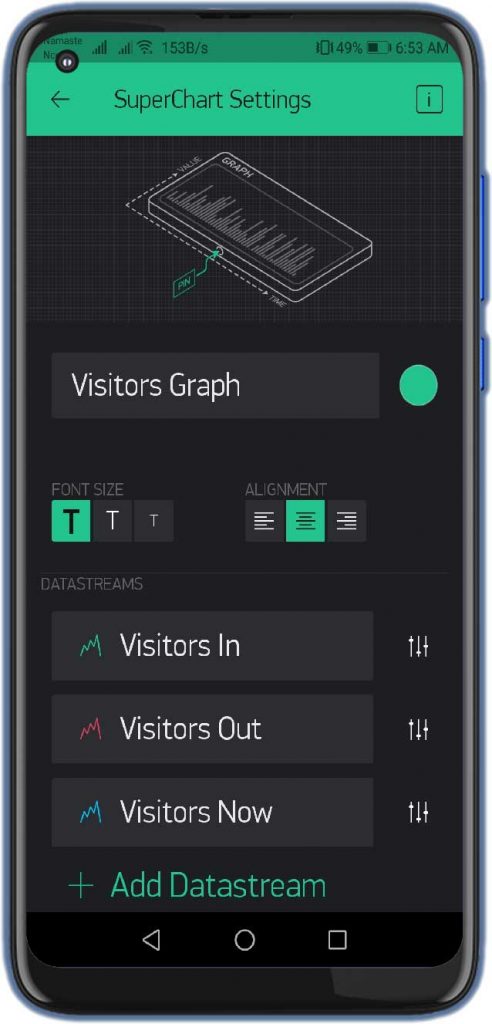
Finally, the Blynk App setup for IoT Visitor Counter using ESP32 & Ultrasonic Sensor is completed.
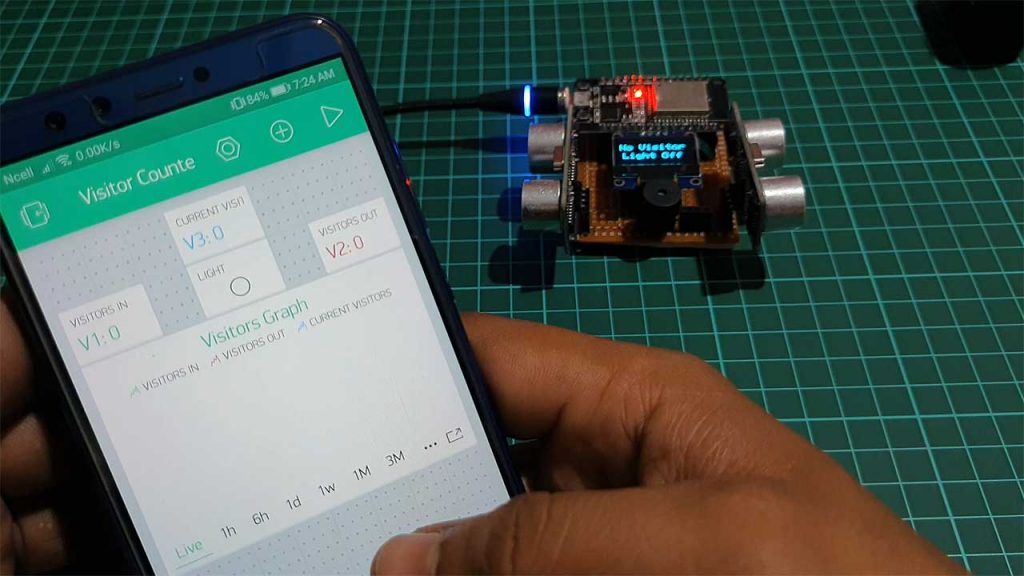
Source Code/Program
The Source Code for ESP8266 based IoT Bidirectional Visitor Counter and Automatic Light Control System is given below. The code requires SSD1306 & Adafruit GFX library & Blynk Library for compilation. First, download the required libraries and add them to the Arduino IDE.
From this code part, change the Wi-Fi SSID, Password & Blynk Authentication Token. Use the same API Token sent to your email address. You can now copy the code and upload it to the ESP32 DOIT V1 Board.
// You should get Auth Token in the Blynk App. // Go to the Project Settings (nut icon). char auth[] = "2rvR6dPiEeBp_TEWxYIydJc4jqgnAqXf"; // Your WiFi credentials. // Set password to "" for open networks. char ssid[] = "Alsan Air WiFi"; char pass[] = "1234567890";
Final Program Code for IoT Visitor Counter & Automatic Light Control using ESP32.
#include <WiFi.h> #include <WiFiClient.h> #include <BlynkSimpleEsp32.h> #include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #include <Ultrasonic.h> // You should get Auth Token in the Blynk App. // Go to the Project Settings (nut icon). char auth[] = "2rvR6dPiEeBp_TEWxYIydJc4jqgnAqXf"; // Your WiFi credentials. // Set password to "" for open networks. char ssid[] = "Alsan Air WiFi"; char pass[] = "1234567890"; #define SCREEN_WIDTH 128 // OLED display width, in pixels #define SCREEN_HEIGHT 64 // OLED display height, in pixels #define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin) Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET); Ultrasonic ultrasonic1(32, 33); Ultrasonic ultrasonic2(4, 2); #define relay 18 //Relay Pin D18 #define BUZZER 19 //buzzer pin D19 int inStatus; int outStatus; int countin = 0; int countout = 0; int in; int out; int now; WidgetLED light(V0); void setup() { Serial.begin(115200); Blynk.begin(auth, ssid, pass); delay(1000); // wait a second pinMode(relay, OUTPUT); digitalWrite(relay, HIGH); pinMode(BUZZER, OUTPUT); digitalWrite(BUZZER, HIGH), delay(100), digitalWrite(BUZZER, LOW); display.begin(SSD1306_SWITCHCAPVCC, 0x3C); //initialize with the I2C addr 0x3C (128x64) delay(2000); Serial.println("Visitor Counter Demo"); display.clearDisplay(); display.setTextSize(2); display.setTextColor(WHITE); display.setCursor(20, 20); display.print("Visitor"); display.setCursor(20, 40); display.print("Counter"); display.display(); delay(3000); } void loop() { Blynk.run(); inStatus = ultrasonic1.read(); outStatus = ultrasonic2.read(); if (inStatus < 20) { in = countin++; digitalWrite(BUZZER, HIGH), delay(200), digitalWrite(BUZZER, LOW); } if (outStatus < 20) { out = countout++; digitalWrite(BUZZER, HIGH), delay(200), digitalWrite(BUZZER, LOW); } now = in - out; if (now <= 0) { light.off(); digitalWrite(relay, HIGH); display.clearDisplay(); display.setTextSize(2); display.setTextColor(WHITE); display.setCursor(0, 15); display.print("No Visitor"); display.setCursor(5, 40); display.print("Light Off"); display.display(); Serial.println("No Visitors! Light Off"); delay(500); } else { light.on(); digitalWrite(relay, LOW); display.clearDisplay(); display.setTextColor(WHITE); display.setTextSize(1); display.setCursor(15, 0); display.print("Current Visitor"); display.setTextSize(2); display.setCursor(50, 15); display.print(now); display.setTextSize(1); display.setCursor(0, 40); display.print("IN: "); display.print(in); display.setTextSize(1); display.setCursor(70, 40); display.print("OUT: "); display.print(out); display.display(); Serial.print("Current Visitor: "); Serial.println(now); Serial.print("IN: "); Serial.println(in); Serial.print("OUT: "); Serial.println(out); delay(500); } Blynk.virtualWrite(V1, in); // Visitors In Blynk.virtualWrite(V2, out); // Visitors Out Blynk.virtualWrite(V3, now); // Current Visitors delay(1000); }
Testing IoT Based Visitor Counter ESP32 Project
The above code for the ESP32-based IoT visitor counter with Blynk fulfills all the requirements of the project. You can upload the code to the ESP32 Wi-Fi Module. Once you upload the code, the ESP32 board will connect to the Blynk Cloud using your Wi-Fi Network. Once it connects to the network, you can open the Serial Monitor. You can simply check all the details on Serial Monitor.
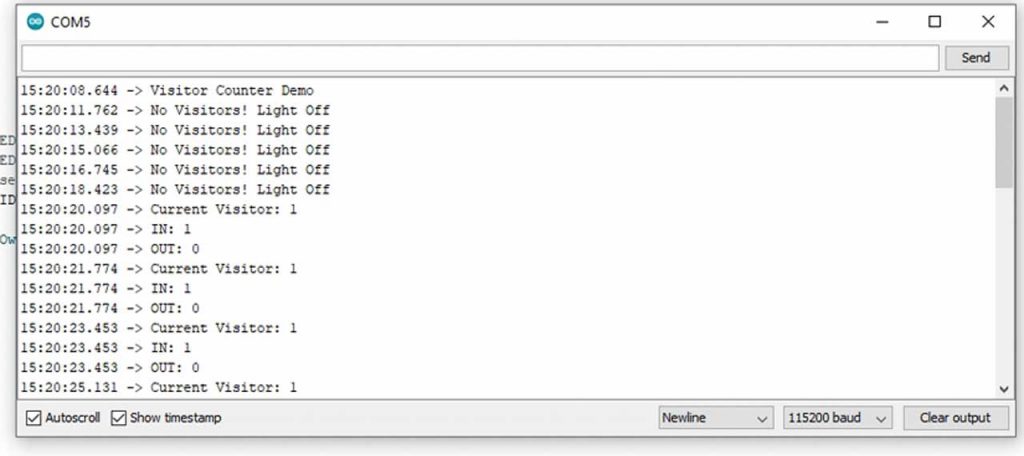
The Serial Monitor will display the connection status of the Wi-Fi Network along with the IP Address. If there is a successful Wi-Fi connection and a further connection to Blynk IoT Cloud. It will update automatically the data after every 1-second interval. The Serial Monitor will also display the number of visitors entering, exiting, current visitors & relay Status.
On the Hardware Part, when there are no visitors, the OLED Display will simply show “No Visitors Lights OFF”.
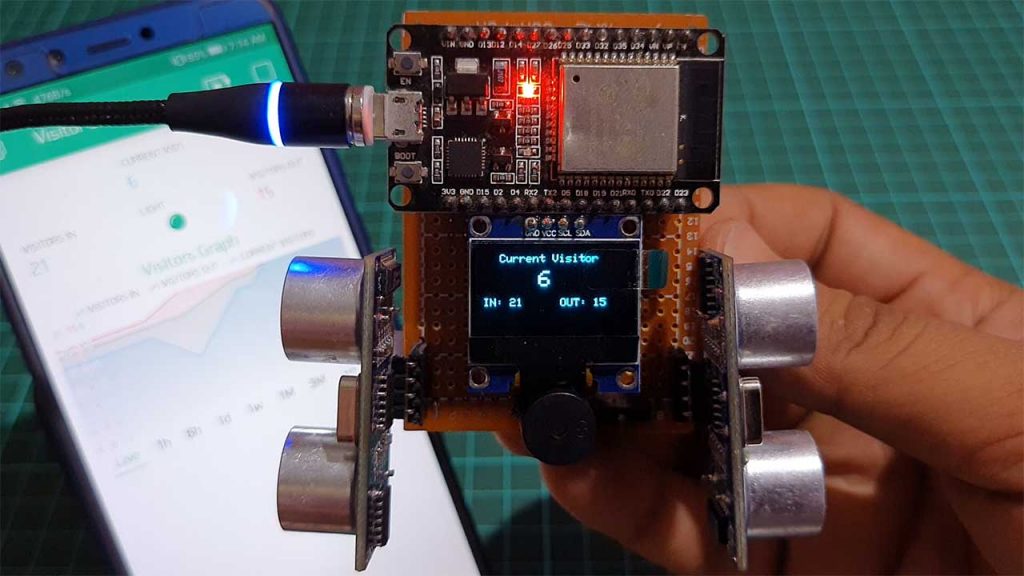
Similarly, when someone enters the room, the light will turn on automatically and OLED Display shows the number of incoming, outgoing, and current visitors.
Monitoring Visitor’s Status Online on Blynk App
As soon as we connected the device to the Wi-Fi and Blynk server, you will see the data appearing on the Blynk App. Here you will see the data for visitors. So the data only changes when an event occurs. You will get a beautiful real-time dashboard as shown in the image below.
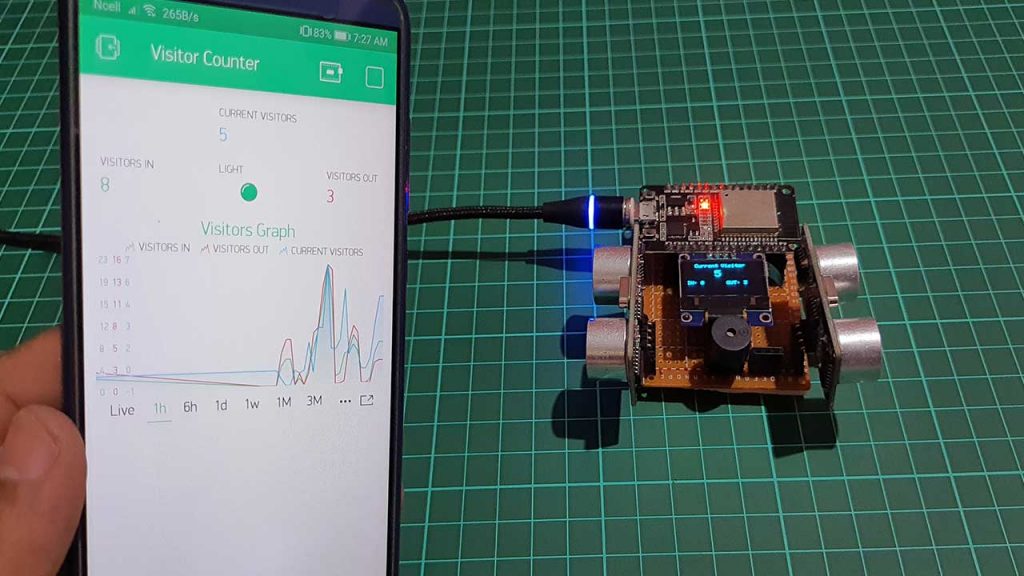
Conclusion
So, that’s pretty much for this tutorial. I hope you enjoyed making your IoT Based Bidirectional Visitor Counter using ESP32, Ultrasonic sensor & Blynk IoT. If you did, don’t forget to share this article with your friends. Want help? Let me know in the comment section below.